Java Reference
In-Depth Information
The code fragment in Figure 1 gives an example where a custom behavior class is cre-
ated for moving a cube upwards when a mouse button is clicked. The example includes the
three basic steps outlined in the previous code development procedure.
Firstly, a constructor is created with a reference to a TransformGroup object of change.
This is done from lines 16 to 20. Secondly, from lines 22 to 26, the initialization() method
is used to specify the initial trigger wakeup condition which, for this case, is an AWTEvent
Figure 1. Code segment for SimpleMouseBehavior.java
1.
import java.awt.event.*;
2.
import java.util.Enumeration;
3.
4.
public class SimpleMouseBehavior extends Applet
5.
{
6.
private WakeupCriterion events[] = new WakeupCriterion[1];
7.
private WakeupOr allEvents;
8.
9.
public class SimpleMouseMovement extends Behavior
10.
{
11.
private TransformGroup targetTG;
12.
private Transform3D trans = new Transform3D();
13.
private double TransX = 0.0;
14.
private double TransY = 0.0;
15.
16.
//create SimpleBehavior
17.
SimpleMouseMovement (TransformGroup targetTG)
18.
{
19.
this.targetTG = targetTG;
20.
}
21.
22.
//initialize the behavior; set initial wakeup condition; called when behavior becomes live
23.
public void initialize()
24.
{
25.
this.wakeupOn(new WakeupOnAWTEvent(MouseEvent.MOUSE_PRESSED));
26.
}
27.
28.
public void processStimulus(Enumeration criteria)
29.
{
30.
//decode event ; do what is necessary
31.
TransY+=0.05;
32.
trans.set(new Vector3f((float)TransX,(float)TransY,0.0f));
33.
targetTG.setTransform(trans);
34.
this.wakeupOn(new WakeupOnAWTEvent(MouseEvent.MOUSE_PRESSED));
35.
}
36.
}
37. }
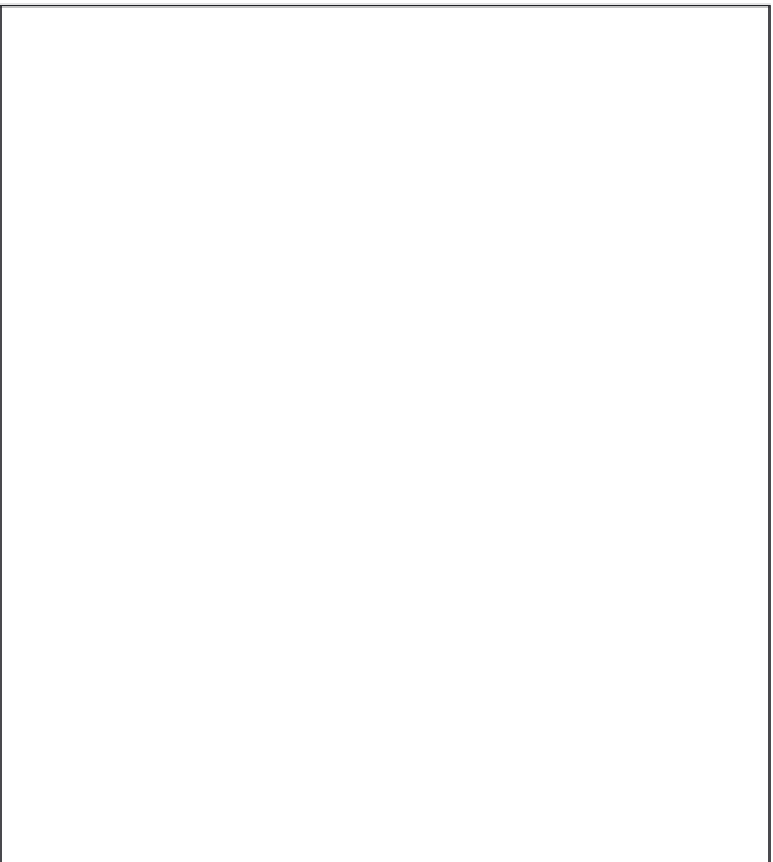
Search WWH ::

Custom Search