Game Development Reference
In-Depth Information
if
(grid[x, y] ==
null
)
{
grid[x, y] = obj;
obj.Parent =
this
;
obj.Position =
new
Vector2(x
∗
cellWidth, y
∗
cellHeight);
return
;
}
}
14.4.4 A Grid of Jewels
For the
Jewel Jam
game, we would like to perform a couple of basic operations on
the grid. For one, we would like to be able to go to a row and shift the elements in the
row to the left or to the right. For example, when the player selects the third row in
the grid and presses the left button, all elements except the leftmost one should shift
to the left, and the leftmost element becomes the rightmost one. Since this kind of
operation is not something that we need in every game that uses a grid, let us create
aclass
JewelGrid
that inherits from
GameObjectGrid
and then we add the operations
we need to that class. The basic structure of this class is given as follows:
class
JewelGrid : GameObjectGrid
{
public
JewelGrid(
int
rows,
int
columns,
int
layer = 0)
:
base
(rows, columns, layer)
{
}
// here we add the row shifting methods
}
The way we implement shifting the columns in a row to the left, is by storing
the first element in a temporary object, then moving the other objects one column
to the left, and finally placing the element stored in the temporary object in the last
column. We add a method
ShiftRowLeft
that does exactly this. Because the method is
only applied to one row, we have to pass the row index as a parameter. The complete
method is given as follows:
public void
ShiftRowLeft(
int
rowIndex)
{
GameObject firstObj = grid[0, rowIndex];
for
(
int
x=0;x<Columns
−
1; x++)
grid[x, rowIndex] = grid[x + 1, rowIndex];
grid[Columns
−
1, rowIndex] = firstObj;
firstObj.Position =
new
Vector2(Columns
∗
objsize, rowIndex
∗
objsize);
}
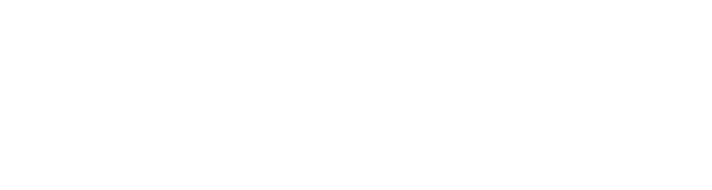
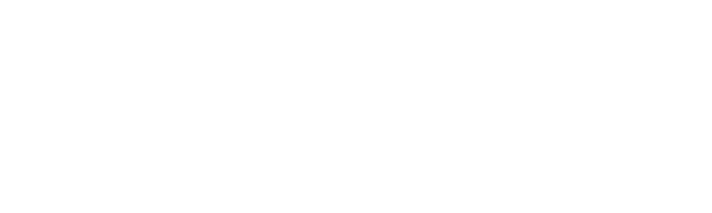
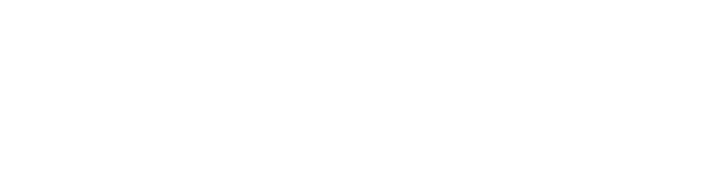