Java Reference
In-Depth Information
//******************************************
//******************************************
public class Acquaintance
{
public static void main(String[] args)
{
// Create objects of the class Window
Window win1 = new Window(10, 20);
Window win2 = new Window(20, 40);
// Create objects passed as arguments
Rectangle aRect = new Rectangle();
Circle aCirc = new Circle();
win1.WinArea(aRect);
win2.WinArea(aCirc);
}
}
Combining Inheritance and Composition
An object is an instance of a class and a class is a collection of data and
methods that work as a unit. The new operator is used in Java in order to
create an object from a class. We have also seen that a class can be defined
as an abstract data type. Since it is possible to create an array of any primi-
tive data type, it is also possible to create an array of an ADT.
Arrays of objects
When we create an array using a class, the result is an array of objects of the
class. For example, if there is a class named Employee, we create an array
of Employee objects the same way that we create an array of int.
int[] intArray = new int[14];
Employee[] empArray = new Employee[10];
After the array of type Employee has been created, we can store 10 ob-
jects of the class Employee in it. An interesting variation on arrays of ob-
jects is that we can store objects of a subclass in an array of a superclass.
In this manner, if the class HourlyEmployee extends Employee, and emp4
is an object of the subclass, then we can code:
empArray[9] = emp4;
Note that the reverse is not true: an array of a subclass cannot store el-
ements of its superclass, except by typecasting.
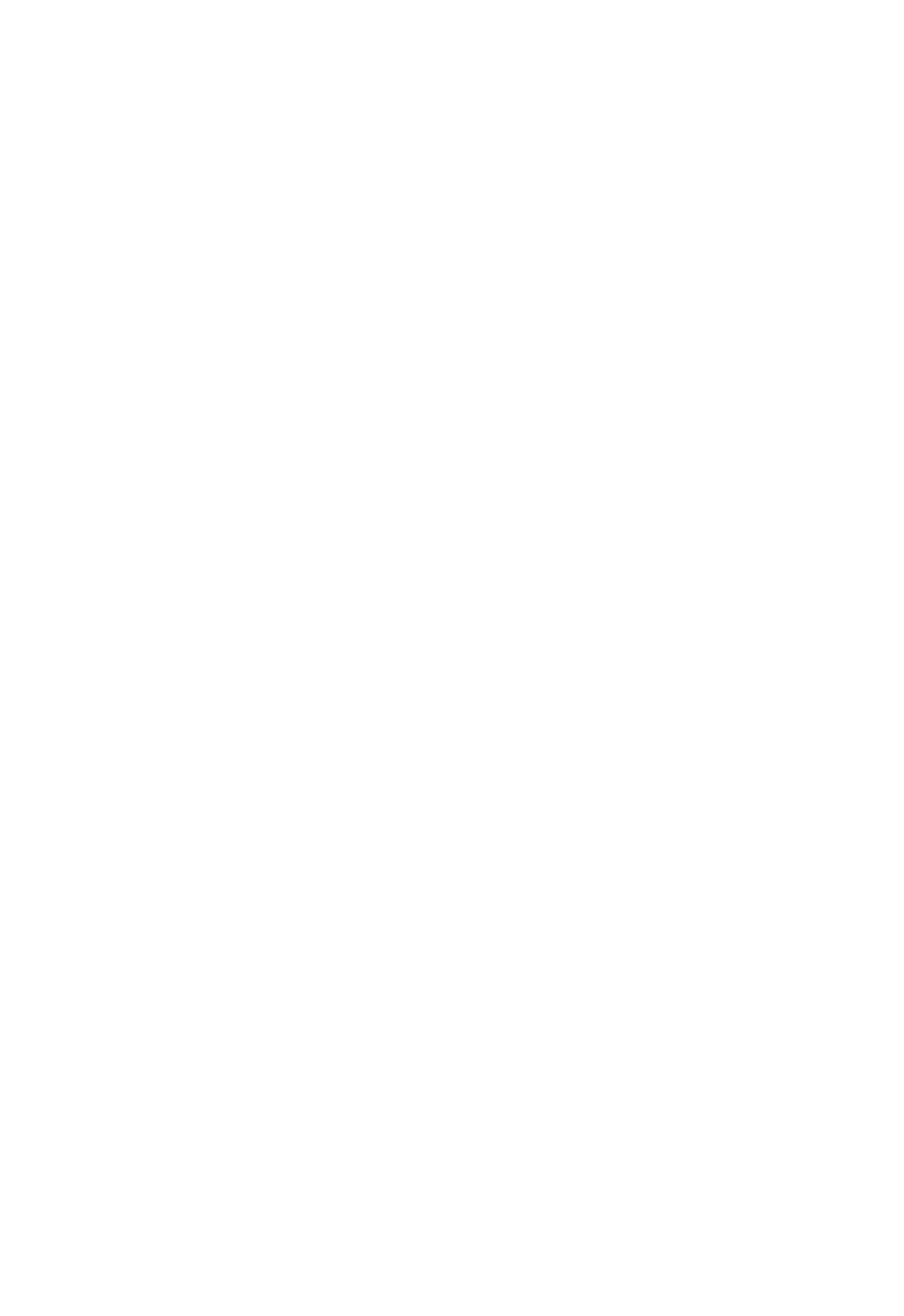






