Java Reference
In-Depth Information
Figure 6. Third code segment for KeyBoardNavigation.java
56. public class KeyBoardNavigation extends Applet
57. {
58.
private static final int
UP = KeyEvent.VK_UP, DOWN = KeyEvent.VK_DOWN,
59.
LEFT = KeyEvent.VK_LEFT, RIGHT = KeyEvent.VK_RIGHT;
60.
private static final float
PAI= 3.14159f, ANGLEPAI = 2.0f*PAI/360.0f,
61.
SPAN = 0.02f, ANGLESTEP = 0.01f;
62.
private float x, y, z, turningAngle = 0.0f; int direction = 1;
63.
private Matrix4d matrix = new Matrix4d(); private Matrix3f comMat = new Matrix3f();
64.
private WakeupCriterion events[] = new WakeupCriterion[1]; private WakeupOr allEvents;
65.
SimpleUniverse universe = null;
66.
67.
public class KeyBoardBehavior extends Behavior
68.
{
69.
private TransformGroup targetTG;
70.
Point3f viewposi = new Point3f(0.0f,0.0f,2.5f);
71.
72.
KeyBoardBehavior(TransformGroup targetTG) { this.targetTG = targetTG; }
73.
74.
public void initialize() {
75.
events[0]=new WakeupOnAWTEvent(KeyEvent.KEY_PRESSED);
76.
this.wakeupOn(new WakeupOnAWTEvent(KeyEvent.KEY_PRESSED));
77.
allEvents=new WakeupOr(events); wakeupOn(allEvents);
78.
comMat.m00 = 1.0f; comMat.m01 = 0.0f; comMat.m02 = 0.0f;
79.
comMat.m10 = 0.0f; comMat.m11 = 1.0f; comMat.m12 = 0.0f;
80.
comMat.m20 = 0.0f; comMat.m21 = 0.0f; comMat.m22 = 1.0f; }
81.
82.
public void keyPressed(KeyEvent e){
83.
int key=e.getKeyCode();
84.
switch(key) {
85.
case RIGHT: turningAngle -= ANGLESTEP;
86.
setRotation3D(targetTG, -ANGLESTEP, comMat, 1); break;
87.
case LEFT: turningAngle += ANGLESTEP;
88.
setRotation3D(targetTG, ANGLESTEP, comMat, 1); break;
89.
case UP: viewposi.x = viewposi.x-3.0f*SPAN*(float)Math.sin(turningAngle);
90.
viewposi.z = viewposi.z-3.0f*SPAN*(float)Math.cos(turningAngle);
91.
setPosition3D(targetTG, viewposi); break;
92.
case DOWN: viewposi.x = viewposi.x+1.0f*SPAN*(float)Math.sin(turningAngle);
93.
viewposi.z = viewposi.z+1.0f*SPAN*(float)Math.cos(turningAngle);
94.
setPosition3D(targetTG, viewposi); break;
95.
default: }
96.
}
may take in an argument of MouseBehavior.INVERT_INPUTS. The latter specifies that
the mouse response will be in a direction opposite to that normally used for manipulation
objects, and will be appropriate for changing the TransformGroup for the ViewPlatform
in 3D navigation.
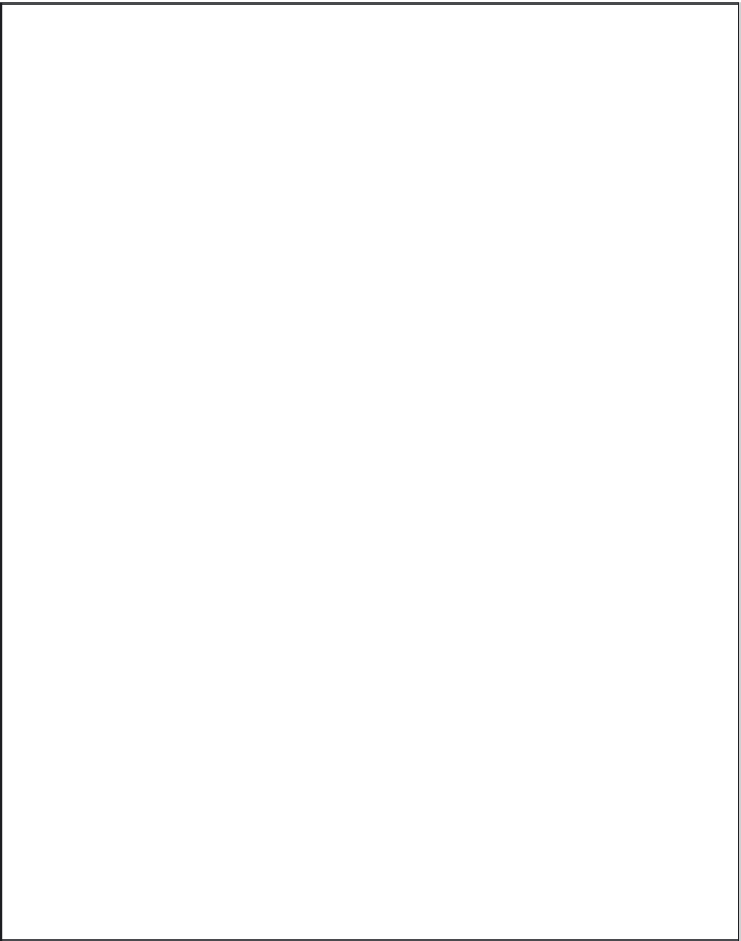
Search WWH ::

Custom Search