Java Reference
In-Depth Information
Chapter 4
Generics
In this chapter, you will learn
•
What generics are
•
How to define generic types, methods, and constructors
•
How to define bounds for type parameters
•
How to use wildcards as the actual type parameters
•
How the compiler infers the actual type parameters for generic type uses
•
Generics and their limitations in array creations
•
How the incorrect use of generics may lead to heap pollution
What Are Generics?
Generics let you write true polymorphic code, which is code that works with any type. Please refer to Chapter 1 in
the topic
Beginning Java Fundamentals
(ISBN 978-1-4302-6652-5) for more details on polymorphism and writing
polymorphic code.
Let's discuss a simple example before I define what generics are and what they do for us. Suppose you want to
create a new class whose sole job is to store a reference to any type, where “any type” means any reference type.
Let's call this class
ObjectWrapper
, as shown in Listing 4-1.
Listing 4-1.
A Wrapper Class to Store a Reference of Any Type
// ObjectWrapper.java
package com.jdojo.generics;
public class ObjectWrapper {
private Object ref;
public ObjectWrapper(Object ref) {
this.ref = ref;
}
public Object get() {
return ref;
}
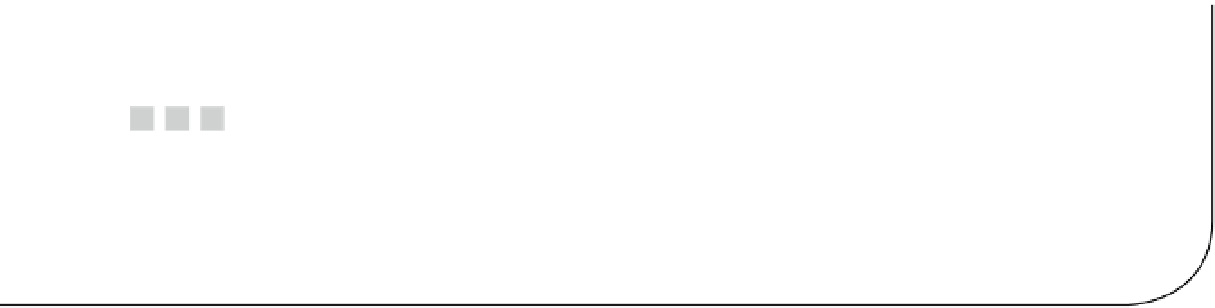