Game Development Reference
In-Depth Information
the player solved the level or not. As you can see, this contents represents the state
where the player starts the game for the first time. In other words: all levels but the
first one are locked, and none of the levels are solved.
We can load this status information after we have loaded all the levels, and up-
date all the
Level
objects. For that, we added a method
LoadLevelsStatus
method and
added it to the
PlayingState
class. In this method, we again create an instance of
the
StreamReader
class, and we read the lines from the text file. We use the
Split
method from the
string
type to split the lines into separate strings, and we then use
bool
.Parse
to convert these strings to boolean values. We then pass along these val-
ues to the
Locked
and
Solved
properties of the different levels. The complete method
is given as follows:
public void
LoadLevelsStatus(
string
path)
{
StreamReader fileReader =
new
StreamReader(path);
for
(
int
i = 0; i < levels.Count; i++)
{
string
line = fileReader.ReadLine();
string
[] elems = line.Split(',');
if
(elems.Length == 2)
{
levels[i].Locked =
bool
.Parse(elems[0]);
levels[i].Solved =
bool
.Parse(elems[1]);
}
}
fileReader.Close();
}
In a similar way, we can create a method
WriteLevelsStatus
that uses a
StreamWriter
to
write the current status of all the levels to a file. Here is the complete method:
public void
WriteLevelsStatus(
string
path)
{
StreamWriter fileWriter =
new
StreamWriter(path,
false
);
for
(
int
i = 0; i < levels.Count; i++)
{
string
line = levels[i].Locked.ToString() + "," + levels[i].Solved.ToString();
fileWriter.WriteLine(line);
}
fileWriter.Close();
}
In the
WriteLevelsStatus
method, we go the other way round. We create the writer
object, then we create the strings representing the status for each level, which are
then written to the file. We pass a boolean value as a parameter to the
StreamWriter
constructor to indicate that we do not want to
append
something to the file, but that
we want to
overwrite
the file with new contents. After writing the level status to the
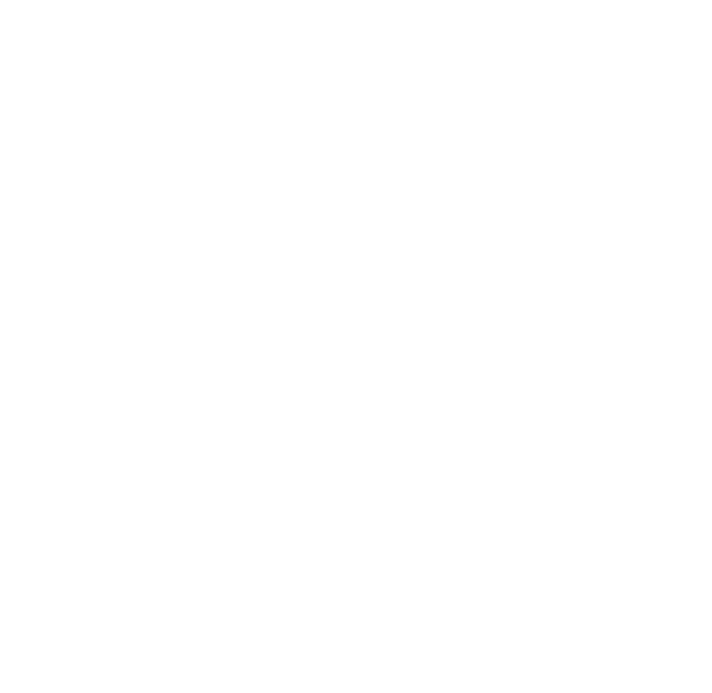