Game Development Reference
In-Depth Information
using
Microsoft.Xna.Framework;
1
using
Microsoft.Xna.Framework.Graphics;
2
3
4
enum
TileType { Normal, Background, Wall };
5
6
class
Tile : SpriteGameObject
{
7
protected
TileType type;
8
9
10
public
Tile(
string
assetname,
int
layer = 0,
string
id = "",
int
stripIndex = 0)
:
base
(assetname, layer, id, stripIndex)
11
{
12
type = TileType.Normal;
13
}
14
15
16
public override void
Draw(GameTime gameTime, SpriteBatch spriteBatch)
{
17
if
(type == TileType.Background)
18
return
;
19
base
.Draw(gameTime, spriteBatch);
20
}
21
22
23
public
TileType TileType
{
24
get
{
return
type; }
25
set
{type=
value
;}
26
}
27
}
28
Listing 22.1
The
Tile
class
Furthermore, we add a property to the
Tile
class to access the current tile type. When
we load the level, we will create a tile for each character, and store it in a grid
structure such as
GameObjectGrid
.
22.4 Other Level Information
Next to the tiles in the level, we need to store a few other things as well in the level
description text file:
•
the title of the level
•
the description of the level
•
the number of pairs to be made
•
the width and the height of the level
•
the location and direction of the hint arrow
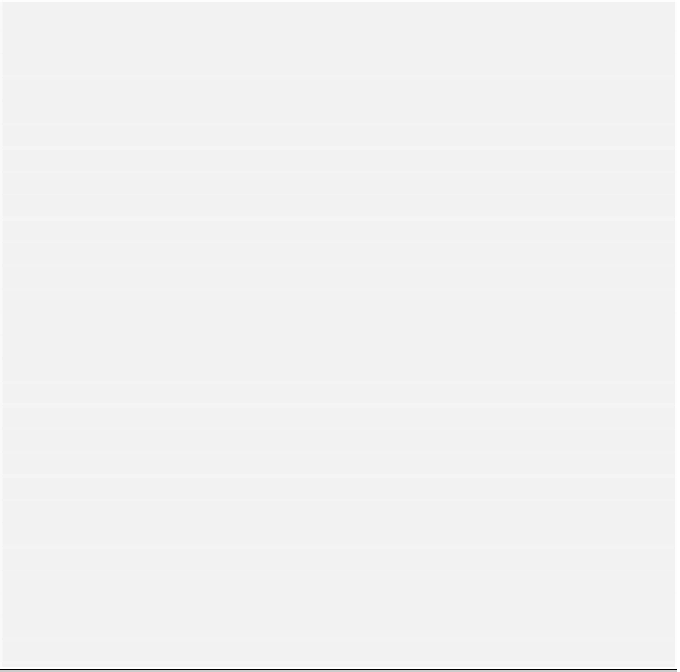
