Java Reference
In-Depth Information
Like the other bitwise operators, the three bitwise shift operators manipulate
the individual bits of an integer value. They all take two operands. The left oper-
and is the value whose bits are shifted; the right operand specifies how many
positions they should move. Prior to performing a shift,
byte
and
short
values
are promoted to
int
for all shift operators. Furthermore, if either of the operands
is
long
, the other operand is promoted to
long
. For readability, we use only 16
bits in the examples in this section, but the concepts are the same when carried
out to 32- or 64-bit strings.
When bits are shifted, some bits are lost off one end, and others need to be
filled in on the other. The
left-shift
operator (
<<
) shifts bits to the left, filling the
right bits with zeros. For example, if the integer variable
number
currently has the
value 13, then the statement
number = number << 2;
stores the value 52 into
number
. Initially,
number
contains the bit string
0000000000001101
. When shifted to the left, the value becomes
0000000000110100
,
or 52. Notice that for each position shifted to the left, the original value is
multiplied by 2.
The sign bit of a number is shifted along with all of the others. Therefore
the sign of the value could change if enough bits are shifted to change the
sign bit. For example, the value −8 is stored in binary two's complement
form as
1111111111111000
. When shifted left two positions, it becomes
1111111111100000
, which is −32. However, if enough positions are shifted, a
negative number can become positive and vice versa.
There are two forms of the right-shift operator: one that preserves the sign of
the original value (
>>
) and one that fills the leftmost bits with zeros (
>>>
).
Let's examine two examples of the
right-shift-with-sign-fill
operator. If the
int
variable
number
currently has the value 39, the expression
(number >> 2)
results
in the value 9. The original bit string stored in
number
is
0000000000100111
, and
the result of a right shift two positions is
0000000000001001
. The leftmost sign
bit, which in this case is a zero, is used to fill from the left.
If
number
has an original value of −16, or
1111111111110000
, the right-
shift (with sign fill) expression
(number >> 3)
results in the binary string
1111111111111110
, or −2. The leftmost sign bit is a 1 in this case and is used to
fill in the new left bits, maintaining the sign.
If maintaining the sign is not desirable, the
right-shift-with-zero-fill
operator
(
>>>
) can be used. It operates similarly to the
>>
operator but fills with zero no
matter what the sign of the original value is.
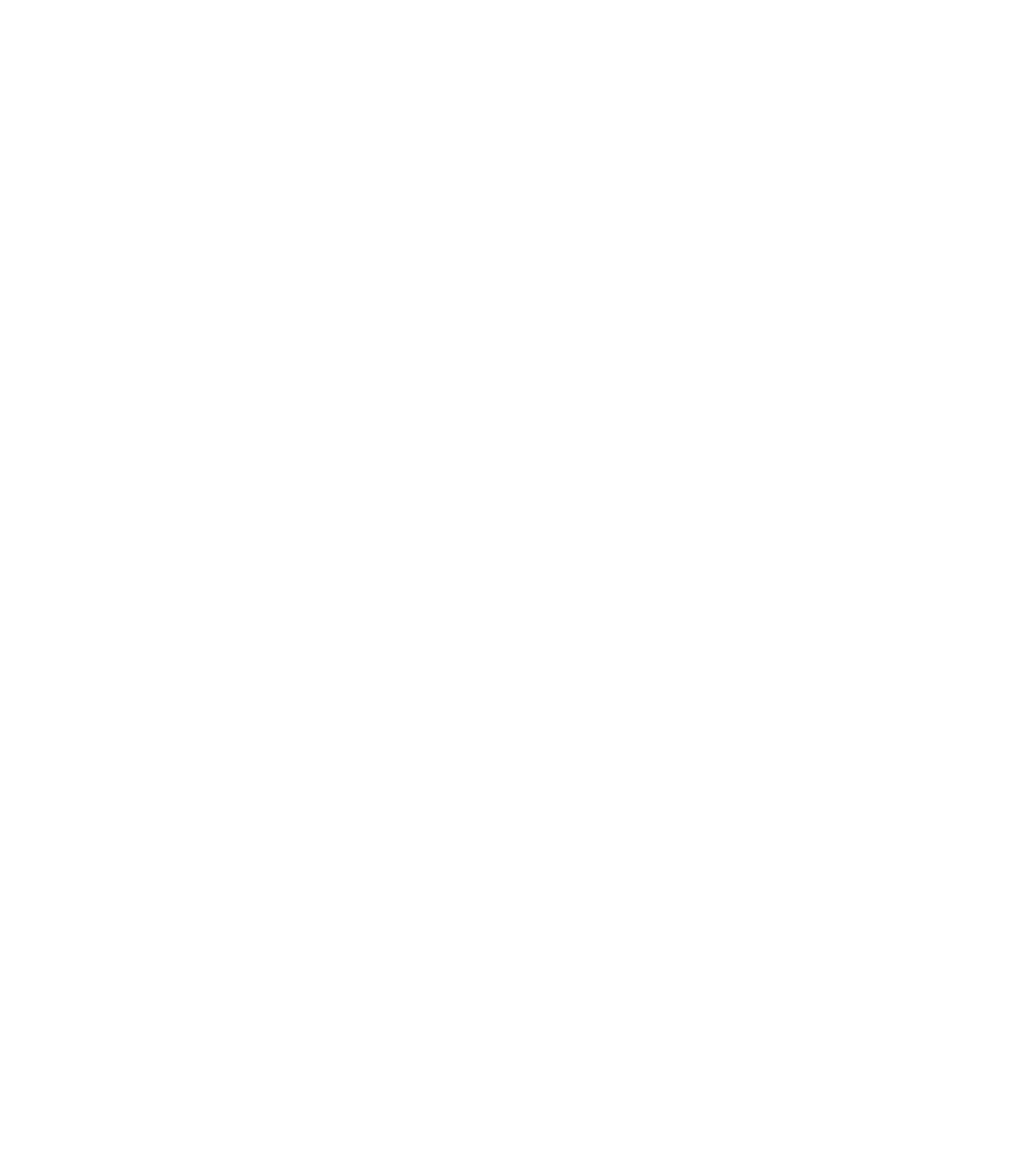
Search WWH ::

Custom Search