Game Development Reference
In-Depth Information
Now we can use an
if
-instruction in each of the game loop methods to determine
what we should do. If the game is over, we do not want the cannon and the ball to
handle input anymore, we simply want to listen if the player presses space. If the
player presses the space bar, we reset the game. So, our new
HandleInput
method
contains the following instructions:
if
(lives > 0)
{
cannon.HandleInput(inputHelper);
ball.HandleInput(inputHelper);
}
else if
(inputHelper.KeyPressed(Keys.Space))
Reset();
We added a
Reset
method to the
GameWorld
class, so that we can reset the game to
its initial state. This means resetting all the game objects. We also need to reset the
allowed number of missed cans to 15 again. Finally, we need to reset the minimal
velocity of each paint can to its original value. For that, we added an extra method
ResetMinVelocity
to the
PaintCan
class. The full
Reset
method in the
GameWorld
class
then becomes
public void
Reset()
{
lives = 5;
cannon.Reset();
ball.Reset();
can1.Reset();
can2.Reset();
can3.Reset();
can1.ResetMinVelocity();
can2.ResetMinVelocity();
can3.ResetMinVelocity();
}
For the
Update
method, we only need to update the game objects if the game is
not over. Therefore, we first check with an
if
-instruction if we need to update the
game objects. If not (in other words: the number of lives is zero or less), we return
from the method:
if
(lives <= 0)
return
;
ball.Update(gameTime);
can1.Update(gameTime);
can2.Update(gameTime);
can3.Update(gameTime);
Finally, in the
Draw
method we draw the game objects, and the game over screen if
the player has no more lives left. This results in the following structure:

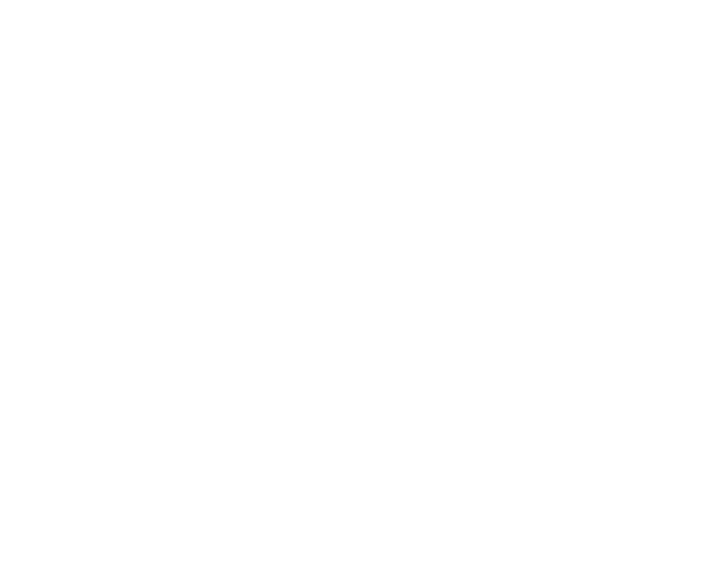