Java Reference
In-Depth Information
LISTING 10.9
//********************************************************************
// Sorting.java Author: Lewis/Loftus
//
// Demonstrates the selection sort and insertion sort algorithms.
//********************************************************************
public class
Sorting
{
//-----------------------------------------------------------------
// Sorts the specified array of objects using the selection
// sort algorithm.
//-----------------------------------------------------------------
public static void
selectionSort (Comparable[] list)
{
int
min;
Comparable temp;
for
(
int
index = 0; index < list.length-1; index++)
{
min = index;
for
(
int
scan = index+1; scan < list.length; scan++)
if
(list[scan].compareTo(list[min]) < 0)
min = scan;
// Swap the values
temp = list[min];
list[min] = list[index];
list[index] = temp;
}
}
//-----------------------------------------------------------------
// Sorts the specified array of objects using the insertion
// sort algorithm.
//-----------------------------------------------------------------
public static void
insertionSort (Comparable[] list)
{
for
(
int
index = 1; index < list.length; index++)
{
Comparable key = list[index];
int
position = index;
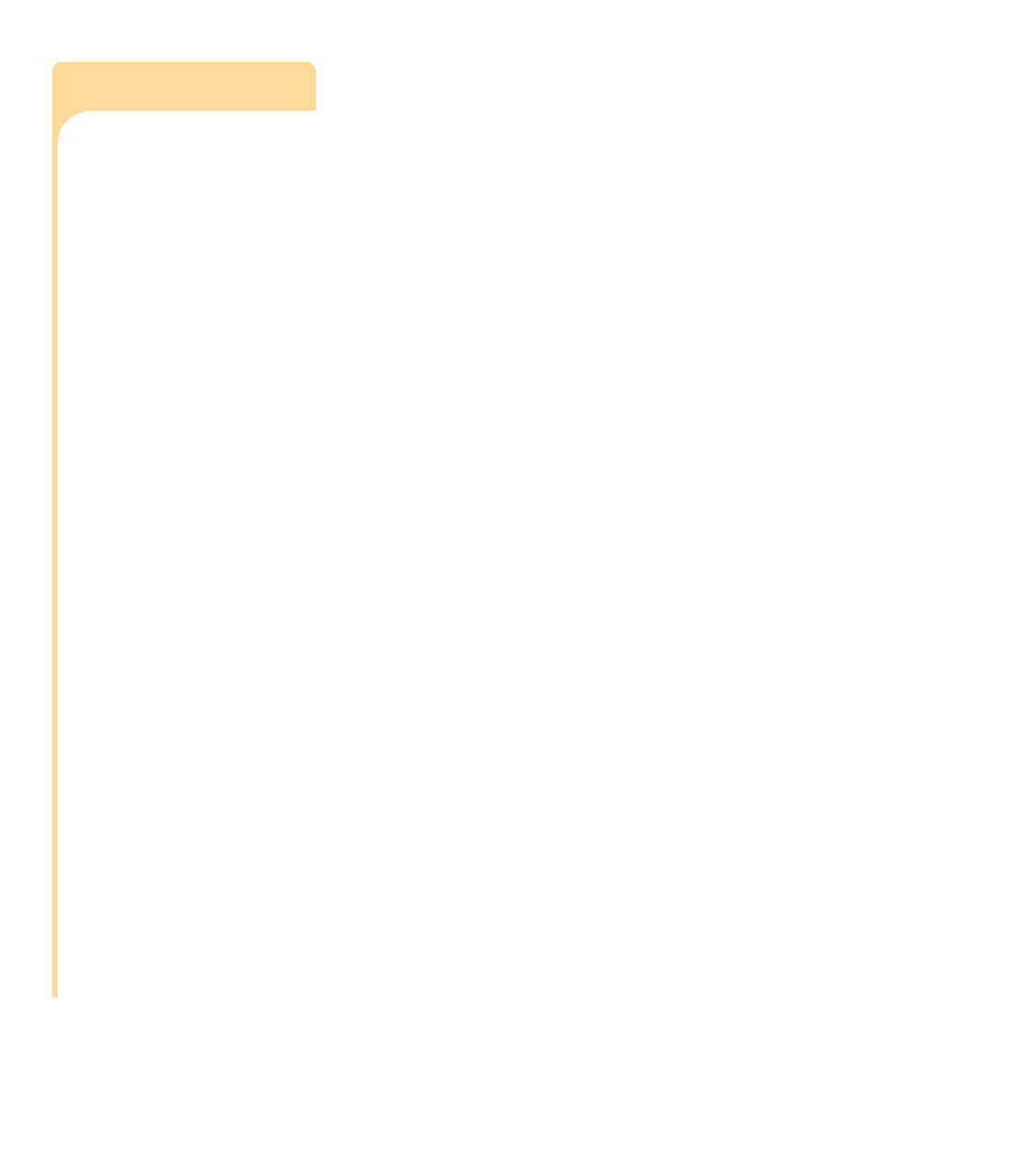
Search WWH ::

Custom Search