Game Development Reference
In-Depth Information
Vector2 glitterCenter =
new
Vector2(glitter.Width, glitter.Height) / 2;
Then, we add a
for
-instruction that traverses all the scales and positions of the glitters
to be drawn. What we still need to do here, is calculate the real scale value based
on the scale value that was stored in the array in the
Update
method. If that value is
between 0 and 1, we do not have to do anything (scale is increasing). If the value is
between 1 and 2, we need to convert that value into a decreasing scale. This is done
using the following instructions:
float
scale = scales[i];
if
(scales[i] > 1)
scale = 2
−
scales[i];
The only thing left to do now is to draw the glitter at the desired position, with the
desired scale, using the
SpriteBatch.Draw
method:
spriteBatch.Draw(glitter,
this
.GlobalPosition + positions[i],
null
,
Color.White, 0f, glitterCenter, scale, SpriteEffects.None, 0);
For the complete
GlitterField
class, have a look at the
JewelJam7
program in the solu-
tion belonging to this chapter.
18.4.6 Adding Glitters to Game Objects
Now that we have made the generic
GlitterField
class, we can make a few simple
extensions to our game objects to add glitters to them. We want to do this with taste,
and not blind the player with glitters. So, we will add some glitters to each of the
jewels in the playing field, as well as the moving jewel cart. First, let us have a look
at how to extend the
Jewel
class to add glitters to it. The first step is adding a new
member variable to the class that contains a reference to the glitter field:
protected
GlitterField glitters;
In the constructor, we give this glitter field a value. We also make sure that its parent
is set to the
Jewel
object. That way, if the jewel is moved in the playing field, the
glitters move along with it:
glitters =
new
GlitterField(
this
.Sprite, 2, sprite.Height, sprite.Height,
variation
∗
sprite.Height);
glitters.Parent =
this
;
You can also see that we calculate the
x
offset using the
variation
member variable.
That way, the glitter field knows on which jewel the glitters are drawn. In the
Update
method of the
Jewel
class, we then have to make sure that the glitter field is updated
as well:
glitters.Update(gameTime);


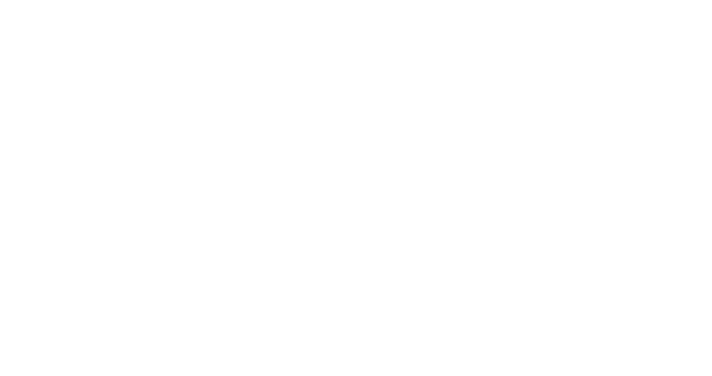