Game Development Reference
In-Depth Information
public float
Square(
float
x)
{
return
x
∗
x;
}
If we look at the header of this method, we see that it takes one parameter of type
float
. The type
float
is also written in front of the method name. This means that the
type of the return value
is
float
, i.e., when we call this function, we can store the
result in a variable of type
float
:
float
f = Square(10.0f);
After this instruction is executed, the variable
f
will contain the value 100. So, the
type indicator in front of the method name tells us which type the return value of
this method has. Inside the method body, we can indicate using the keyword
return
what the actual value is that the method returns. In the case of
Square
, the method
returns the outcome of the expression
x
∗
x
. Of course, the type of the expression
after the
return
keyword needs to be the same type as the type written in front of the
method name, which in this case is
float
. Note that executing the
return
instruction
also terminates executing the rest of the instructions in a method. Any instructions
placed
after
the
return
instruction will not be executed. For example, consider the
following method:
public int
SomeMethod()
{
return
12;
int
tmp = 45;
}
In this example, the second instruction (
int
tmp = 45;
) will never be executed because
the instruction before it will end the method. This is a very handy feature of the
return
instruction, and we can use it to our advantage:
public float
SquareRoot(
float
f)
{
if
(f < 0.0f)
return
0.0f;
Calculatethesquareroot,wearenowsurethat
f
≥
0
.
}
In this example, we use the
return
instruction as a safeguard against wrong input by
the user of the method. We cannot calculate the square root of a negative number,
so we handle the case where
f
is negative before we do any calculations.
An example of a method that does not have a return value is the
HandleInput
method. Let us have a look at its header:


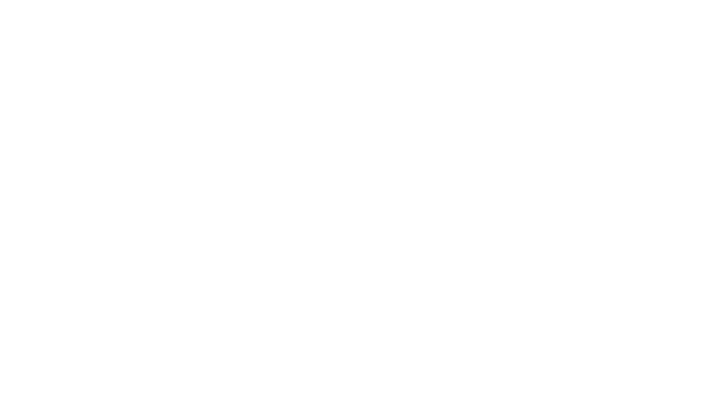