Game Development Reference
In-Depth Information
class
Combination : Truck
{
public void
Draw(Game g,
int
x,
int
y)
{
// the truck
g.spriteBatch.Draw(g.truck,
new
Vector2(x, y
−
45), Color.Green);
g.spriteBatch.Draw(g.wheel,
new
Vector2(x + 5, y
−
10), Color.Red);
g.spriteBatch.Draw(g.wheel,
new
Vector2(x + 20, y
−
10), Color.Red);
−
g.spriteBatch.Draw(g.wheel,
new
Vector2(x + 55, y
10), Color.Red);
// the trailer
g.spriteBatch.Draw(g.connection,
new
Vector2(x
−
5, y
−
10), Color.Black);
−
45, y
−
45), Color.Green);
g.spriteBatch.Draw(g.trailer,
new
Vector2(x
−
40, y
−
10), Color.Red);
g.spriteBatch.Draw(g.wheel,
new
Vector2(x
−
20, y
−
10), Color.Red);
g.spriteBatch.Draw(g.wheel,
new
Vector2(x
}
}
(a) One declaration is still missing. Write down this declaration and indicate
where it should be placed in the program.
(b) Write down the missing part of the
Highway
constructor.
(c) Because the program still contains an error, nothing is drawn on the screen.
What error is this, and how can it be corrected?
(d) The programmer has duplicated quite some code with copy and paste. Why
is that not a good idea?
(e) How could this code copying have been avoided?
(f) We would like to continue improving the object-oriented approach of this
program, so that also the 'wheel' and 'trailer' concepts are modeled with
classes. How can that be done properly? Make sure that code duplication is
avoided as much as possible, and that a loose trailer can never end up on
the highway because of a programming error. You don't have to completely
implement this, just indicate which extra classes are needed, how they are re-
lated to their subclasses and which declarations are needed in new or existing
classes.
4.
Searching and sorting
(a) Write a method
Largest
which has as a parameter an array of doubles that
gives as a result the
largest value
that occurs in the array.
(b) Write another method
IndexLargest
which has as a result the index in the array
of the largest value. If there are more than one largest values in the array, the
method should return the index of the first one.
(c) Write a method
HowManySmallest
which has as a parameter an array of dou-
bles. The method should return how often the smallest value of the array
occurs in it. For example, if the array contains the values 9, 12, 9, 7, 12, 7, 8,
25, 7, then the result of the method call will be 3 because the smallest value
(7) occurs three times in the array.
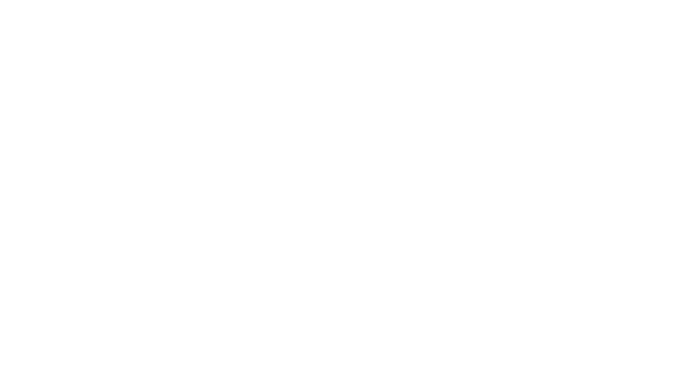