Game Development Reference
In-Depth Information
The MessageBox Function
There is one last API function that we have not yet covered, and that is
the
MessageBox
function. This function is a very handy way to provide
the user with information and get some quick input. The declaration for
the
MessageBox
function looks like this:
int MessageBox(
HWND hWnd, // Handle of owner window, may specify null.
LPCTSTR lpText, // Text to put in the message box.
LPCTSTR lpCaption, // Text to put for the title of the message
// box.
UINT uType
// Style of the message box.
);
The return value for the
MessageBox
function depends on the type of
message box. See the MSDN library for a list of possible return values
and styles.
A Better Message Loop
Games are very different applications than traditional Windows applica-
tions, such as office type applications and web browsers. Games are not
typically event driven in the usual sense, and they must be updated
constantly. For this reason, when we actually start writing our 3D pro-
grams we will, for the most part, not deal with Windows messages.
Therefore, we will want to modify the message loop so that if there is a
message, we will process it. But if there is not a message, then we
want to run our game code. Our new message loop is as follows:
int Run()
{
MSG msg;
while(true)
{
if(::PeekMessage(&msg, 0, 0, 0, PM_REMOVE))
{
if(msg.message == WM_QUIT)
break;
::TranslateMessage(&msg);
::DispatchMessage(&msg);
}
else
// run game code
}
return msg.wParam;
}





















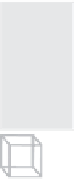

Search WWH ::

Custom Search