Java Reference
In-Depth Information
not using operations available on the Option type, which enforces the idea of “null checking.”
You can no longer forget to do it because it's enforced by the type system.
Okay, we diverged a bit, and all this sounds fairly abstract. You might now wonder “So, what
about Java 8?” Well actually, Java 8 takes inspiration from this idea of an “optional value” by
introducing a new class called java.util.Optional<T>! In this chapter, we show the advantages of
using it to model potentially absent values instead of assigning a null reference to them. We also
clarify how this migration from nulls to Optionals requires you to rethink the way you deal with
optional values in your domain model. Finally, we explore the features of this new Optional class
and provide a few practical examples showing how to use it effectively. Ultimately, you'll learn
how to design better APIs in which—just by reading the signature of a method—users can tell
whether to expect an optional value.
10.2. Introducing the Optional class
Java 8 introduces a new class called java.util.Optional<T> that's inspired by the ideas of Haskell
and Scala. It's a class that encapsulates an optional value. This means, for example, that if you
know a person might or might not have a car, the car variable inside the Person class shouldn't
be declared type Car and assigned to a null reference when the person doesn't own a car, but
instead should be type Optional<Car>, as illustrated in
figure 10.1
.
Figure 10.1. An optional
Car
When a value is present, the Optional class just wraps it. Conversely, the absence of a value is
modeled with an “empty” optional returned by the method Optional.empty. It's a static factory
method that returns a special singleton instance of the Optional class. You might wonder what
the difference is between a null reference and Optional .empty(). Semantically, they could be
seen as the same thing, but in practice the difference is huge: trying to dereference a null will
invariably cause a NullPointer-Exception, whereas Optional.empty() is a valid, workable object
of type Optional that can be invoked in useful ways. You'll soon see how.
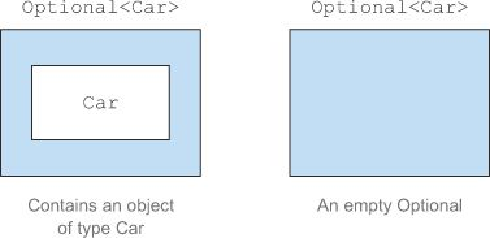
Search WWH ::

Custom Search