Java Reference
In-Depth Information
Using Try and Catch
Use the try and catch structure to ensure that all errors are
handled by the program as required by Java.
Although the BillPayer program does not use a random access file, you should
be aware that the RandomAccessFile class also contains methods to read, write,
close, and seek. The
seek() method
allows programmers to select a beginning
position within a file before they read or write data. The syntax is as follows:
myFile.seek(147);
When executed, this line of code selects, or points to, the 148th byte in the file,
because — as with array index numbers — numbering starts with zero. A sub-
sequent read() or write() method would then begin at that specific location.
Because Java considers data in bytes, the programmer needs to know how many
bytes are in a record. Though Java can read and write to the same random access
file, sequential files must be either input or output.
The checkFields() method
The checkFields() method is a user-defined method to look for blank or empty
fields in the interface. Figure 8-27 displays the code for the checkFields() method.
195
196
public boolean
checkFields
()
197
{
198
if
((
acctNum.getText
()
.compareTo
(
""
)
<1
)
||
199
(
pmt.getText
()
.compareTo
(
""
)
<1
)
||
200
(
firstName.getText
()
.compareTo
(
""
)
<1
)
||
201
(
lastName.getText
()
.compareTo
(
""
)
<1
)
||
202
(
address.getText
()
.compareTo
(
""
)
<1
)
||
203
(
city.getText
()
.compareTo
(
""
)
<1
)
||
204
(
state.getText
()
.compareTo
(
""
)
<1
)
||
205
(
zip.getText
()
.compareTo
(
""
)
<1
))
206
{
207
JOptionPane.showMessageDialog
(
null
,
"You must complete all fields."
,
"Data Entry Error"
,JOptionPane.WARNING_MESSAGE
)
;
208
return false
;
209
}
210
else
211
{
212
return true
;
213
}
214
}
FIGURE 8-27
Lines 198 through 205 employ a method named compareTo(), which is used
to compare the content of strings in Java. Recall that the compareTo() method
compares two String objects: the String object that calls the method and the String
object used as the method's parenthetical argument. The compareTo() method
returns a negative integer if the calling String object alphabetically precedes the
argument string and returns a value of zero when the two are equal. A positive
integer is returned if the calling String object alphabetically follows the argument
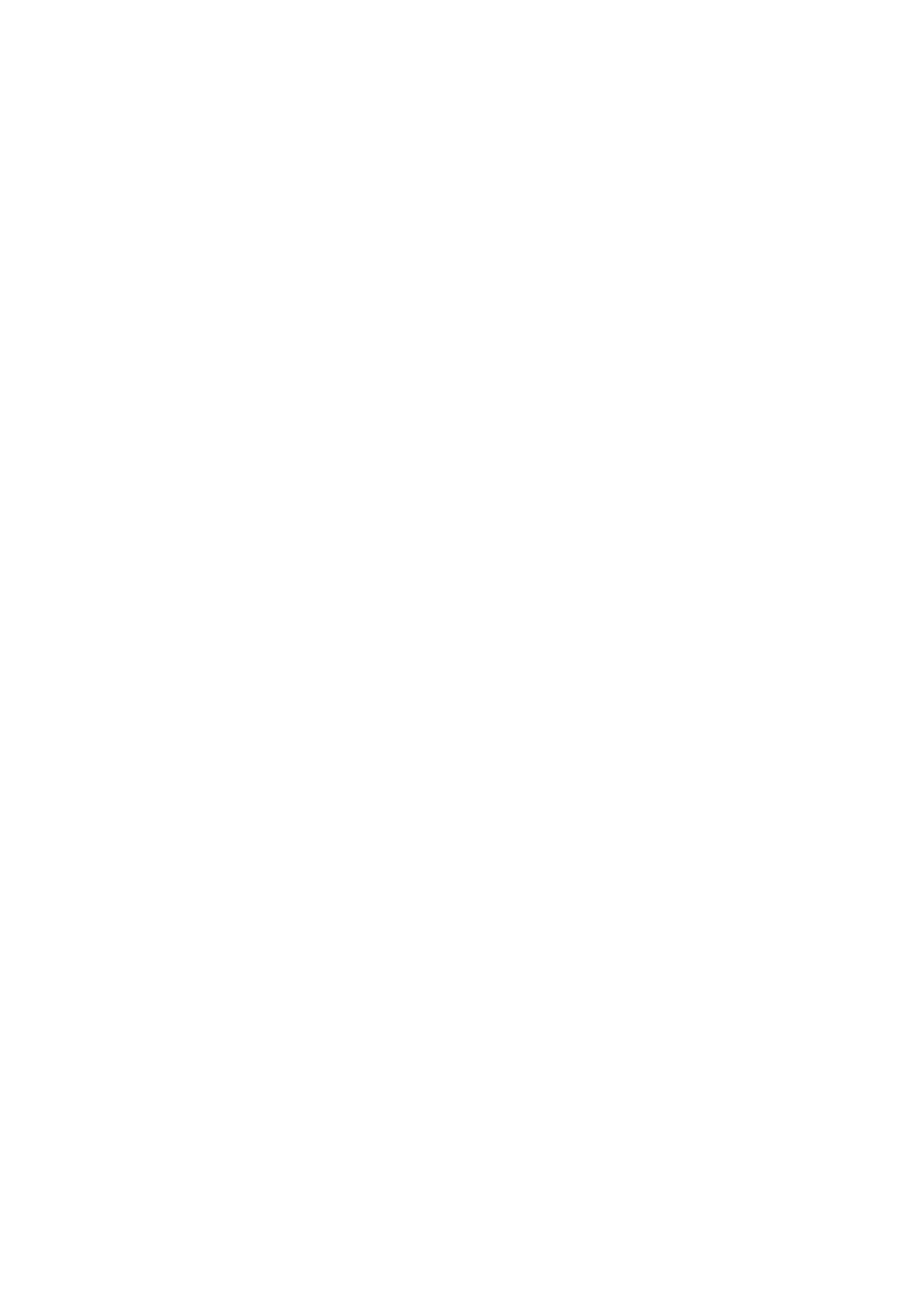

