Game Development Reference
In-Depth Information
return
parent.Root;
else
return this
;
}
}
The code inside the property is very simple. If our current parent is not
null
(meaning
we have a parent), we ask that parent for the root game object. If the parent is
null
it means that the game object we are currently manipulating is at the root of the
hierarchy, meaning that it is the game world. For convenience, we add an extra
property to retrieve the game world as a
GameObjectList
instance which relies on the
Root
property:
public
GameObjectList GameWorld
{
get
{
return
Root
as
GameObjectList;
}
}
Assumptions in software design—
We made quite a few assumptions in these
last paragraphs. For one, we assume that every game object is part of a hier-
archy so that we can use that hierarchy to find the game world. This can lead
to problems. For example, suppose that you already need access to the game
world in the constructor. Chances are that the game object we are construct-
ing is not yet added to a hierarchy so its parent will still be
null
. In the current
design, there is no way to access the game world in the constructor, unless we
explicitly pass it along as a parameter. Here you see that we made a trade-off
in the software design. We will not have to declare the game world as a static
variable using our technique, but the price we pay is that we sometimes may
have to pass the game world as a parameter to a method. Another assumption
is that the object at the root of the hierarchy always is of type
GameObjectList
.
However, you could image that someone would like to use the
GameObjectGrid
type as the root of a game world. Our current design does not cater for this.
One way to solve it would be to redesign the
GameObjectGrid
class so that it
inherits from the
GameObjectList
class. We would not use a two-dimensional
array in that case to store the grid, but we would need to write a few instruc-
tions that deal with the translation between two-dimensional coordinates and
their actual index in the
gameObjects
member variable. Even better would be
to make a distinction between the hierarchy that game objects are in, and
the
layout
that they should follow. In the
GameObjectGrid
class, these two are
currently mixed since it serves both as a game object container, and as a de-
scription of the layout for the game objects (which is a grid).

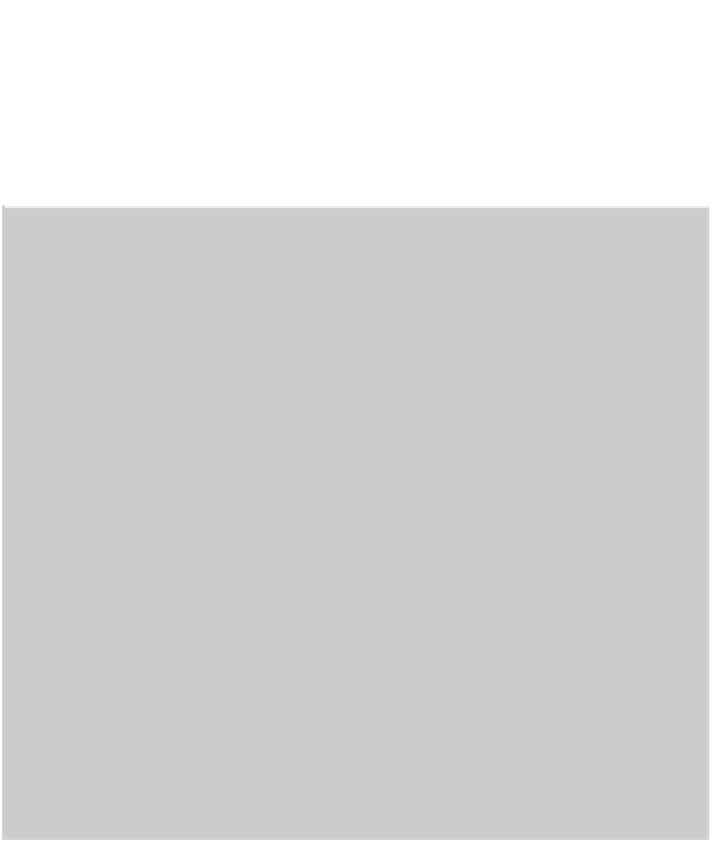


