Java Reference
In-Depth Information
public class MenuDemo
{
public static void main(String[] args)
{
// Local variable
int swValue;
// Display menu graphics
System.out.println("============================");
System.out.println("| MENU SELECTION DEMO |");
System.out.println("============================");
System.out.println("| Options: |");
System.out.println("| 1. Option 1 |");
System.out.println("| 2. Option 2 |");
System.out.println("| 3. Exit |");
System.out.println("============================");
swValue = Keyin.inInt(" Select option: ");
// Switch construct
switch(swValue)
{
case 1:
System.out.println("Option 1 selected");
break;
case 2:
System.out.println("Option 2 selected");
break;
case 3:
System.out.println("Exit selected");
break;
default:
System.out.println("Invalid selection");
break;
// This break is not really necessary
}
}
}
Conditional Expressions
Java expressions usually contain a single operand. There is one ternary op-
erator that uses two operands. Java's ternary operator is also called the
conditional operator
. A conditional expression is used to substitute a sim-
pleif-elseconstruct.Thesyntaxofaconditionalstatementcanbesketched
as follows:
exp1 ? exp2 : exp3
In the above pseudocode exp1, exp2, and exp3 are Java expressions.
First, exp1 is tested. If exp1 is true, then exp2 executes. If exp1 is false,
then exp3 executes. Suppose you want to assign the value of the smaller
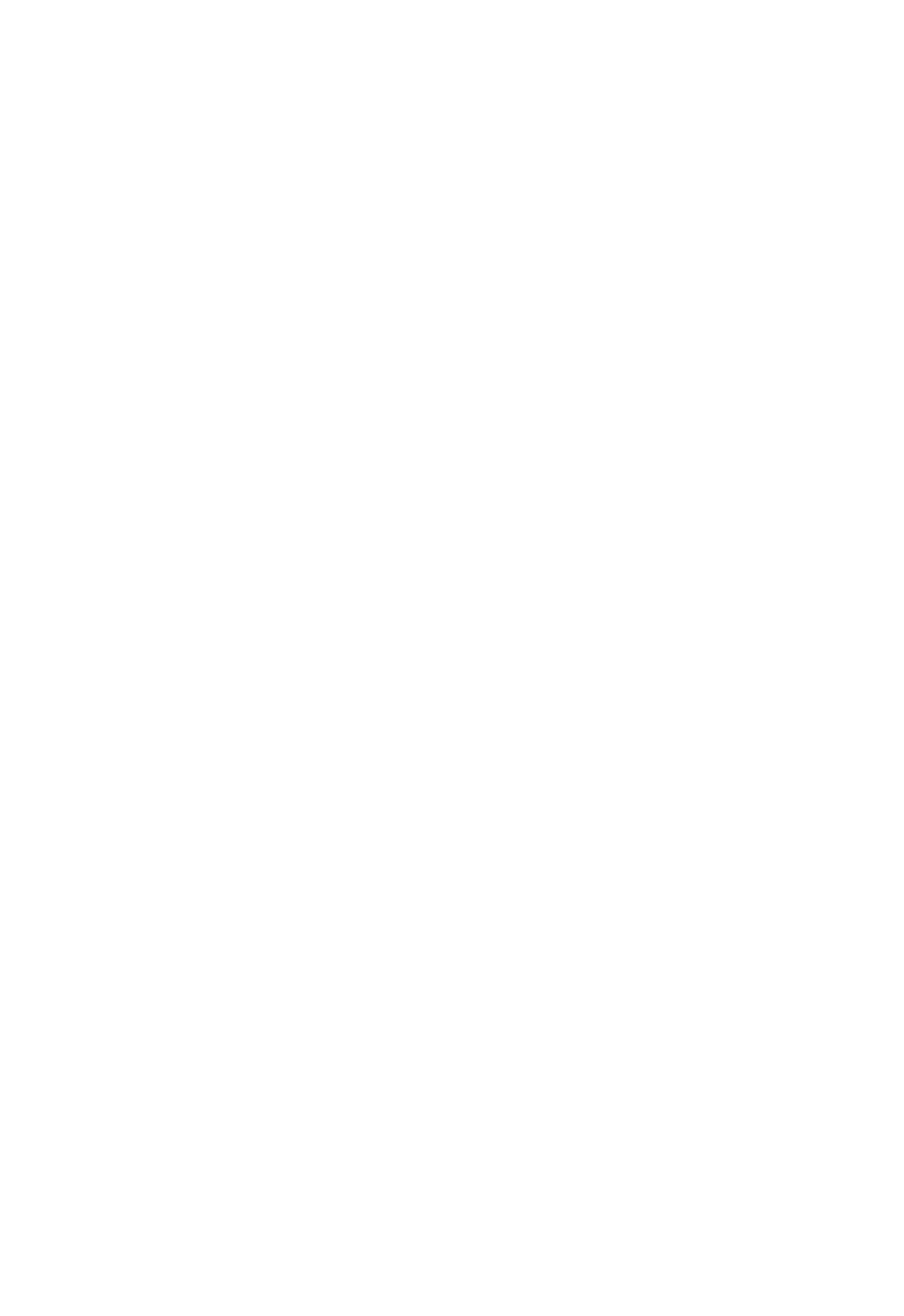