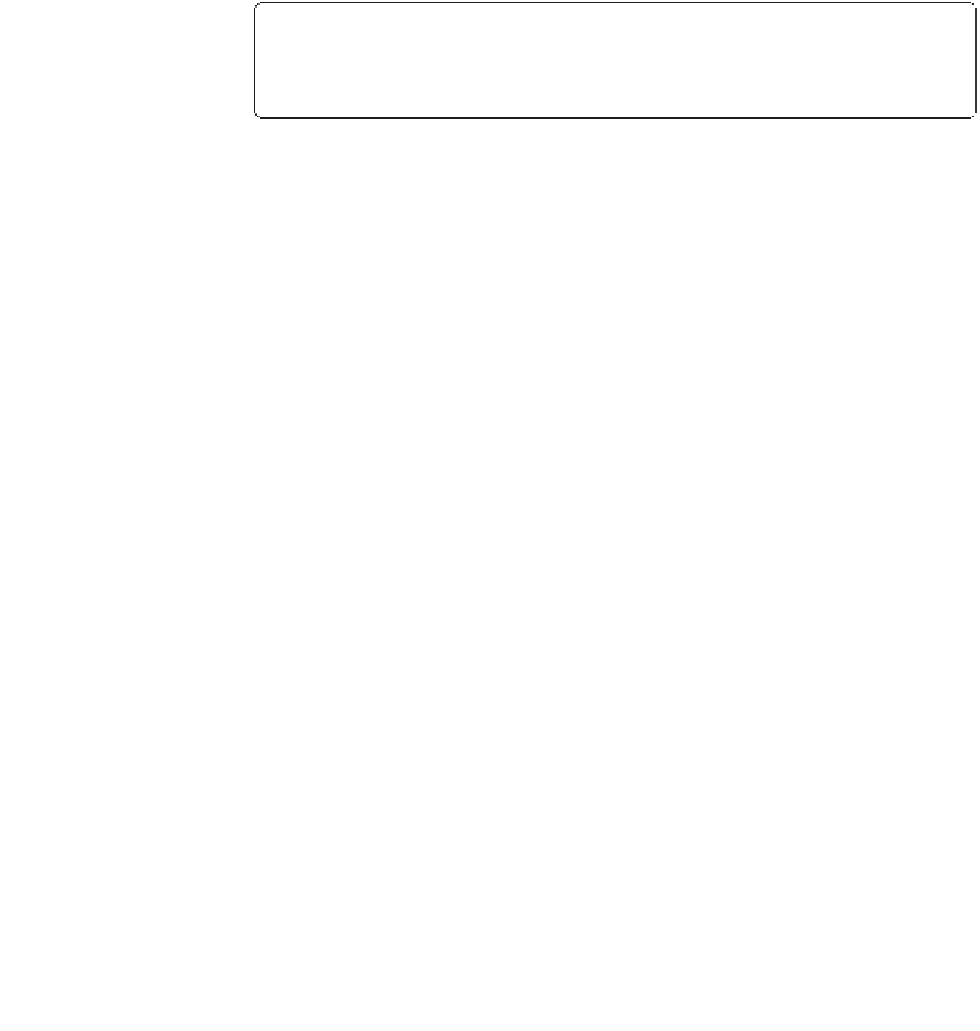


























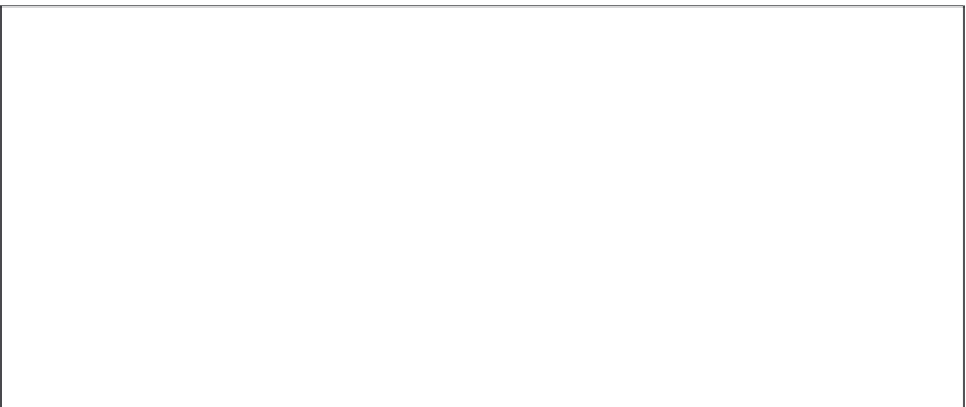
Search WWH ::

Custom Search