Java Reference
In-Depth Information
seen, as picking will not work for these objects. For instance, the back face of a solid ob-
ject, a fully transparent window or a light source cannot be picked. In such applications,
another object that can be picked will have to be created to control the unpickable target
object indirectly.
Since the program will need to change the attributes of objects in a live scene graph, the
appropriate capabilities must be set. For this application, ENABLE_PICK_REPORTING
must be set for the relevant transform group so that it is possible for the scene graph to be
Figure 15. First code segment for PickConeRayBehavior.java
1.
public BranchGroup createSceneGraph()
2.
{
3.
BranchGroup objRoot = new BranchGroup();
4.
TransformGroup objTrans1 = new TransformGroup();
5.
TransformGroup objTrans2 = new TransformGroup();
6.
objRoot.addChild (objTrans1 );
7.
objRoot.addChild (objTrans2 );
8.
9.
// setting the appropriate capabilities
10.
objTrans1.setCapability ( Group.ALLOW_CHILDREN_READ );
11.
objTrans1.setCapability ( TransformGroup.ENABLE_PICK_REPORTING );
12.
objTrans2.setCapability ( Group.ALLOW_CHILDREN_READ );
13.
objTrans2.setCapability ( TransformGroup.ENABLE_PICK_REPORTING );
14.
15.
Background backgd = new Background( 0.0f, 0.0f, 1.0f );
16.
BoundingSphere bounds = new BoundingSphere();
17.
backgd.setApplicationBounds( bounds );
18.
objRoot.addChild( backgd );
19.
20.
// creating a small sphere to be picked in the scene.
21.
Sphere small_sphere1 = new Sphere( 0.005f, createAppearance1() );
22.
objTrans1.addChild( small_sphere1 );
23.
PointLight pointlight1 = new PointLight();
24.
Color3f light_colour = new Color3f( 1.0f, 0.0f, 0.0f );
25.
pointlight1.setColor( light_colour );
26.
pointlight1.setInfluencingBounds( bounds );
27.
pointlight1.setCapability ( Light.ALLOW_STATE_WRITE );
28.
pointlight1.setCapability ( Light.ALLOW_STATE_READ );
29.
pointlight1.setCapability ( Light.ALLOW_COLOR_READ );
30.
pointlight1.setCapability ( Light.ALLOW_COLOR_WRITE );
31.
objTrans1.addChild( pointlight1 );
32.
Transform3D translate = new Transform3D();
33.
translate.set( new Vector3f( 0.5f, 0.5f, 0.5f ) );
34.
objTrans1.setTransform( translate );
35.
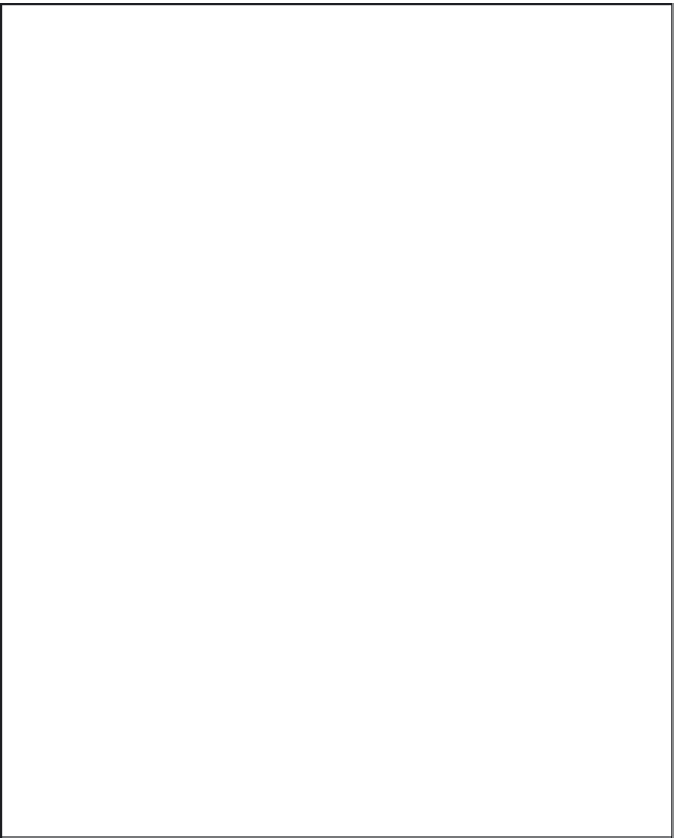
Search WWH ::

Custom Search