Java Reference
In-Depth Information
the candidate is divisible by a prime as soon as the next prime is greater than the candidate's
root. Unfortunately, there isn't such a method available in the Streams API. You could use
filter(p -> p <= candidateRoot) to filter the prime numbers smaller than the candidate root. But
filter would process the whole stream before returning the adequate stream. If both the list of
primes and the candidate number were very large, this would be problematic. You don't need to
do this; all you want is to stop once you find a prime that's greater than the candidate root!
Therefore, you'll create a method called takeWhile, which, given a sorted list and a predicate,
returns the longest prefix of this list whose elements satisfy the predicate:
Using this method, you can optimize the isPrime method by testing only the candidate prime
against only the primes that are not greater than its square root:
public static boolean isPrime(List<Integer> primes, int candidate){
int candidateRoot = (int) Math.sqrt((double) candidate);
return takeWhile(primes, i -> i <= candidateRoot)
.stream()
.noneMatch(p -> candidate % p == 0);
}
Note that this is an eager implementation of takeWhile. Ideally you'd like a lazy version of
takeWhile so it can be merged with the noneMatch operation. Unfortunately, implementing it
would be beyond the scope of this chapter because you'd need to get a grip on the Streams API
implementation.
With this new isPrime method in hand, you're now ready to implement your own custom
collector. First, you need to declare a new class that implements the Collector interface. Then,
you need to develop the five methods required by the Collector interface.
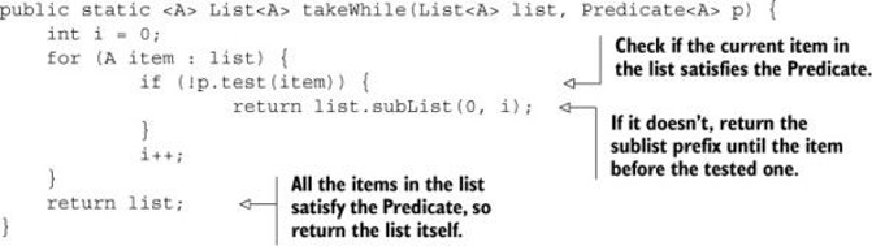
Search WWH ::

Custom Search