Game Development Reference
In-Depth Information
catch
(Exception e)
{
spriteBatch.DrawString(s + "is not a number", Vector2.Zero, Color.Red);
}
In the
catch
-part the exception is 'caught'. Behind the word
catch
, there is some
kind of parameter. Through this parameter it is possible to find out what exactly
went wrong. In the case of
Parse
, there are not a lot of options, but the parameter
has to be declared in any case, even if we do not use it.
In the body of the
try
-instruction, multiple instructions may be placed. But as
soon as an exception occurs, the execution is halted and the body of the
catch
is
executed. The remaining instructions after the
try
may therefore assume that there
was no exception if they are executed.
Watch out that the bodies of the
try
and the
catch
part need to be between braces,
even if there is only a single instruction in the body. This is a bit illogical, given that
the braces may be omitted in for example the
if
or
while
instructions.
The
Exception
type, of which we declared a variable after the
catch
, is a class with
all kinds of subclasses:
FormatException
,
OverflowException
,
DivideByZeroException
, and
so on. Each subclass differs in the kind of details that may be obtained from them
through accessing the properties. Instead of using these subclasses, you could sim-
ply use the
Exception
type between the parentheses after the
catch
keyword as we
did in the example code. However, this will catch an exception of
any
type, and you
will not know what kind of exception it is. Therefore, always catch exceptions by
using the subclasses, unless you have a good reason not to.
It is allowed to place multiple
catch
parts with one
try
-instruction. You can give
each of these
catch
parts a different exception type. When an exception occurs, the
first
catch
with a corresponding exception type is selected. For example:
try
{
n=
int
.Parse(s);
}
catch
(FormatException e)
{
spriteBatch.DrawString(s + "is not a number", Vector2.Zero, Color.Red);
}
catch
(OverflowException e)
{
spriteBatch.DrawString(s + "is too big", Vector2.Zero, Color.Red);
}
After the
catch
part, it is possible to place a
finally
part, which is executed no matter
whether an exception of a certain kind is caught or not. For example:
try
{
n=
int
.Parse(s);

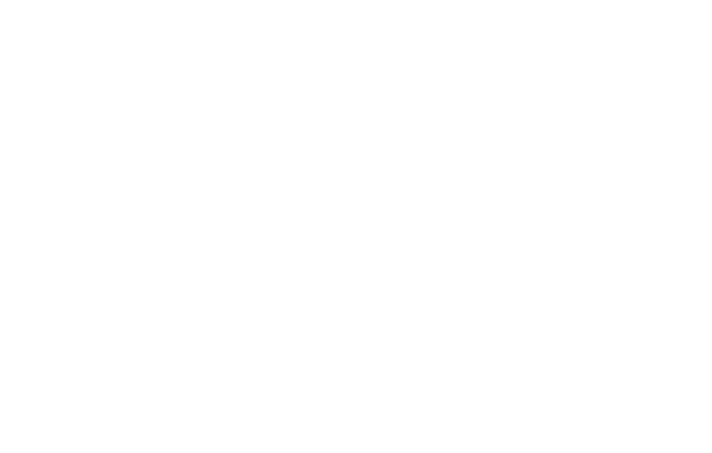