Graphics Reference
In-Depth Information
// check for OpenGL errors so far
CheckGlErrors( “Vertex Shader 1” );
// see if we had compilation errors
glGetShaderiv( vertShader, GL_COMPILE_STATUS, &status );
if( status == GL_FALSE )
{
fprintf( stderr, “Vertex shader compilation failed.\n” );
glGetShaderiv( vertShader, GL_INFO_LOG_LENGTH, &logLength
);
GLchar *log = new GLchar [logLength];
glGetShaderInfoLog( vertShader, logLength, NULL, log );
fprintf( stderr, “\n%s\n”, log );
delete [ ] log;
exit( 1 );
}
CheckGlErrors( “Vertex Shader 2” );
In the call to
glCreateShader( ),
the argument,
GL_VERTEX_SHADER
, has
been highlighted. This is to emphasize that this is the only place that identi-
fies what type of shader this is. Additional legal values are
GL_TESSELLATION_
CONTROL_SHADER
,
GL_TESSELLATION_EVALUATION_SHADER
,
GL_GEOMETRY_
SHADER
and
GL_FRAGMENT_SHADER
. This shader type is then stored in the shader
object for later use. Other than this, each shader is compiled and atached the
same way. It is important, of course, to set the shader type correctly so that
the handling of the overall shader program knows what to do with each indi-
