Java Reference
In-Depth Information
Guideline for using an inner class
. If the purpose of a class
In
is simply to support another class
Out
—meaning that
In
is used
only in
Out
and in no other part of the program— and if
In
needs
to reference non-static components of
Out
, then make
In
an inner
class of
Out
.
An inner class that is an iterator
We provide a second example of a useful inner class, having to do with iter-
ators. Consider a class
Bank
that maintains a set of bank accounts, as outlined in
Fig. 12.15. The accounts are stored in array segment
bank[0..size-1]
. Con-
sider writing an
Iterator
that will produce the accounts, but in reverse order.
We show it as a separate class, also in Fig. 12.15.
Activity
12-6.3
import
java.util.*;
/**
An instance is an array of bank accounts
*/
public class
Bank {
//
Class invariant: the accounts are in
bank[0..size - 1]
private
BankAccount[] bank;
private int
size;
/**
A (reverse) iterator for bank accounts
*/
private class
BAIterator
implements
Iterator {
/** bank[0..n-1]
remains to be enumerated
*/
private int
n=
size;
/**
= "
there is another account to enumerate
"
*/
private int
hasNext()
{
return
n > 0; }
/**
=
the next item to be enumerated
*/
public
Object next() {
n= n - 1;
return
bank[n];
}
/** remove
is not implemented
*/
public void
remove() {}
}
/**
An iterator that enumerates bank accounts in reverse order
*/
public
Iterator iterator()
{
return new
BAIterator(); }
}
Figure 12.16:
Class Bank, with BAIterator as an inner class
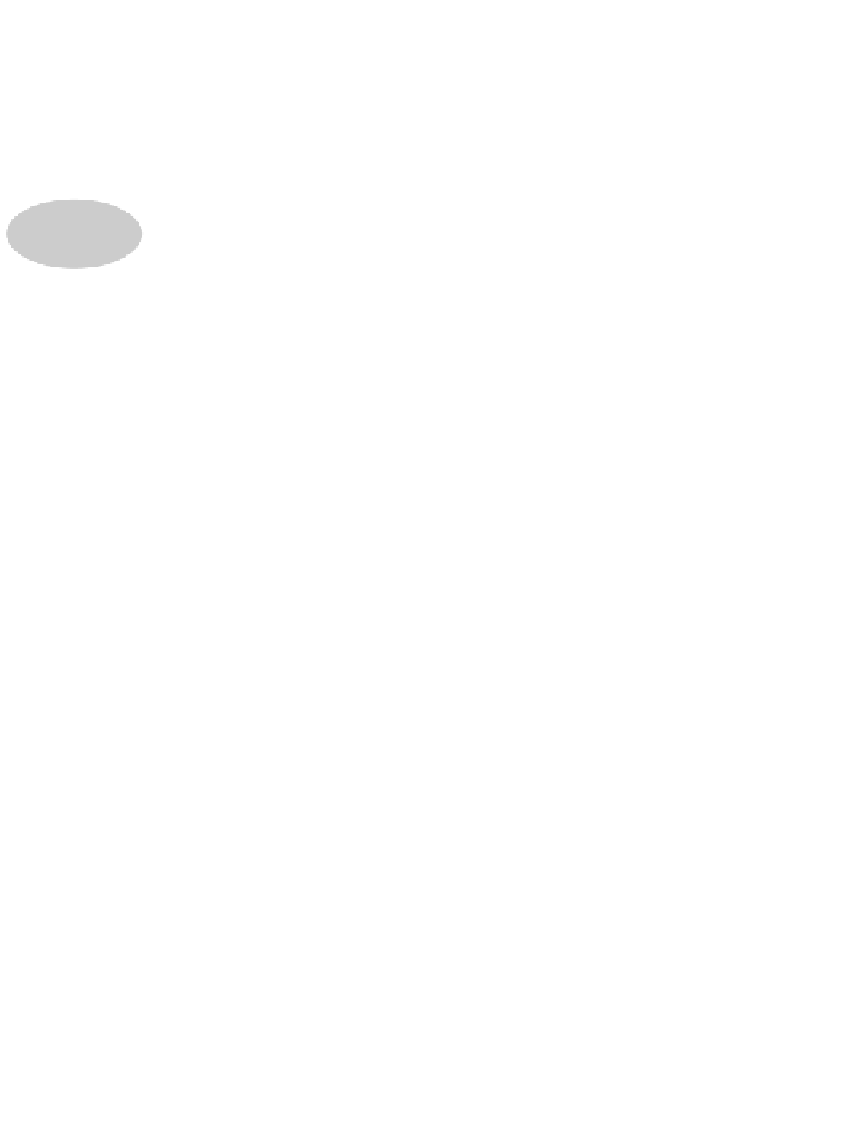
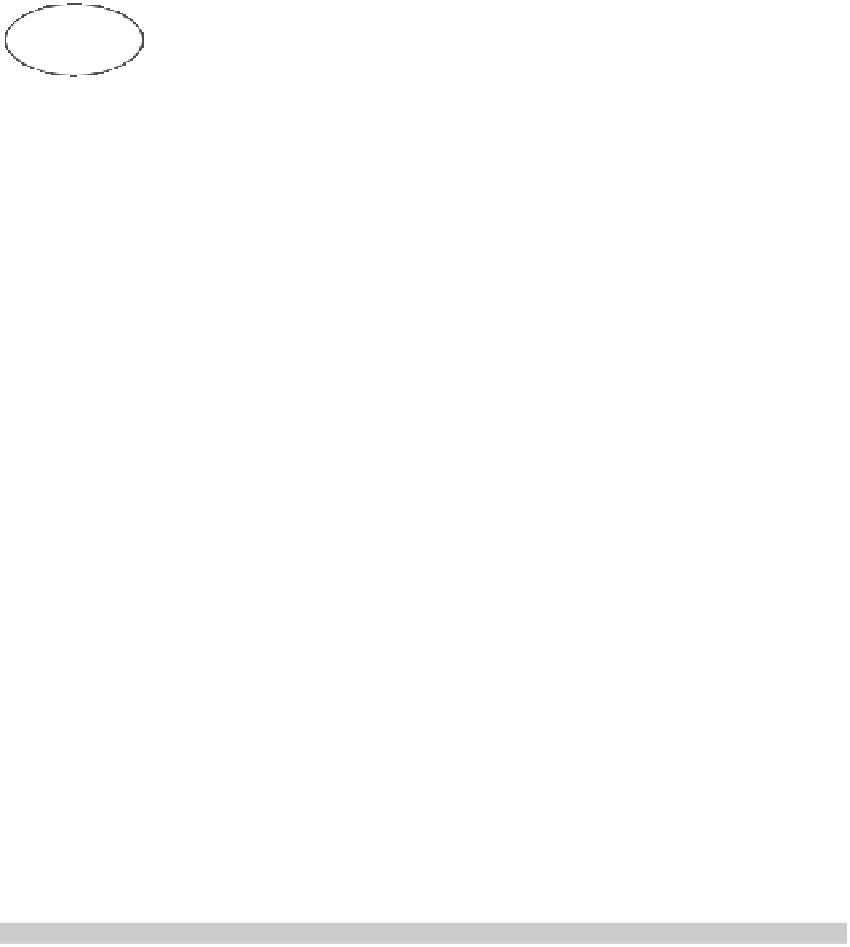

Search WWH ::

Custom Search