Game Development Reference
In-Depth Information
the game objects. We add a
Draw
method to the
GameWorld
class, which is called
from the
Painter
class, where we pass a sprite batch as a parameter. The
Draw
method
in the
Painter
class then contains only a few instructions:
protected override void
Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.White);
gameWorld.Draw(gameTime, spriteBatch);
}
The
Draw
method in the
GameWorld
class then basically asks all the game objects to
draw themselves. Before drawing the game objects, the background sprite is drawn.
So, the instructions in the
Draw
method are:
spriteBatch.Begin();
spriteBatch.Draw(background, Vector2.Zero, Color.White);
cannon.Draw(gameTime, spriteBatch);
spriteBatch.End();
You may think it is overkill to define a separate
GameWorld
class for these few
tasks. We could choose to leave them in the
Painter
class. Again, this is a design
choice. Why did we do this? One reason is because it separates the handling of
the actual game objects from generic game management such as initializing the
graphics device and creating a sprite batch. This is a good thing, because later on
in this topic, both tasks are going to become more complicated. There will be extra
game management code (for example for going to full screen mode and back), and
the later game examples will have many more game objects.
Use static objects sparingly—
Making the
gameWorld
object static is another
design choice that makes it easier to access the object in different classes later
on. If we did not declare the object as static, we would have to pass it as a
parameter to every method that would need it. This would needlessly compli-
cate the way that the other classes are designed, because it would introduce
an extra parameter in all of these methods. So, why not make every variable
static then? We would not need parameters at all! This is not a good idea. By
making every variable static, we create the impression that we will only ever
need a single instance of that type. As a result, you are basically ignoring half
of the power that object-oriented programming offers. Actually, you can use
the fact that an object is not always easily accessible to your advantage. For
instance, we have chosen not to make the
inputHelper
object static, but pass it
along as a parameter to the
HandleInput
methods. By doing that, we enforce a
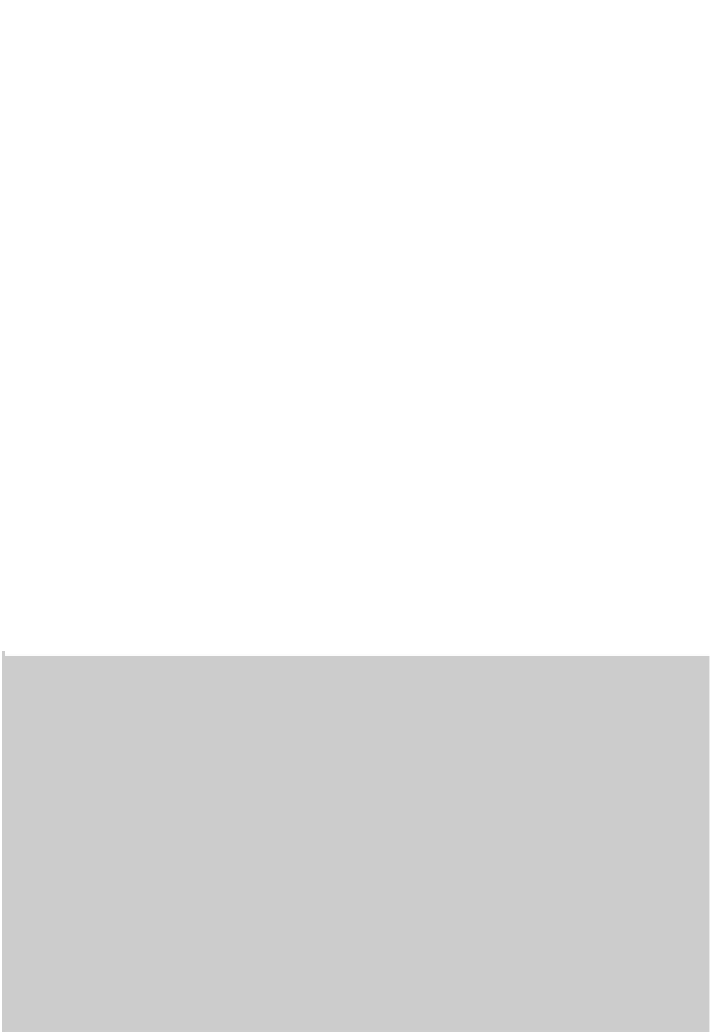