vidual shader. Also, the compiler will sometimes produce different errors,
depending on the type of shader; this is because certain things are legal in one
type of shader but not others.
You will notice the construction
(const GLchar **)&str
for the shader
source string. You can, of course, use a simpler construction for this and only
read in a single string, but GLSL lets you construct a shader from a collec-
tion of source fragments that are stored in an array of strings and are only
assembled at compile time. This gives you extra flexibility and lets you build
a shader toolkit that is much finer grained than only having full shader source
files.
As an example of taking this approach, you could use the same shader
source, and insert the appropriate
#define
statements at the beginning by hav-
ing each set of
#define
s in its own text ile, leting you avoid time-consuming
if
tests. You can insert a common header file (a standard
.h
file) in the source
if you like, or you can simulate the
#include
to re-use common pieces of code,
such as frequently used functions.
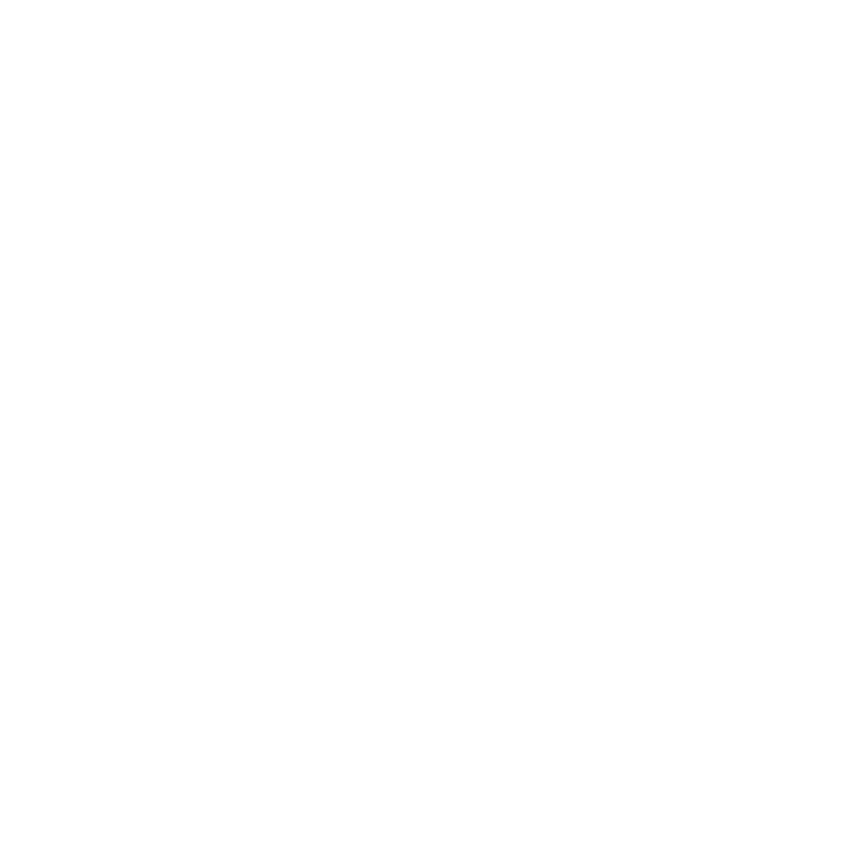

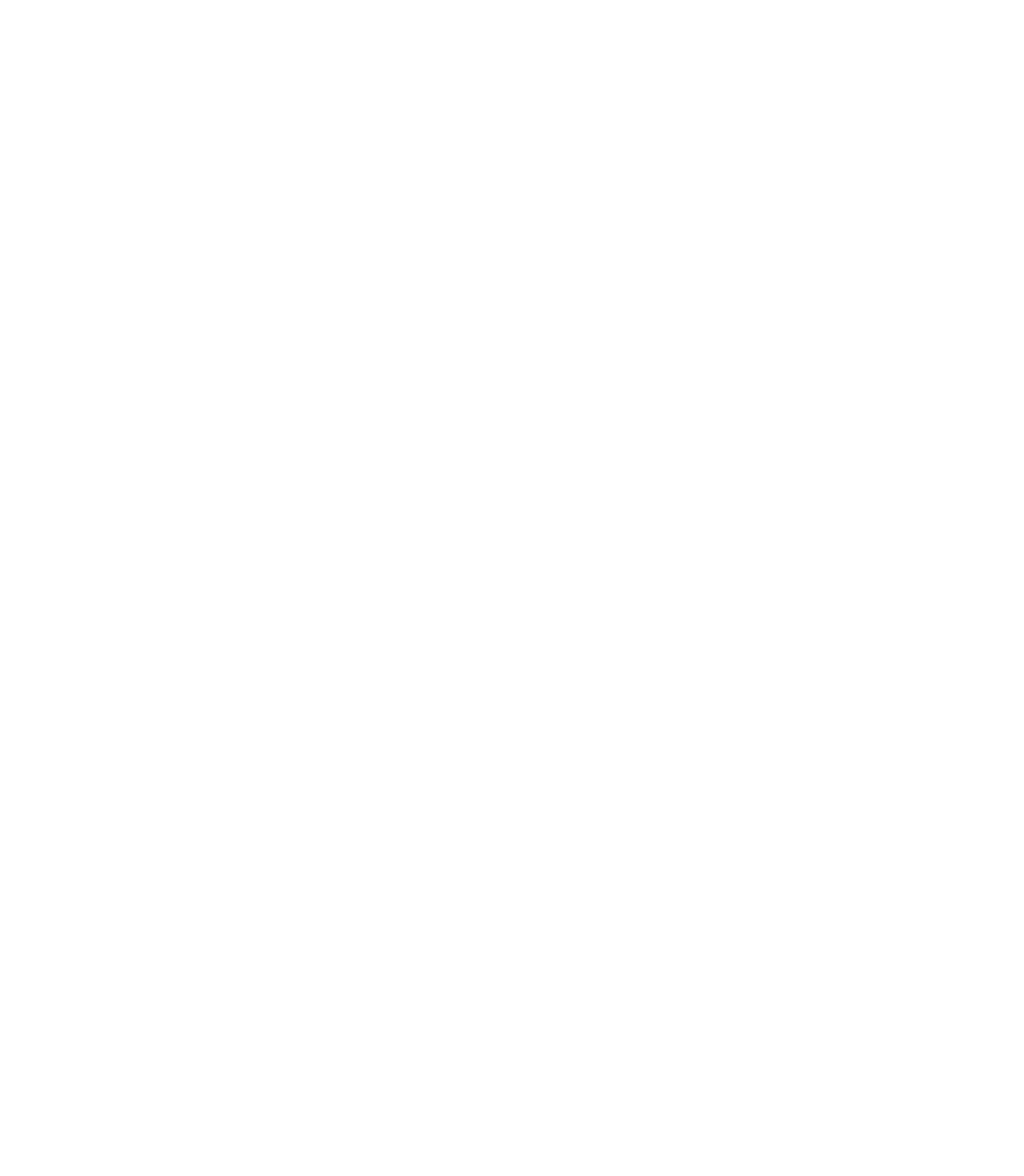