Game Development Reference
In-Depth Information
using
Microsoft.Xna.Framework;
1
2
3
class
JewelCart : SpriteGameObject
{
4
protected float
push;
5
protected float
minxpos;
6
7
8
public
JewelCart(
int
layer = 0,
string
id = "")
:
base
("spr_jewelcart", layer, id)
9
{
10
velocity.X = 6;
11
push = 50.0f;
12
}
13
14
15
public void
Push()
{
16
−
position.X = MathHelper.Max(position.X
push, minxpos);
17
}
18
19
20
public float
MinXPos
{
21
get
{
return
minxpos; }
22
set
{ minxpos =
value
;}
23
}
24
}
25
Listing 16.3
A game object for displaying a slowly moving jewel cart
16.4.2 Finding Valid Combinations of Jewels
Inside the
HandleInput
method, we need to check for all groups of three adjacent
jewels to see if they form a valid combination. In order to help us do that, we are
going to add a method
IsValidCombination
, which has the following header:
public bool
IsValidCombination(Jewel a, Jewel b, Jewel c)
This method takes three
Jewel
objects, and it returns a boolean value indicating if the
three jewels form a valid combination or not. So now the question is: how can we
evaluate whether three jewels form a valid combination? To recall: a valid combi-
nation means that for each property (color, shape, number), each jewel should have
either the same or a different value. In order to make things a bit easier, let us
encode
each symbol by using three integers between 0-2. Let us say that the first integer
represents the color (yellow, blue, or red), the second integer the shape (diamond,
oval or round), and the last integer the number of jewels (one, two or three). Us-
ing this encoding scheme, we can, for example, encode the blue oval-shaped single
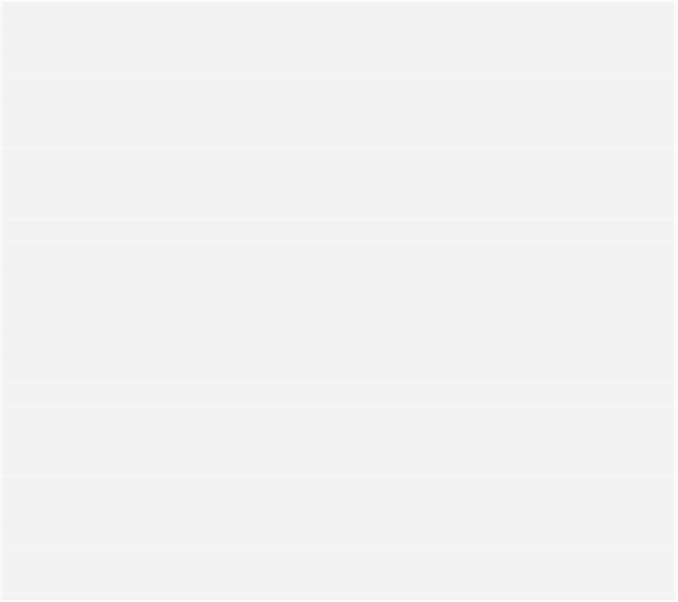


