Game Development Reference
In-Depth Information
8.2.3.4
Part IV
The next part of the
RenderMirror
function computes the matrix that
positions the reflection in the scene:
// position reflection
D3DXMATRIX W, T, R;
D3DXPLANE plane(0.0f, 0.0f, 1.0f, 0.0f); // xy plane
D3DXMatrixReflect(&R, &plane);
D3DXMatrixTranslation(&T,
TeapotPosition.x,
TeapotPosition.y,
TeapotPosition.z);
W=T*R;
Notice that we first translate to where the non-reflection teapot is posi-
tioned. Then, once positioned there, we reflect across the xy plane.
This order of transformation is specified by the order in which we mul-
tiply the matrices.
8.2.3.5
Part V
We are almost ready to render the reflected teapot. However, if we ren-
der it now, it will not be displayed. Why? Because the reflected teapot's
depth is greater than the mirror's depth, and thus the mirror primitives
technically obscure the reflected teapot. To get around this, we clear
the depth buffer:
Device->Clear(0, 0, D3DCLEAR_ZBUFFER, 0, 1.0f, 0);
Not all problems are solved, however. If we simply clear the depth
buffer, the reflected teapot is drawn in front of the mirror and things do
not look right. What we want to do is clear the depth buffer in addition
to blending the reflected teapot with the mirror. In this way, the
reflected teapot looks like it is “in” the mirror. We can blend the
reflected teapot with the mirror with the following blending equation:
FinalPixel
sourcePixel
destPixel DestPixel
0, 0, 0, 0
sourcePixel
destPixel
Since the source pixel will be from the reflected teapot and the destina-
tion pixel will be from the mirror, we can see from this equation how
they will be blended together. In code we have:
Device->SetRenderState(D3DRS_SRCBLEND, D3DBLEND_DESTCOLOR);
Device->SetRenderState(D3DRS_DESTBLEND, D3DBLEND_ZERO);










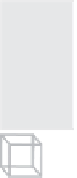
Search WWH ::

Custom Search