Game Development Reference
In-Depth Information
The following example illustrates this. Suppose we wish to check if
a hardware device is capable of doing vertex processing in hardware (or
in other words, whether the device supports transformation and light-
ing calculations in hardware). By looking up the
D3DCAPS9
structure in
the SDK documentation, we find that the bit
D3DDEVCAPS_HWTRANS-
FORMANDLIGHT
in the data member
D3DCAPS9::DevCaps
indicates
whether the device supports transformation and lighting calculations in
hardware. Our test then, assuming
caps
is a
D3DCAPS9
instance and
has already been initialized, is:
bool supportsHardwareVertexProcessing;
// If the bit is “on” then that implies the hardware device
// supports it.
if( caps.DevCaps & D3DDEVCAPS_HWTRANSFORMANDLIGHT )
{
// Yes, the bit is on, so it is supported.
supportsHardwareVertexProcessing = true;
}
else
{
// No, the bit is off, so it is not supported.
hardwareSupportsVertexProcessing = false;
}
Note:
DevCaps
stands for “device capabilities.”
Note:
We learn how we initialize a
D3DCAPS9
instance based on a
particular hardware device's capabilities in the next section.
Note:
We recommend that you look up the
D3DCAPS9
structure in
the SDK documentation and examine the complete list of capabilities
that Direct3D exposes.
1.4
Initializing Direct3D
The following subsections show how to initialize Direct3D. The pro-
cess of initializing Direct3D can be broken down into the following
steps:
1.
Acquire a pointer to an
IDirect3D9
interface. This interface is
used for finding out information about the physical hardware
devices on a system and creating the
IDirect3DDevice9
inter-
face, which is our C++ object that represents the physical
hardware device we use for displaying 3D graphics.
2.
Check the device capabilities (
D3DCAPS9
) to see if the primary dis-
play adapter (primary graphics card) supports hardware vertex












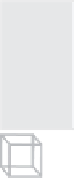
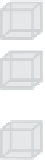




Search WWH ::

Custom Search