Game Development Reference
In-Depth Information
Fig. 23.4
The sprite containing all the possible pair images
and the colored pairs are ordered in the same way as the penguins (see Fig.
23.4
).
The rightmost image in the strip (sheet index equals seven) is the image we want
to display if the pair still needs to be made. So if the
pairs
array would contain
the values
{0,0,2,7,7}
then it would mean that the player made two pairs of blue
penguins, one pair of green penguins, and that the player would need to make two
more pairs in order to finish the level.
We pass along a parameter,
nrPairs
, to the constructor of the
PairList
class, so that
we know how large the array should be. We then fill the array so that each element
is set to the empty slot (sheet index equals seven):
pairs =
new int
[nrPairs];
for
(
int
i = 0; i< pairs.Length; i++)
pairs[i] = 7;
We also add a method
AddPair
to the class, which will find the first occurrence of
the value seven in the array and replace it with the index that was passed along as a
parameter:
public void
AddPair(
int
index)
{
int
i=0;
while
(i < pairs.Length && pairs[i] != 7)
i++;
if
(i < pairs.Length)
pairs[i] = index;
}
In this example, we used a
while
-instruction to increment the
i
variable until we find
an empty spot.
Now we will add a useful property to check whether we have completed the
level. The level is completed if the list of pairs no longer contains any value of
seven (meaning that all empty spots have been replaced by a pair):
public bool
Completed
{
get
{
for
(
int
i = 0; i < pairs.Length; i++)
if
(pairs[i] == 7)
return false
;
return true
;
}
}


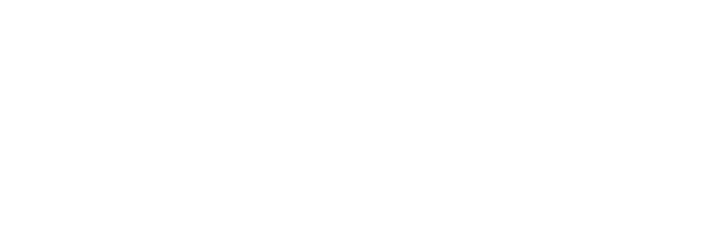