Game Development Reference
In-Depth Information
Fig. 27.1
Different types of collision: circle-circle, circle-rectangle, and rectangle-rectangle
uses
axis-aligned
bounding boxes, meaning that we do not consider boxes whose
sides are not parallel to the
x
- and
y
-axes.
Unfortunately, just doing collision detection using these bounding boxes is not
always precise enough. Especially when game objects are close to each other their
bounding shapes may intersect (and thus trigger a collision), but the actual objects
do not. Especially in the case when the game objects are animated, their shape
changes over time. Now you could make the bounding shape bigger so that the ob-
ject fits in it under all circumstances but that would lead to even more false collision
triggers.
A solution for this is to check for collisions on a per-pixel basis. So basically,
you could write an algorithm that walks over the non-transparent pixels in the sprite
(using a nested
for
-instruction), and checking if one or more of these pixels collide
with one of the pixels in another sprite (again, by walking through them using a
nested
for
-instruction). Fortunately, we will not have to perform this rather expensive
task very often. It only has to be done when two bounding shapes intersect. And then
we only have to do it for the parts of the shapes that actually intersect.
When we use circles and rectangles for detecting collisions, we need to handle
three different cases (see also Fig.
27.1
):
•
a circle intersects with another circle
•
a circle intersects with a rectangle
•
a rectangle intersects with another rectangle
The first case is the simplest one. The only thing we need to do is to check if the dis-
tance between the two centers is smaller than the sum of the radii. We have already
seen an example of how to do that.
For the case where a circle intersects a rectangle, we can use the following ap-
proach:
•
locate the point on the rectangle that lies closest to the circle center
•
calculate the distance between this point and the center of the circle
•
if this distance is smaller than the radius of the circle, there is a collision
Let us assume we want to find out if an object of type
Rectangle
intersects with a
circle, represented by an object of type
Vector2
and a radius. We can find the point
closest to the circle center by using
MathHelper.Clamp
in a smart way. Take a look at
the following code:
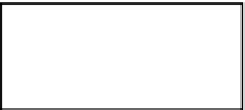
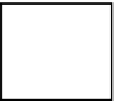
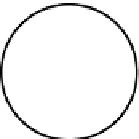


