Java Reference
In-Depth Information
Java provides the ability to define an
enumerated type
, which can then be used as
the type of a variable when it is declared. An enumerated type establishes all pos-
sible values of a variable of that type by listing, or enumerating, them. The values
are identifiers, and can be anything desired.
For example, the following declaration defines an enumerated type called
Season
, whose possible values are
winter
,
spring
,
summer
, and
fall
:
enum
Season {winter, spring, summer, fall}
There is no limit to the number of values that you can list for an enumerated
type. Once the type is defined, a variable can be declared of that type:
Season time;
The variable
time
is now restricted in the values it can take on.
It can hold one of the four
Season
values, but nothing else. Java
enumerated types are considered to be
type-safe,
meaning that any
attempt to use a value other than one of the enumerated values will
result in a compile-time error.
The values are accessed through the name of the type. For example:
KEY CONCEPT
Enumerated types are type-safe,
ensuring that invalid values will not
be used.
time = Season.spring;
Enumerated types can be quite helpful in situations in which you have a rela-
tively small number of distinct values that a variable can assume. For example,
suppose we wanted to represent the various letter grades a student could earn. We
might declare the following enumerated type:
enum
Grade {A, B, C, D, F}
Any initialized variable that holds a
Grade
is guaranteed to have one of those valid
grades. That's better than using a simple character or string variable to represent
the grade, which could take on any value.
Suppose we also wanted to represent plus and minus grades, such as A- and
B+. We couldn't use A- or B+ as values, because they are not valid identifiers (the
characters
'-'
and
'+'
cannot be part of an identifier in Java). However, the same
values could be represented using the identifiers
Aminus
,
Bplus
, etc.
Internally, each value in an enumerated type is stored as an integer, which is
referred to as its
ordinal value
. The first value in an enumerated type has an ordi-
nal value of 0, the second one has an ordinal value of 1, the third one 2, and so on.
The ordinal values are used internally only. You cannot assign a numeric value to
an enumerated type, even if it corresponds to a valid ordinal value.

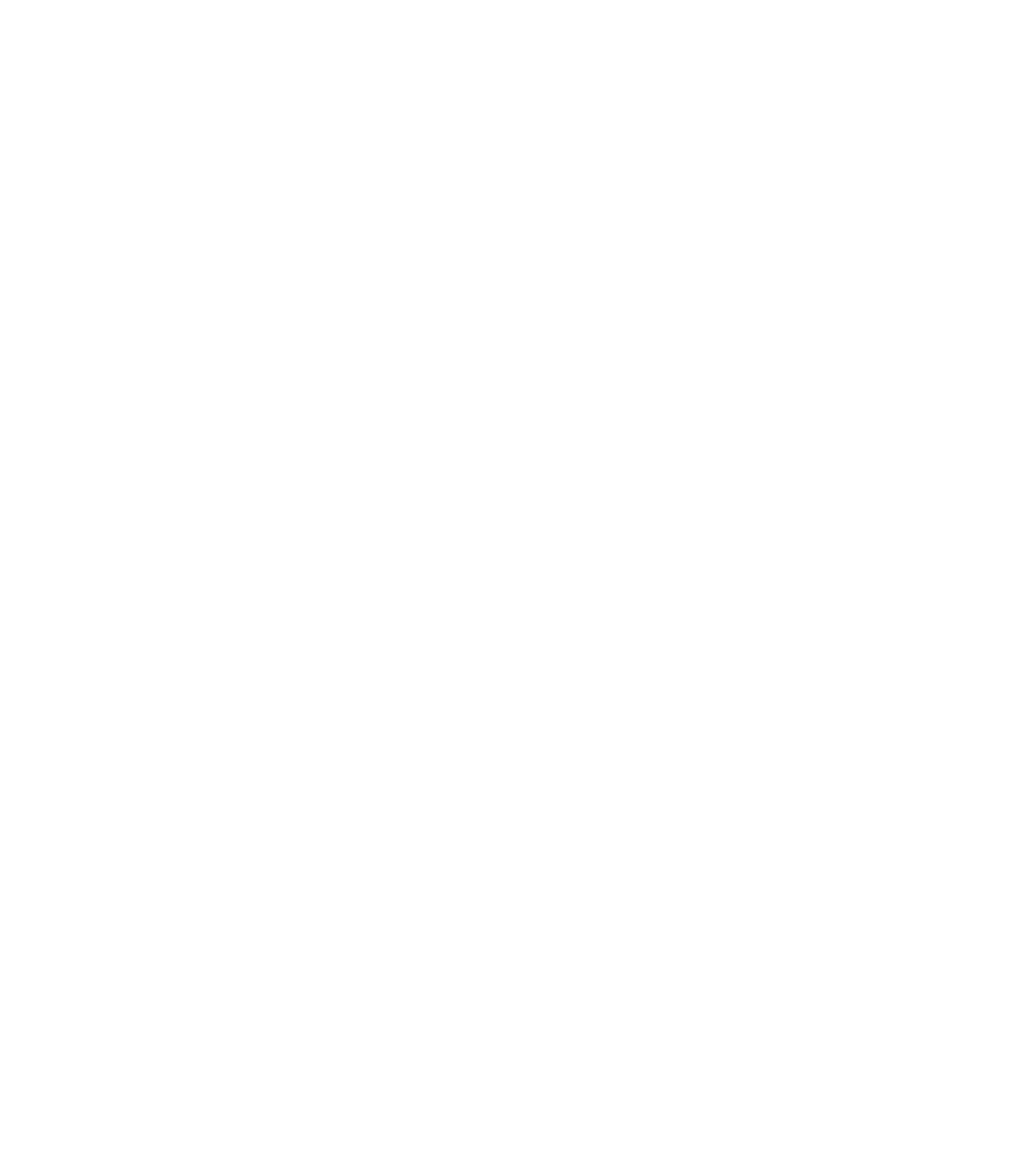
Search WWH ::

Custom Search