Java Reference
In-Depth Information
In Java, an
assignment operator
, or
shortcut operator
, is used to perform
arithmetic and an assignment operation all with one operator, thus providing a
shortened form of that variable accumulation. One of the assignment operators,
the
add and assign operator
(
+=
), performs both addition of a new value and
assignment to a storage location, replacing the repetitive portion of the above
code with the following code:
variable += newValue;
For example, if you declare a variable with the identifier name counter and want
to add five to it each time the line of code is executed, the code would use the
add and assign operator (+=), as follows:
counter += 5;
Each time the above code is executed, Java adds five to the previous value of
counter and writes the new value back into the same storage location, effectively
overwriting the earlier value.
An easy way to increment a running count or counter is to use a special
operator called a unary operator. A
unary operator
is an operator that needs
only one value, or operand, to perform its function. Java supports the use of two
unary operators: the
increment operator
(
++
), which adds one to the operand,
and the
decrement operator
(
- -
), which subtracts one from the operand.
With traditional operators, such as an addition operator, you must supply at
least two values to be added. With unary operators, you use a special symbol that
performs a mathematical operation on a single value. For example, if you want
to increment the variable, i, by one each time the code executes, you could write
the following code using an addition operator:
i = i + 1;
Using an assignment operator, the code becomes a little shorter.
i+=1;
Using a unary operator, the code becomes even simpler.
i++;
An interesting feature of unary operators is that they behave differently
depending on whether the unary operator is positioned before or after the vari-
able. If the increment operator comes after the variable, as in the above example,
the value is incremented after the line of code is executed. If, however, the incre-
ment operator precedes the variable, the value is incremented before the line of
code is executed. In a single line of code, the placement of the unary operator
does not make any difference. If, however, the unary operator is included as part
of a formula or in an assignment statement, the placement is crucial. For exam-
ple, the following code:
i = 5;
answer = ++i;
results in the value of answer being equal to six, because the variable, i, is
incremented before the line of code is executed. Any later references to the
value, i, will be evaluated as six, as well.
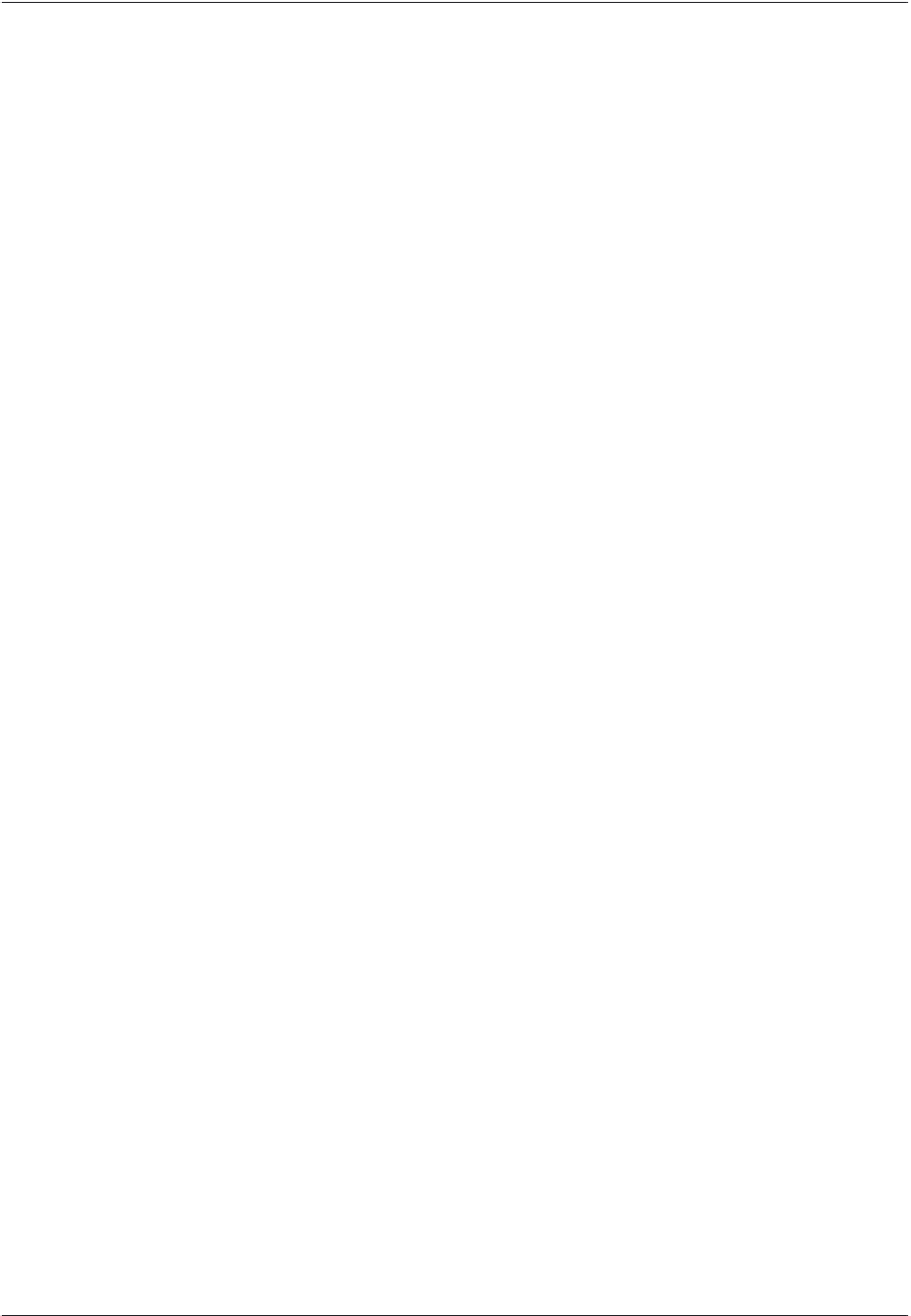
Search WWH ::

Custom Search