Game Development Reference
In-Depth Information
using
Microsoft.Xna.Framework;
1
using
Microsoft.Xna.Framework.Graphics;
2
using
Microsoft.Xna.Framework.Input;
3
4
5
class
Balloon : Game
{
6
GraphicsDeviceManager graphics;
7
SpriteBatch spriteBatch;
8
Texture2D balloon, background;
9
Vector2 balloonPosition;
10
11
12
static void
Main()
{
13
Balloon game =
new
Balloon();
14
game.Run();
15
}
16
17
18
public
Balloon()
{
19
Content.RootDirectory = "Content";
20
graphics =
new
GraphicsDeviceManager(
this
);
21
}
22
23
24
protected override void
LoadContent()
{
25
spriteBatch =
new
SpriteBatch(GraphicsDevice);
26
balloon = Content.Load<Texture2D>("spr_lives");
27
background = Content.Load<Texture2D>("spr_background");
28
}
29
30
31
protected override void
Update(GameTime gameTime)
{
32
MouseState currentMouseState = Mouse.GetState();
33
balloonPosition =
new
Vector2(currentMouseState.X, currentMouseState.Y);
34
}
35
36
37
protected override void
Draw(GameTime gameTime)
{
38
GraphicsDevice.Clear(Color.White);
39
spriteBatch.Begin();
40
spriteBatch.Draw(background, Vector2.Zero, Color.White);
41
spriteBatch.Draw(balloon, balloonPosition, Color.White);
42
spriteBatch.End();
43
}
44
}
45
Listing 5.1
A program that shows a balloon following the mouse
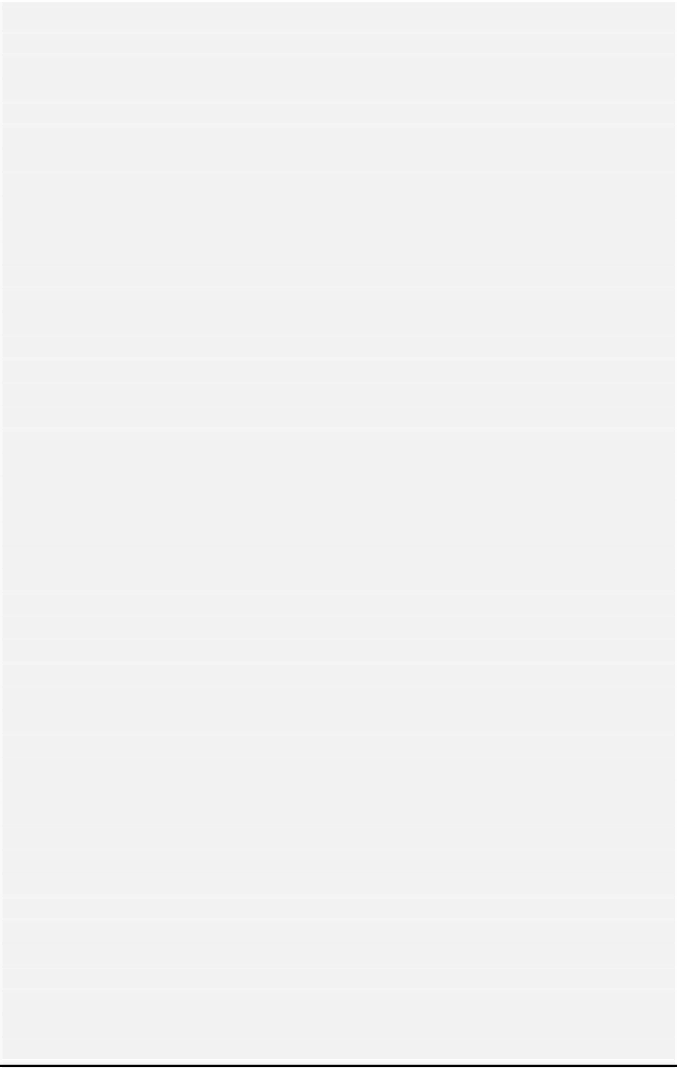
