Java Reference
In-Depth Information
Adding Components to the Interface
Adding components to the BillPayer interface involves the following:
(1) constructing the components, (2) implementing layout managers for place-
ment, and (3) using the add() methods to insert the components into the JFrame.
The components in the BillPayer interface include the JFrame, which already was
created; the content pane to hold all of the components; JLabels to hold words,
instructions, or prompts; JTextFields to allow for user data entry; JButtons to add
functionality to the interface; and JPanels to assist with component placement.
You may remember that JPanels and JFrames are special containers that house
other components, such as user-interface controls and other containers.
Constructing the Components
Figure 8-12 displays the constructors for the BillPayer interface and the
declaration of the variable to hold the DataOutputStream. Eight JPanel contain-
ers are created in lines 21 through 28. Recall from the storyboard in Figure 8-3
on page 490 that each row of JLabels and JTextFields will be placed in its own
panel. Then, in line 31, a larger panel, named fieldPanel, will contain all of the
rows. Finally, line 32 constructs the buttonPanel.
15
public class
BillPayer
extends
JFrame
implements
ActionListener
16
{
17
//Declare output stream
18
DataOutputStream
output;
19
20
//Construct a panel for each row
21
JPanel firstRow =
new
JPanel
()
;
22
JPanel secondRow =
new
JPanel
()
;
23
JPanel thirdRow =
new
JPanel
()
;
24
JPanel fourthRow =
new
JPanel
()
;
25
JPanel fifthRow =
new
JPanel
()
;
26
JPanel sixthRow =
new
JPanel
()
;
27
JPanel seventhRow =
new
JPanel
()
;
28
JPanel eighthRow =
new
JPanel
()
;
29
30
//Construct a panel for the fields and buttons
31
JPanel fieldPanel =
new
JPanel
()
;
32
JPanel buttonPanel =
new
JPanel
()
;
33
34
//Construct labels and text boxes
35
JLabel acctNumLabel =
new
JLabel
(
"Account Number:
"
)
;
36
JTextField acctNum =
new
JTextField
(
15
)
;
37
JLabel pmtLabel =
new
JLabel
(
"Payment Amount:"
)
;
38
JTextField pmt =
new
JTextField
(
10
)
;
39
JLabel firstNameLabel =
new
JLabel
(
"First Name:
"
)
;
40
JTextField firstName =
new
JTextField
(
10
)
;
41
JLabel lastNameLabel =
new
JLabel
(
"Last Name:"
)
;
42
JTextField lastName =
new
JTextField
(
20
)
;
43
JLabel addressLabel =
new
JLabel
(
"Address:"
)
;
44
JTextField address =
new
JTextField
(
35
)
;
45
JLabel cityLabel =
new
JLabel
(
"City:
"
)
;
46
JTextField city =
new
JTextField
(
10
)
;
47
JLabel stateLabel =
new
JLabel
(
"State:"
)
;
48
JTextField state =
new
JTextField
(
2
)
;
49
JLabel zipLabel =
new
JLabel
(
"Zip:"
)
;
50
JTextField zip =
new
JTextField
(
9
)
;
51
52
//Construct button
53
JButton submitButton =
new
JButton
(
"Submit"
)
;
54
55
public static void
main
(
String
[]
args
)
FIGURE 8-12
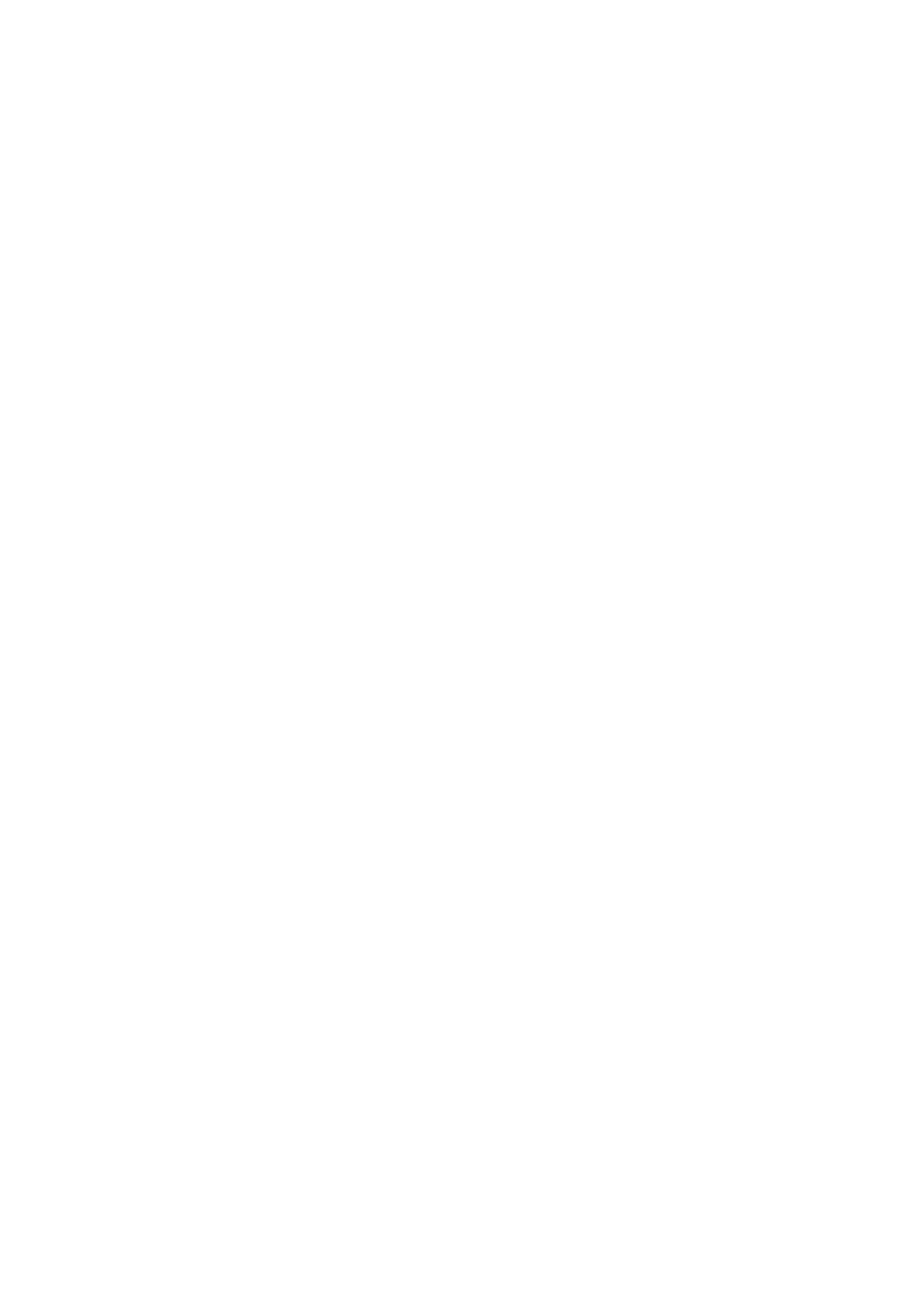



































Search WWH ::

Custom Search