Java Reference
In-Depth Information
expression that evaluates to true or false. The condition repeats as long as the
condition is evaluated as true. The condition eventually must evaluate to false in
order to exit the loop, based on some condition or user input that changed.
Java provides another type of loop to use if you know exactly how many
times you want the program to repeat a set of instructions. A loop that executes
a specific number of times is called a
counter-controlled loop
, or
measured
loop
.
The for Statement
Java uses a
for statement
to implement a counter-controlled loop, also
called a
for loop
. The for statement lists the parameters of a for loop, which
include the beginning value, the stop condition that tests whether to continue to
loop, and the increment to use for the counter. The three parameters
are enclosed in parentheses and separated by semicolons. The for statement is
followed by a line of code or a block of statements, enclosed in braces, which
execute the number of times defined in the for statement.
Table 5-7 displays the general form of the for statement.
Table 5-7
The for Statement
General form:
for (start; stop; counter-control)
{
. . . lines of code to repeat;
}
Comment:
The word,
for
, is a reserved keyword. The start parameter typically is a variable initialized to
a beginning value and is done only once per invocation of the loop. The stop parameter is a
condition that tests whether to continue the loop. As long as the condition evaluates to
true, the loop continues. The
counter-control
, sometimes called the update, typically is an
expression indicating how to increment or decrement the counter. Semicolons separate the
three parameters enclosed in parentheses.
Examples:
1.
for (int j=1; j<5; j++)
//j is initialized to 1; the loop continues while j is less than 5; j is incremented by 1 at each
pass of the loop; the loop will execute 4 times.
2.
for (int counter=6; counter>0; counter--)
//counter is initialized to 6; the loop continues while counter is greater than 0; counter is
decremented by 1 at each pass of the loop; the loop will execute 6 times.
3.
for (int evenValues=2; evenValues<=100; evenValues+=2)
//evenValues is initialized to 2; the loop continues while evenValues is less than or equal to
100; evenValues is incremented by 2 at each pass of the loop; the loop will execute 50
times.
The first parameter in the for statement is an assignment statement to tell the
compiler to store an integer, positive or negative, as the start value. Any valid inte-
ger can be used as the start value for the for loop. That value defines where the
compiler will start counting as it executes the for loop. For example, if you wanted
to manipulate each element of an array in a for loop, you would set your first
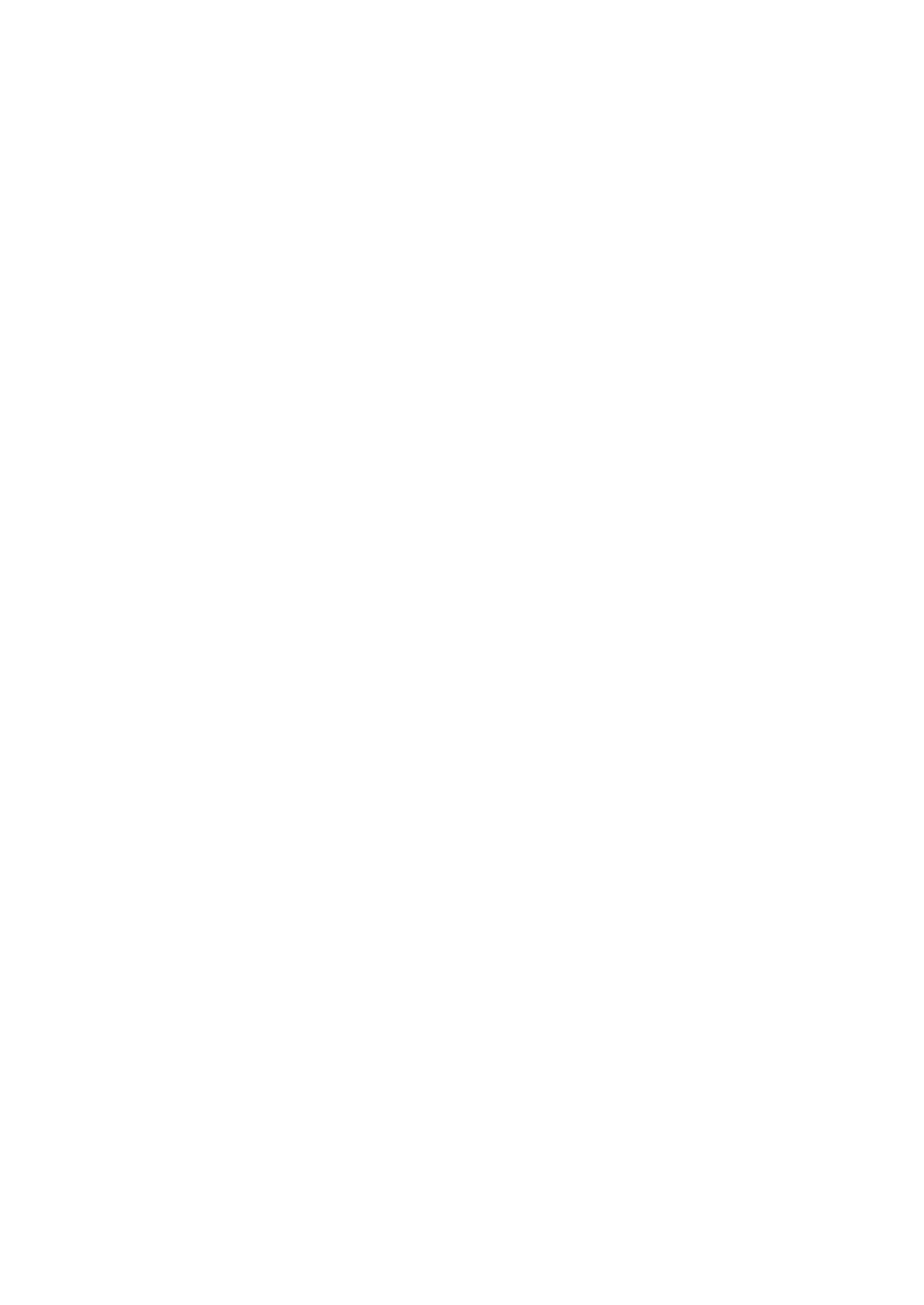
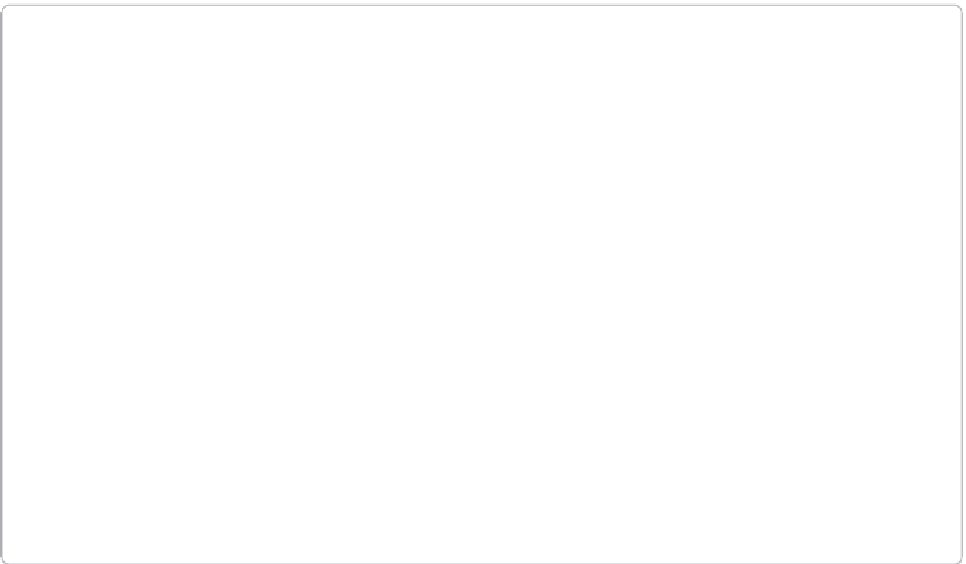



Search WWH ::

Custom Search