Graphics Reference
In-Depth Information
fFragColor = vLightIntensity*AMBCOEFF*vColor;
// Add diffuse light based on spotlight
float myAngleCosine = dot( LightDirection, EyeDirection );
float CutoffCosine = cos( radians(uAngle) );
float BlendFactor = smoothstep( CutoffCosine - uWidth,
CutoffCosine + uWidth, myAngleCosine);
fFragColor += DIFFCOEFF*BlendFactor*vColor*vLightIntensity;
}
Of course, in an application,
uAngle
and
uWidth
would be passed to the
shader as uniform variables from the application, and it would be beter to
compute the value of
CutoffCosine
there, instead of for each pixel. We do it as
above in order to take advantage of
glman
.
Setting Up Lighting for Shading
Shading is the process of determining the color of each pixel in each primitive
in your scene. This is actually carried out in the fragment processing part of
the graphics processor that we described in Figure 1.5, but the vertex proces-
sor must set up the right environment for the kind of shading that you will
implement. In this section, we will discuss some kinds of shading and how
they are set up. In our discussion, we will draw on several shader concepts
from Chapter 2.
The standard shading models available in fixed-function OpenGL are
limited. They are
flat shading
, where a polygon is given a single color, and
smooth shading
, where the colors at the vertices of the polygon are interpo-
lated to fill its interior. These are far from the only kinds of shading that have
been used in the graphics field, but they are enough for many kinds of graph-
ics work. More sophisticated shading is discussed later in this chapter and in
Chapter 8.
Recall from the discussions in Chapter 1 that the fixed-function vertex
processor must set a color for each vertex, and that the fragment processor can
only interpolate vertex colors. This gives us our first two kinds of shading: flat
shading and smooth shading. However, if we have vertex and fragment shad-
ers, we can set up
out
variables in the vertex shader so that the fragment shader
can interpolate other information and compute each pixel's color directly.
This gives us two other kinds of shading: Phong shading and anisotropic
shading.
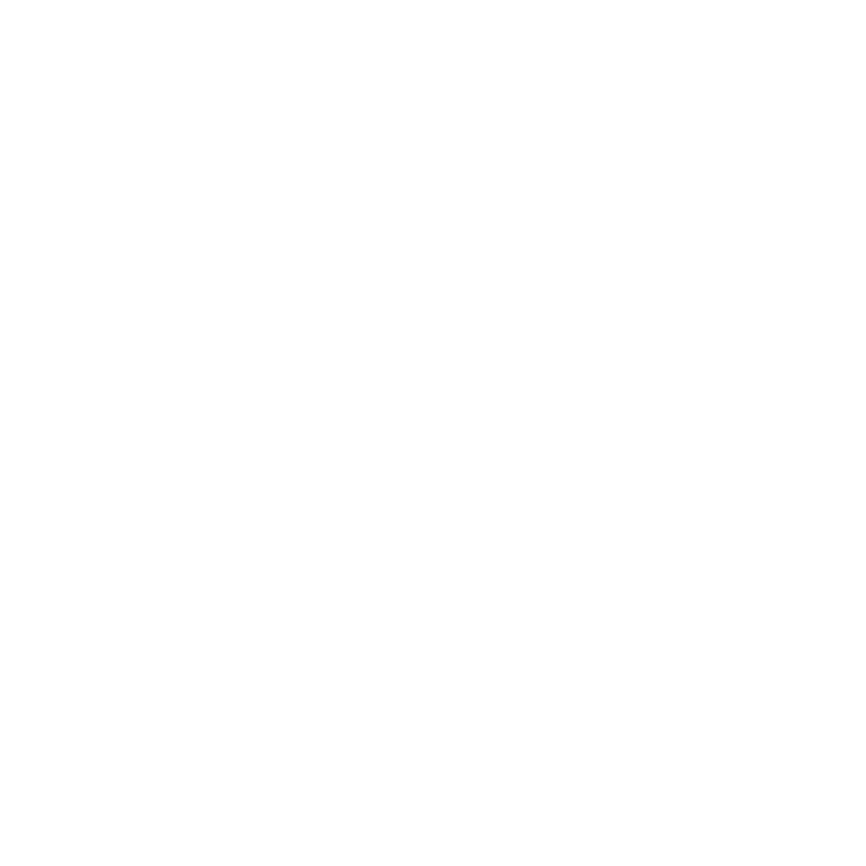
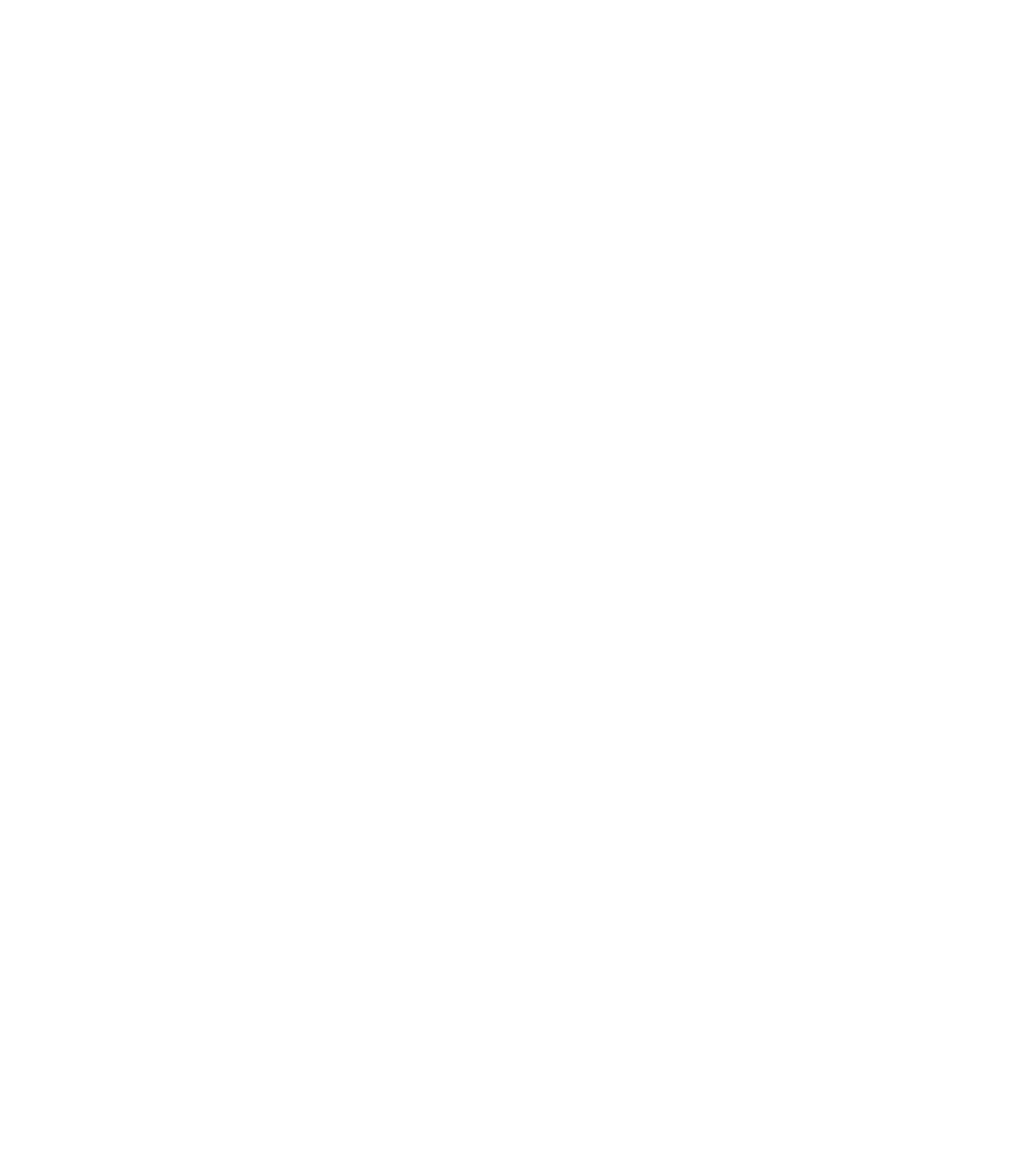