Java Reference
In-Depth Information
SELF-REVIEW QUESTIONS
(see answers in Appendix N)
SR 10.1
What is polymorphism?
SR 10.2
Why is compile time binding considered more efficient than dynamic
binding?
When we declare a reference variable using a particular class name, it can be used
to refer to any object of that class. In addition, it can also refer to any object of
any class that is related to its declared type by inheritance. For example, if the
class
Mammal
is the parent of the class
Horse
, then a
Mammal
reference
can be used to refer to any object of class
Horse
. This ability is shown
in the following code segment:
KEY CONCEPT
A reference variable can refer to any
object created from any class related
to it by inheritance.
Mammal pet;
Horse secretariat =
new
Horse();
pet = secretariat;
// a valid assignment
The reverse operation, assigning the
Mammal
object to a
Horse
reference, can
also be done but it requires an explicit cast. Assigning a reference in this direc-
tion is generally less useful and more likely to cause problems, because although
a horse has all the functionality of a mammal (because a horse
is-a
mammal), the
reverse is not necessarily true.
This relationship works throughout a class hierarchy. If the
Mammal
class were
derived from a class called
Animal
, the following assignment would also be valid:
Animal creature =
new
Horse();
Carrying this to the limit, an
Object
reference can be used to refer to any
object, because ultimately all classes are descendants of the
Object
class. An
ArrayList
, for example, uses polymorphism in that it is designed to hold
Object
references. That's why an
ArrayList
that doesn't specify an element
type can be used to store any kind of object. In fact, a particular
ArrayList
can be used to hold several different types of objects at one time, because, by
inheritance, they are all
Object
objects.
The reference variable
creature
can be polymorphic, because at
any point in time it can refer to an
Animal
object, a
Mammal
object,
or a
Horse
object. Suppose that all three of these classes have a
method called
move
that is implemented in different ways (because
KEY CONCEPT
The type of the object, not the type
of the reference, is used to determine
which version of a method to invoke.
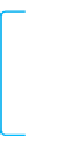
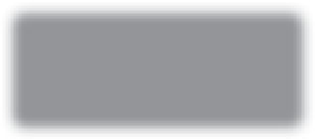
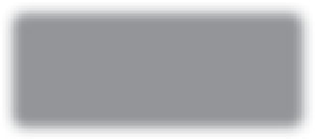

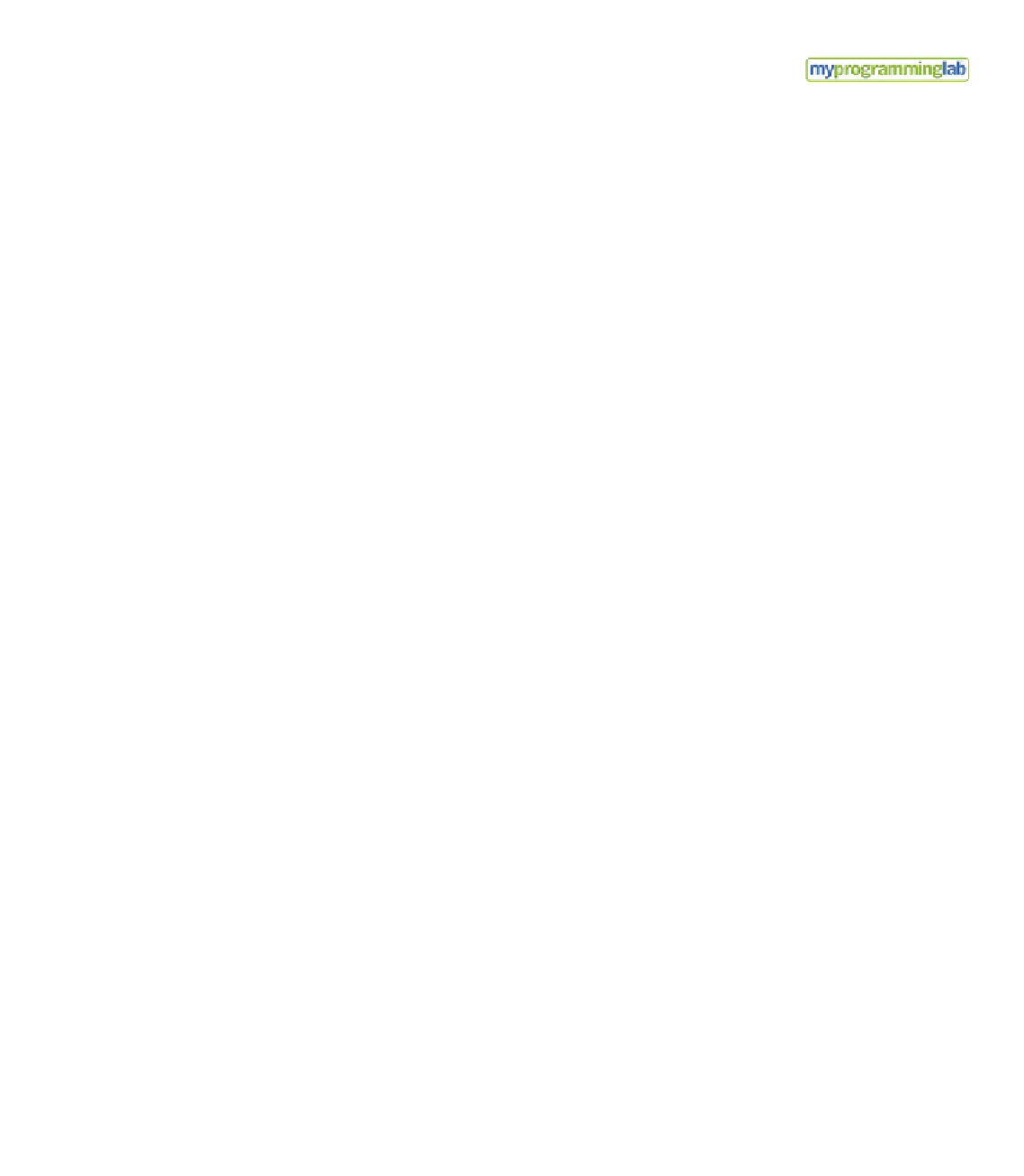
Search WWH ::

Custom Search