Java Reference
In-Depth Information
Java exception processing
Processing exception conditions in Java code consists of three basic opera-
tions:
1. Raising user-defined exceptions by means of a throw clause. This usually
requires extending the Java Exception class in order to provide the excep-
tion response.
2. Handling exceptions, either system generated (implicit) or user-defined
(explicit). Exception handling is based on coding the corresponding try,
catch, and finally clauses.
3. Propagating exceptions into the application's method hierarchy. This is ac-
complished by means of a throws clause.
Note that the throw keyword is used to raise an exception and the
throws keyword to propagate it. It is unfortunate that the designers of the
Java language were unable to find more adequate mnemonics for two
functions that produce so different results. In this topic, we try to reduce
the confusion by referring to the action of the throw keyword as raising
an exception and that of the throws keyword as throwing an exception.
Raising exceptions
Code can use its own exception classes in order to accommodate specific
error conditions. This can be accomplished by defining subclasses of Ex-
ception, or more commonly, by extending Java's Exception class. The Java
Exception class contains two constructors, defined as follows:
Exception()
Exception(String s)
The easiest way to create an exception handler is to extend the Java
Exception class and to call its parameterized constructor. For example,
the following method provides an exception handler for a division by zero
error; the following class extends the Java Exception class and provides a
simple handler for a division by zero error:
class DivByZeroException extends Exception
{
// Parameterized constructor
public DivByZeroException(String message)
{
super(message);
}
}
The method that intends to use the handler in the DivByZeroException
must declare that it throws a DivByZeroException. This done, a throw
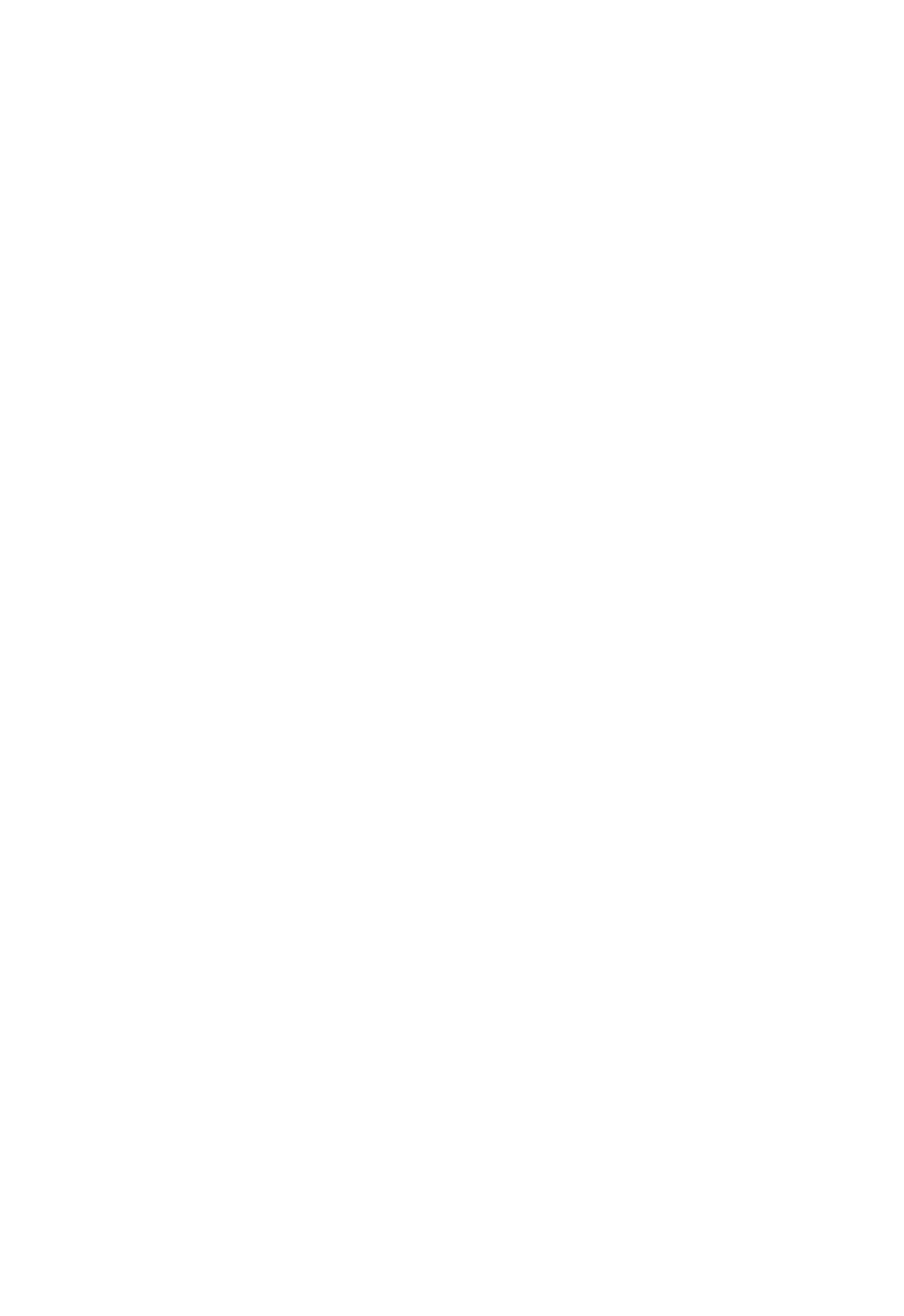






