Game Development Reference
In-Depth Information
member variable
shooting
. In total, the
Ball
class will have the following member
variables:
Texture2D colorRed, colorGreen, colorBlue;
Texture2D currentColor;
Vector2 position, velocity;
Color color;
bool
shooting;
In the games that we develop in this topic, most of the objects will have a position
and a velocity. Since we only concern ourselves with 2D games, both the position
and the velocity will always be of type
Vector2
. When we update these game objects,
we need to calculate the new position based on the velocity vector and the time that
has passed. Later on, we will see how we can do that using the parameter
gameTime
that is passed to the
Update
method of the game object.
8.2.2 Initializing the Ball
In the constructor method of the
Ball
class, we have to assign values to the member
variables, just as in the case of the
Cannon
class. We pass the content manager as
a parameter, so that we can load the required sprites in the constructor. Then, we
assign values to the other member variables. When the game starts, the ball should
not be moving. Therefore, we set the velocity of the ball to zero (
Vector2.Zero
). Also,
we initially set the ball to a position so that it is hidden behind the cannon. That way,
when the ball is not moving, we do not see it. Of course, we have to make sure that
the ball is drawn before the cannon in that case. We initially set the color of the ball
to blue (
Color.Blue
), and we set the
shooting
member variable to
false
. The complete
constructor then becomes
public
Ball(ContentManager Content)
{
this
.colorRed = Content.Load<Texture2D>("spr_ball_red");
this
.colorGreen = Content.Load<Texture2D>("spr_ball_green");
this
.colorBlue = Content.Load<Texture2D>("spr_ball_blue");
position =
new
Vector2(65, 390);
velocity = Vector2.Zero;
shooting =
false
;
Color = Color.Blue;
}
As you can see in the last instruction of the constructor, we use the
Color
property.
We added this property to the
Ball
class so that we can retrieve and change the color
of the ball. And just like the
Cannon
class, we added a
Reset
method so that we can
reset the ball to its initial state. Have a look at the source code in the
Painter5
program
to see the complete
Ball
class.

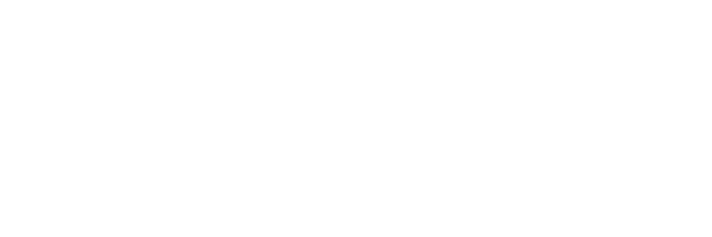