Game Development Reference
In-Depth Information
// Compute projection matrix.
D3DXMatrixPerspectiveFovLH(
&Proj, D3DX_PI * 0.25f,
(float)Width / (float)Height, 1.0f, 1000.0f);
return true;
}
The
Display
function is quite simple. It tests for user input and
updates the view matrix accordingly. However, because we perform the
view matrix transformation in the shader, we must also update the view
matrix variable within the shader. We do this using the constant table:
bool Display(float timeDelta)
{
if( Device )
{
//
// Update view matrix code snipped...
//
D3DXMATRIX V;
D3DXMatrixLookAtLH(&V, &position, &target, &up);
DiffuseConstTable->SetMatrix(Device, ViewMatrixHandle, &V);
D3DXMATRIX ViewProj=V*Proj;
DiffuseConstTable->SetMatrix(Device, ViewProjMatrixHandle,
&ViewProj);
//
// Render
//
Device->Clear(0, 0, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER,
0xffffffff, 1.0f, 0);
Device->BeginScene();
Device->SetVertexShader(DiffuseShader);
Teapot->DrawSubset(0);
Device->EndScene();
Device->Present(0, 0, 0, 0);
}
return true;
}
Also observe that we enable the vertex shader that we wish to use
right before the
DrawSubset
call.
Cleaning up is done as expected; we simply release the allocated
interfaces:
void Cleanup()
{
d3d::Release<ID3DXMesh*>(Teapot);











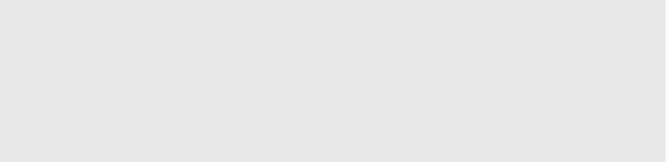


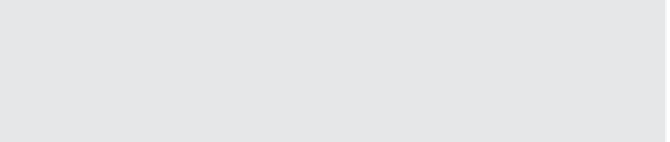






Search WWH ::

Custom Search