Game Development Reference
In-Depth Information
Fig. 20.1
The sprite strip
used for the on/off button
20.3 Adding an on/off Button
The next step is adding an on/off button for being able to show a hint or not during
game play. How the value of the on/off button is used is something that we will
look into later on in this chapter. Just like we did for the
Button
class in the
Jewel
Jam
game, we are going to make a special class for on/off buttons, which we will
(surprisingly) call
OnOffButton
. The class is a subclass of
SpriteGameObject
and it will
expect a
sprite strip
containing two sprites: one for the 'off' state and one for the
'on' state (see Fig.
20.1
).
An important part of the button is that we need to be able to read and set whether
it is on or off. Since the button is based on a sprite strip of length two, we can define
the button to be in the 'off' state if the sheet index is zero, and in the 'on' state if the
sheet index equals one. We can then add a boolean property that gets and sets this
value (you can see the code for this property in Listing
20.1
).
Finally, we need to handle mouse clicks on the button to toggle its on or off state.
Similar to what we did in the
Button
class, we check in the
HandleInput
method if the
left mouse button was pressed and if the mouse position is within the bounding box
of the button. If that is the case, we modify the sheet index. If the sheet index is zero,
it should become one, and vice versa. We can achieve this effect with the following
instruction:
sprite.SheetIndex = 1
−
sprite.SheetIndex;
For the complete class, see Listing
20.1
.
In the
PenguinPairs
class, we add an
OnOffButton
instance to the game world, at the
desired position:
onOffButton =
new
OnOffButton("Sprites/spr_button_offon@2");
onOffButton.Position =
new
Vector2(650, 340);
gameWorld.Add(onOffButton);
20.4 Adding a Slider Button
A second kind of GUI control we would like to add is a slider. This slider will
be used to control the volume of the background music in the game. The slider will
consist of two sprites: a back sprite that represents the bar, and a front sprite that rep-
resents the actual slider. Therefore, the
Slider
class will inherit from
GameObjectList
.
Because the back sprite has a border, we need to take that into account when we
move or draw the slider. Therefore, we also define left and right margins that define

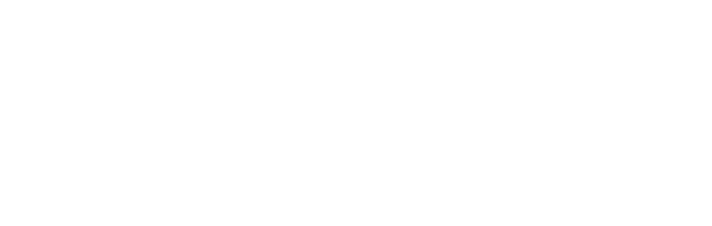