Java Reference
In-Depth Information
Static methods and interfaces
A common pattern in Java is to define both an interface and a utility companion class defining
many static methods for working with instances of the interface. For example, Collections is a
companion class to deal with Collection objects. Now that static methods can exist inside
interfaces, such utility classes in your code can go away and their static methods can be moved
inside an interface. These companion classes will remain in the Java API in order to preserve
backward compatibility.
The chapter is structured as follows. We first walk you through a use case of evolving an API and
the problems that can arise. We then explain what default methods are and how they can tackle
the problems faced in the use case. Next, we show how you can create your own default methods
to achieve a form of
multiple inheritance
in Java. We conclude with some more technical
information about how the Java compiler resolves possible ambiguities when a class inherits
several default methods with the same signature.
9.1. Evolving APIs
To understand why it's difficult to evolve an API once it's been published, let's say for the
purpose of this section that you're the designer of a popular Java drawing library. Your library
contains a Resizable interface that defines many methods a simple resizable shape must support:
setHeight, setWidth, getHeight, getWidth, and setAbsoluteSize. In addition, you provide several
out-of-the-box implementations for it such as Square and Rectangle. Because your library is so
popular, you have some users who have created their own interesting implementations such as
Ellipse using your Resizable interface.
A few months after releasing your API, you realize that Resizable is missing some features. For
example, it would be nice if the interface had a setRelativeSize method that takes as argument a
growth factor to resize a shape. You might say that it's easy to fix: just add the setRelativeSize
method to Resizable and update your implementations of Square and Rectangle. Well, not so
fast! What about all your users who created their own implementations of the Resizable
interface? Unfortunately, you don't have access to and can't change their classes that implement
Resizable. This is the same problem that the Java library designers face when they need to
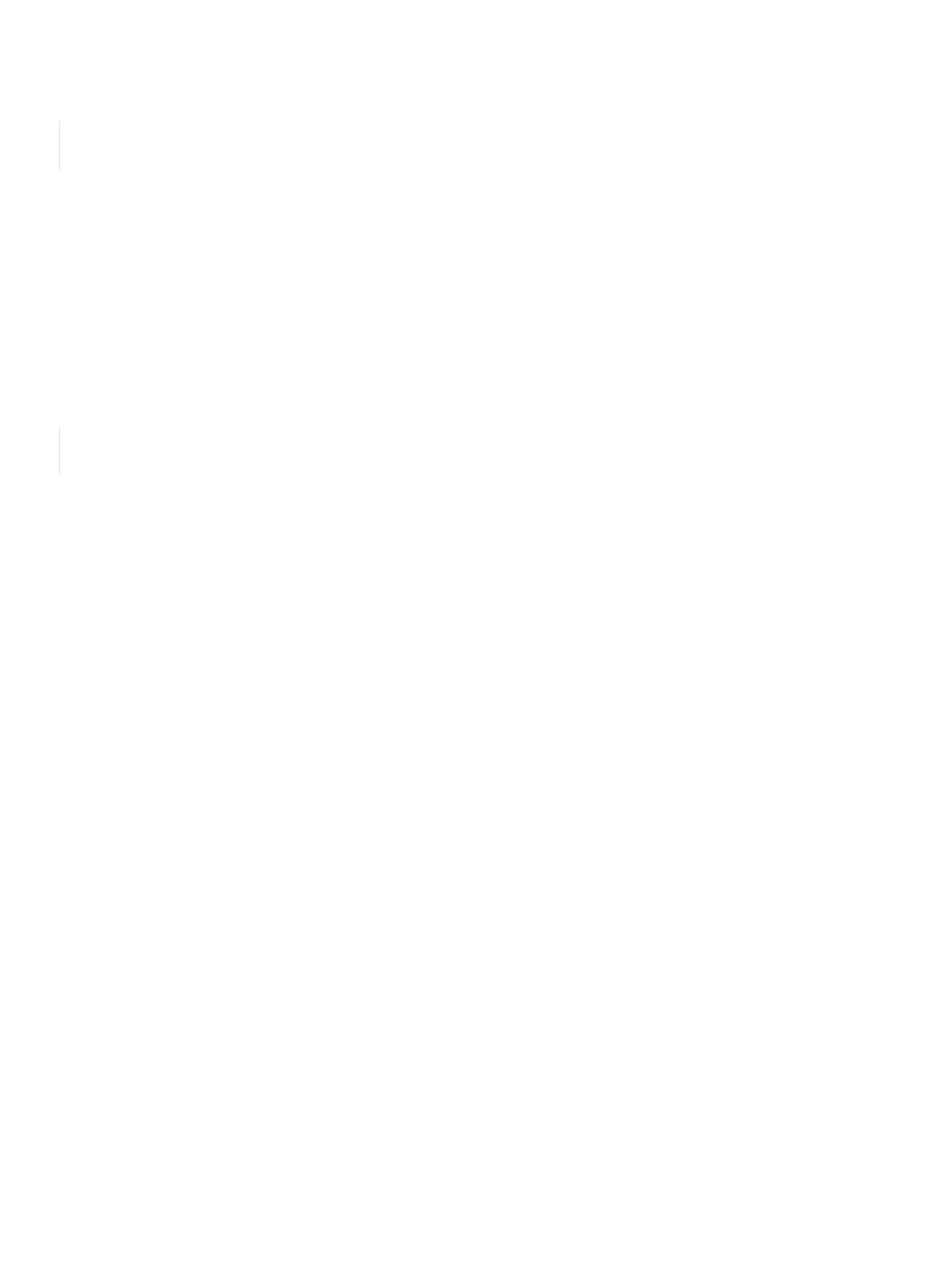









Search WWH ::

Custom Search