Java Reference
In-Depth Information
additional parameter for the maximum number of uses; the int value,
numMaxUses, is passed to the constructor and assigned to both the class
variables, maxUses and remainingUses (lines 36 and 37). Lines 41 through 46
include a third constructor with parameters for automatic expiration; the boolean
parameter pswdAutoExpires is assigned to the instance variable autoExpires. Lines
48 through 55 include a fourth constructor that has parameters for the maximum
number of uses and for automatic expiration.
Any instance variables not assigned new values retain their initial, or default,
values assigned at declaration. Because each constructor receives a password
value, each calls the set() method to set the current password to the new value.
The set() method, to be coded later, will verify that the new password value uses
the proper format and has not been used recently, and then it will call the appro-
priate method to encrypt the new value. Because the set() method, as well as
other methods it calls, may throw an exception to indicate a problem with the
new password, the constructors must claim the exception, as they will not handle
them. Because no specific type of exception yet exists for an invalid password,
each constructor simply claims a general Exception by using the keyword,
throws, followed by the word, Exception, in the method header (lines 27, 33, 41,
and 48 of Figure 9-9).
25
26
// Constructors for objects of class Password
27
public
Password
(
String
newPassword
)
throws
Exception
28
{
29
pswdHistory =
new
ArrayList
(
maxHistory
)
;
30
set
(
newPassword
)
;
31
}
32
33
public
Password
(
String
newPassword,
int
numMaxUses
)
throws
Exception
34
{
35
pswdHistory =
new
ArrayList
(
maxHistory
)
;
36
maxUses = numMaxUses;
37
remainingUses = numMaxUses;
38
set
(
newPassword
)
;
39
}
40
41
public
Password
(
String
newPassword,
boolean
pswdAutoExpires
)
throws
Exception
42
{
43
pswdHistory =
new
ArrayList
(
maxHistory
)
;
44
autoExpires = pswdAutoExpires;
45
set
(
newPassword
)
;
46
}
47
48
public
Password
(
String
newPassword,
int
numMaxUses,
boolean
pswdAutoExpires
)
throws
Exception
49
{
50
pswdHistory =
new
ArrayList
(
maxHistory
)
;
51
maxUses = numMaxUses;
52
remainingUses = numMaxUses;
53
autoExpires = pswdAutoExpires;
54
set
(
newPassword
)
;
55
}
56
FIGURE 9-9



















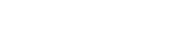







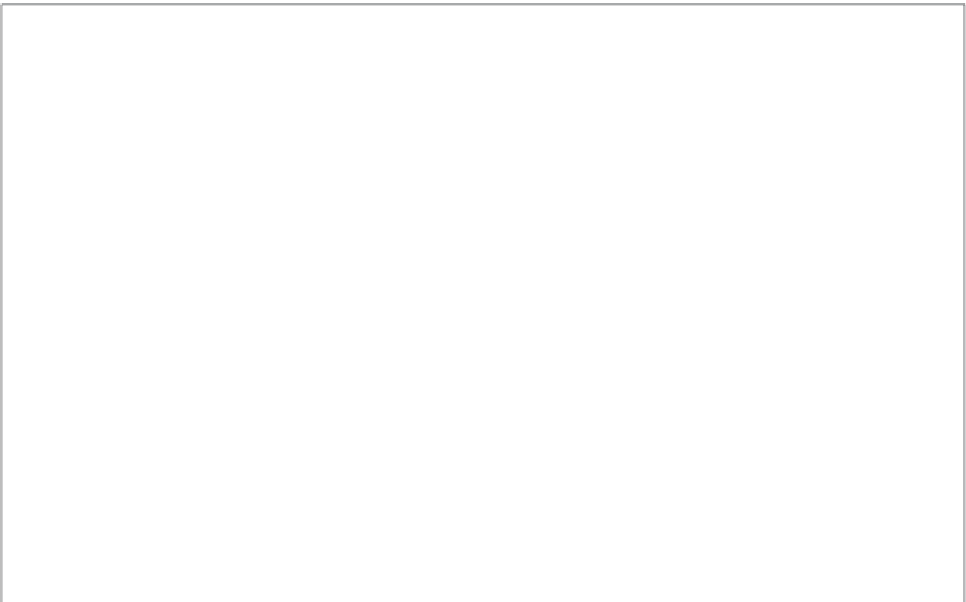
Search WWH ::

Custom Search