Java Reference
In-Depth Information
Three different SoundScape objects are then created, each having different AuralAt-
tributes. As defined in line 20, the first Soundscape increases the frequency of the sound
20 times. However, as given by lines 22 and 23, the second Soundscape has no effect on
Figure 5. Code segment for PointSoundScape.java
1.
private void addSound() {
2.
BoundingSphere sBounds1 = new BoundingSphere(new Point3d(), 40.0);
3.
BoundingSphere sBounds2 = new BoundingSphere(new Point3d(-20.0f, 0.0f, 60.0f), 40.0);
4.
PointSound sound1 = new PointSound();
5.
PointSound sound2 = new PointSound(); sound2.setPosition(-20.0f, 0.0f, 60.0f);
6.
MediaContainer data1 = new MediaContainer("file:sound files/howdeep.wav");
7.
MediaContainer data2 = new MediaContainer("file:sound files/ifihadu.wav");
8.
sound1.setSoundData(data1); sound1.setInitialGain(1.0f);
9.
sound2.setSoundData(data2); sound2.setInitialGain(1.0f);
10.
Point2f distanceGain[] = { new Point2f(0,2), new Point2f(10,1.5), new Point2f( 20,1),
11.
new Point2f(30,0.5), new Point2f(50,0) };
12.
sound1.setDistanceGain(distanceGain);
sound2.setDistanceGain(distanceGain);
13.
sound1.setLoop(Sound.INFINITE_LOOPS); sound2.setLoop(Sound.INFINITE_LOOPS);
14.
sound1.setEnable(true); sound2.setEnable(true);
15.
sound1.setSchedulingBounds(sBounds1); sound2.setSchedulingBounds(sBounds2);
16.
BoundingSphere sScapeBounds1 = new BoundingSphere(new Point3d(), 1.0);
17.
BoundingSphere sScapeBounds2 = new BoundingSphere(new Point3d(), 1000.0);
18.
BoundingSphere sScapeBounds3 = new BoundingSphere(new Point3d(-20.0f, 0.0f, 60.0f), 1.0);
19.
AuralAttributes aAttributes1 = new AuralAttributes();
20.
aAttributes1.setFrequencyScaleFactor(20.0f); // increases frequency of the sound rendered
21.
Soundscape sScape1 = new Soundscape(sScapeBounds1, aAttributes1);
22.
AuralAttributes aAttributes2 = new AuralAttributes();
23.
Soundscape sScape2 = new Soundscape(sScapeBounds2, aAttributes2); // default AuralAttribute
24.
AuralAttributes aAttributes3 = new AuralAttributes();
25.
aAttributes3.setReverbDelay(600); // model a large cavern
26.
aAttributes3.setReflectionCoefficient(1); aAttributes3.setReverbOrder(20);
27.
Soundscape sScape3 = new Soundscape(sScapeBounds3, aAttributes3);
28.
this.addChild(sScape2); this.addChild(sScape1); this.addChild(sScape3);
29.
this.addChild(sound1); this.addChild(sound2); }
30.
31. public static void addAvatar(TransformGroup objTransform) {
32. objTransform.addChild(new ColorCube(0.25f)); PointSound sound = new PointSound();
33. MediaContainer data = new MediaContainer("file:sound files/footsteps.wav");
34. sound.setSoundData(data); sound.setInitialGain(0.0f);
35. sound.setCapability(Sound.ALLOW_INITIAL_GAIN_WRITE);
36. sound.setLoop(Sound.INFINITE_LOOPS); sound.setEnable(true);
37. sound.setSchedulingBounds(new BoundingSphere()); objTransform.addChild(sound);
38. KeySoundBehavior keySoundBehavior = new KeySoundBehavior(sound);
39.
keySoundBehavior.setSchedulingBounds(new BoundingSphere(new Point3d(),1000.0));
40.
objTransform.addChild(keySoundBehavior);
41.
PointLight lightPW = new PointLight(new Color3f(1,1,1), new Point3f(0,0,0), new Point3f(1,1,0));
42.
lightPW.setInfluencingBounds(new BoundingSphere(new Point3d(), 5.0));
43.
objTransform.addChild(lightPW); }
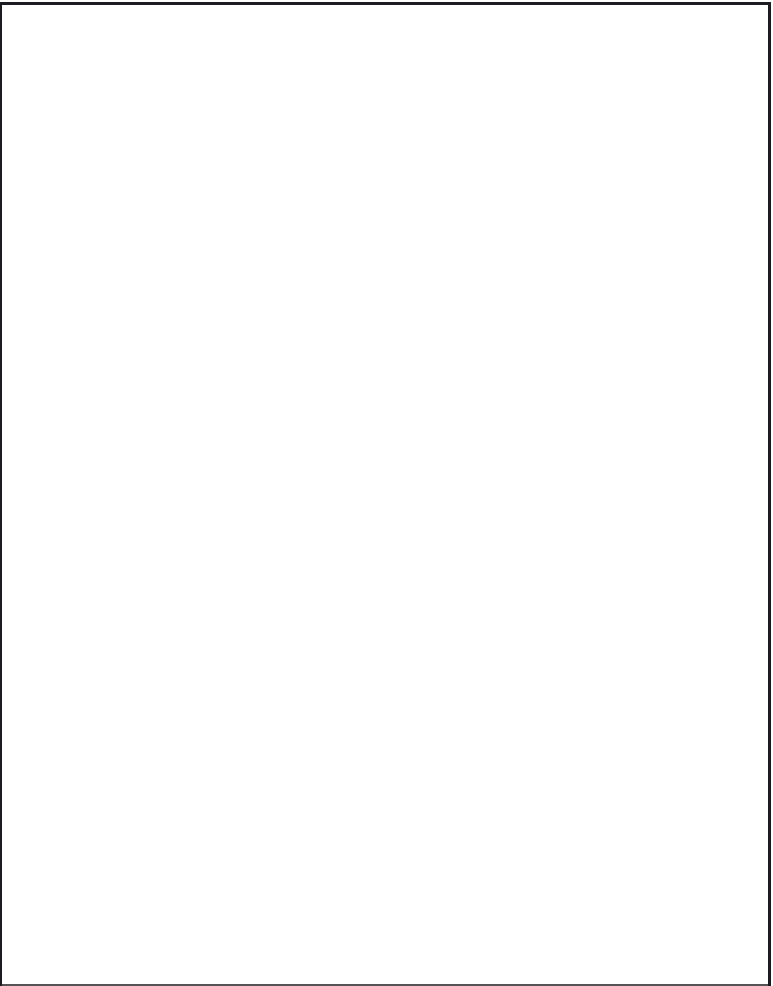
Search WWH ::

Custom Search