Game Development Reference
In-Depth Information
using
System.Collections.Generic;
1
using
Microsoft.Xna.Framework;
2
using
Microsoft.Xna.Framework.Graphics;
3
4
5
class
GameObjectList : GameObject
{
6
protected
List<GameObject> gameObjects;
7
8
9
public
GameObjectList(
int
layer = 0) :
base
(layer)
{ gameObjects =
new
List<GameObject>();
10
}
11
12
13
public void
Add(GameObject obj)
{ obj.Parent =
this
;
14
for
(
int
i = 0; i < gameObjects.Count; i++)
15
if
(gameObjects[i].Layer > obj.Layer)
16
{
17
gameObjects.Insert(i, obj);
18
return
;
19
}
20
gameObjects.Add(obj);
21
}
22
23
24
public void
Remove(GameObject obj)
{ gameObjects.Remove(obj);
25
obj.Parent =
null
;
26
}
27
28
29
public override void
HandleInput(InputHelper inputHelper)
{
foreach
(GameObject obj
in
gameObjects)
30
obj.HandleInput(inputHelper);
31
}
32
33
34
public override void
Update(GameTime gameTime)
{
foreach
(GameObject obj
in
gameObjects)
35
obj.Update(gameTime);
36
}
37
38
39
public override void
Draw(GameTime gameTime, SpriteBatch spriteBatch)
{
if
(!visible)
40
return
;
41
List<GameObject>.Enumerator e = gameObjects.GetEnumerator();
42
while
(e.MoveNext())
43
e.Current.Draw(gameTime, spriteBatch);
44
}
45
46
47
public override void
Reset()
{
foreach
(GameObject obj
in
gameObjects)
48
obj.Reset();
49
}
50
}
51
Listing 14.2
The
GameObjectList
class
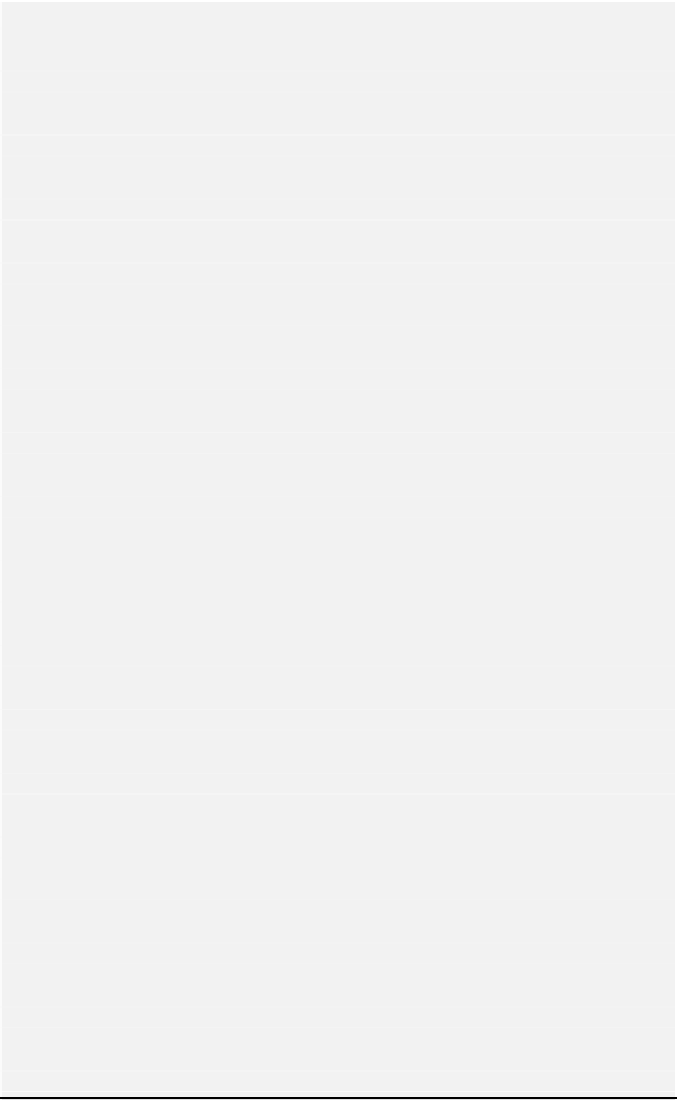
