Java Reference
In-Depth Information
5
. “Iron Man” is an expression, not a statement. To make this lambda valid, you can remove the
curly braces and semicolon as follows: (String s) -> "Iron Man". Or if you prefer, you can use an
explicit return statement as follows: (String s) -> {return "Iron Man";}.
Table 3.1
provides a list of example lambdas with examples of use cases.
Table 3.1. Examples of lambdas
Use case
Examples of lambdas
A boolean expression
(List<String> list) -> list.isEmpty()
Creating objects
() -> new Apple(10)
Consuming from an object
(Apple a) -> {
System.out.println(a.getWeight());
}
Select/extract from an object
(String s) -> s.length()
Combine two values
(int a, int b) -> a * b
Compare two objects
(Apple a1, Apple a2) -> a1.getWeight().compareTo(a2.getWeight())
3.2. Where and how to use lambdas
You may now be wondering where you're allowed to use lambda expressions. In the previous
example, you assigned a lambda to a variable of type Comparator<Apple>. You could also use
another lambda with the filter method you implemented in the previous chapter:
List<Apple> greenApples =
filter(inventory,
(Apple a) -> "green".equals(a.getColor())
);
So where exactly can you use lambdas? You can use a lambda expression in the context of a
functional interface. In the code shown here, you can pass a lambda as second argument to the
method filter because it expects a Predicate<T>, which is a functional interface. Don't worry if
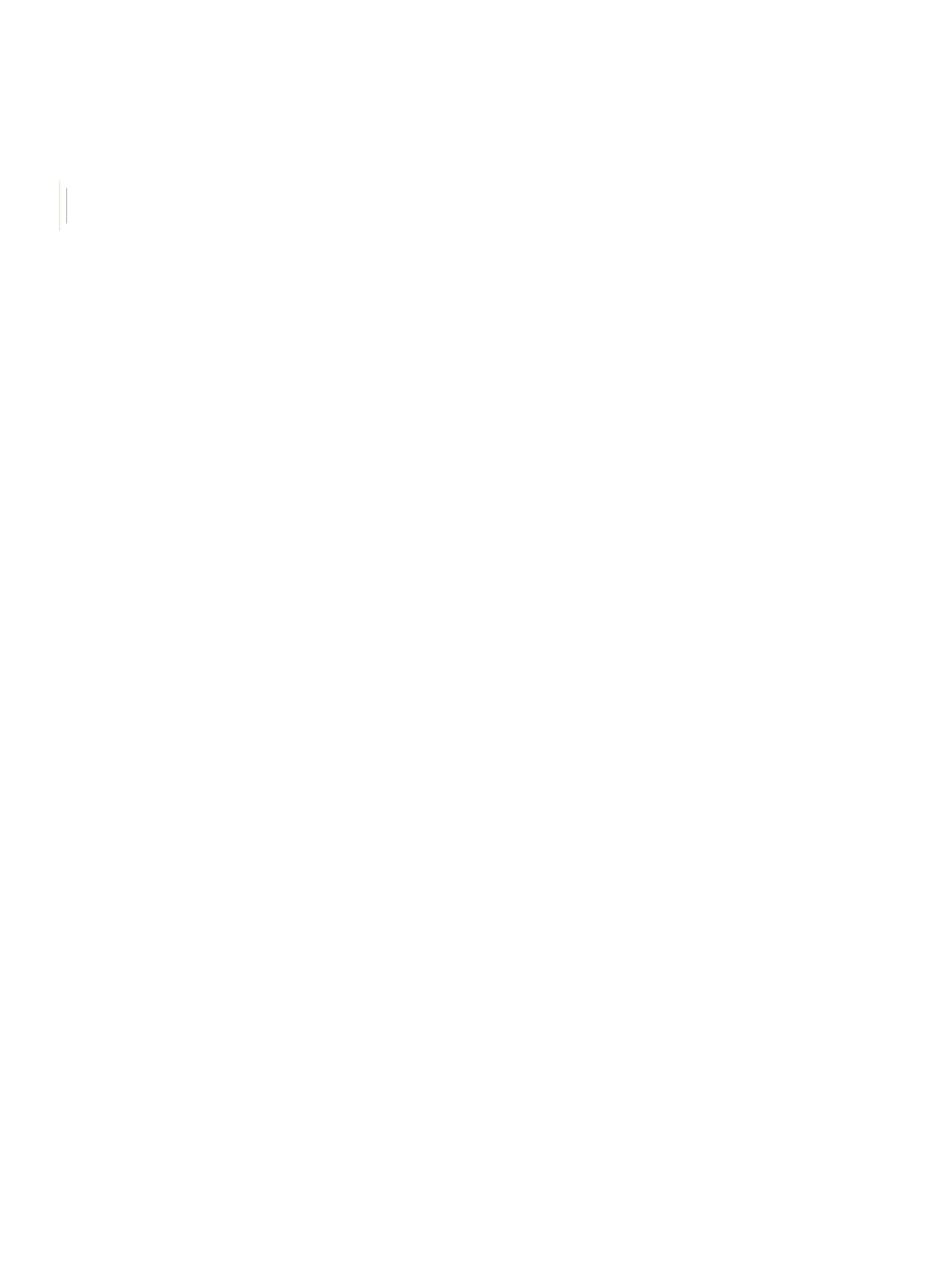



Search WWH ::

Custom Search