Java Reference
In-Depth Information
When a run-time exception occurs, the run-time system then looks for a
handler, or a way to handle the exception
.
A
checked exception
is one in which
the compiler checks each method during compilation to ensure that each
method has a
handler
— the code used to address any possible exceptions.
A method can handle a checked exception in one of two ways: (1) by han-
dling the exception using a catch statement, or (2) by throwing the exception to
the code that called the method. By using the keyword, throws, in the method
header, the method
claims
the exception; in other words, it lets the compiler
know that it may pass along an exception rather than handling it.
Using a checked exception thus allows programmers to catch an exception
and handle it in the program. Often programmers write code to catch the excep-
tion at the exact point in the program where it might occur, or in other cases,
they write code within a calling method, thereby handling it at a higher level.
If the programmer does not code a way to catch the exception, the program
may terminate prematurely and display an exception error message. In some
cases, Java may throw an exception representing an unrecoverable situation, such
as an out of memory error, which is a more serious problem. The object created
in response to such an exception can be caught; however, it is not required
because it indicates a serious problem that an application should not try to catch.
Handling Exceptions Using try and catch Statements
Java provides several ways to write code that checks for exceptions. One
object-oriented way to handle exceptions is to include the lines of code that
might cause exceptions inside a try statement. The
try statement
identifies a
block of statements that potentially may throw an exception. If an exception
occurs, the try statement transfers execution to a handler. Table 4-6 shows
an example of the try statement.
Table 4-6
The try Statement
General form:
try
{
. . . lines of code that might generate an exception;
. . .throw new exceptionName;
}
Purpose:
To enclose the code statements that might throw an exception.
Try
and
throw new
are
reserved words. All statements within the braces in the try statement are monitored for
exceptions. Programmers may
explicitly
, or purposefully, cause an exception by typing the
words, throw new, followed by the name of a standard Java exception object. A try
statement must be followed by a catch statement.
Example:
try
{
answer = 23 / 0; //Java throws exception automatically
throw new DivideByZeroException(); //programmer explicitly
throws exception
}
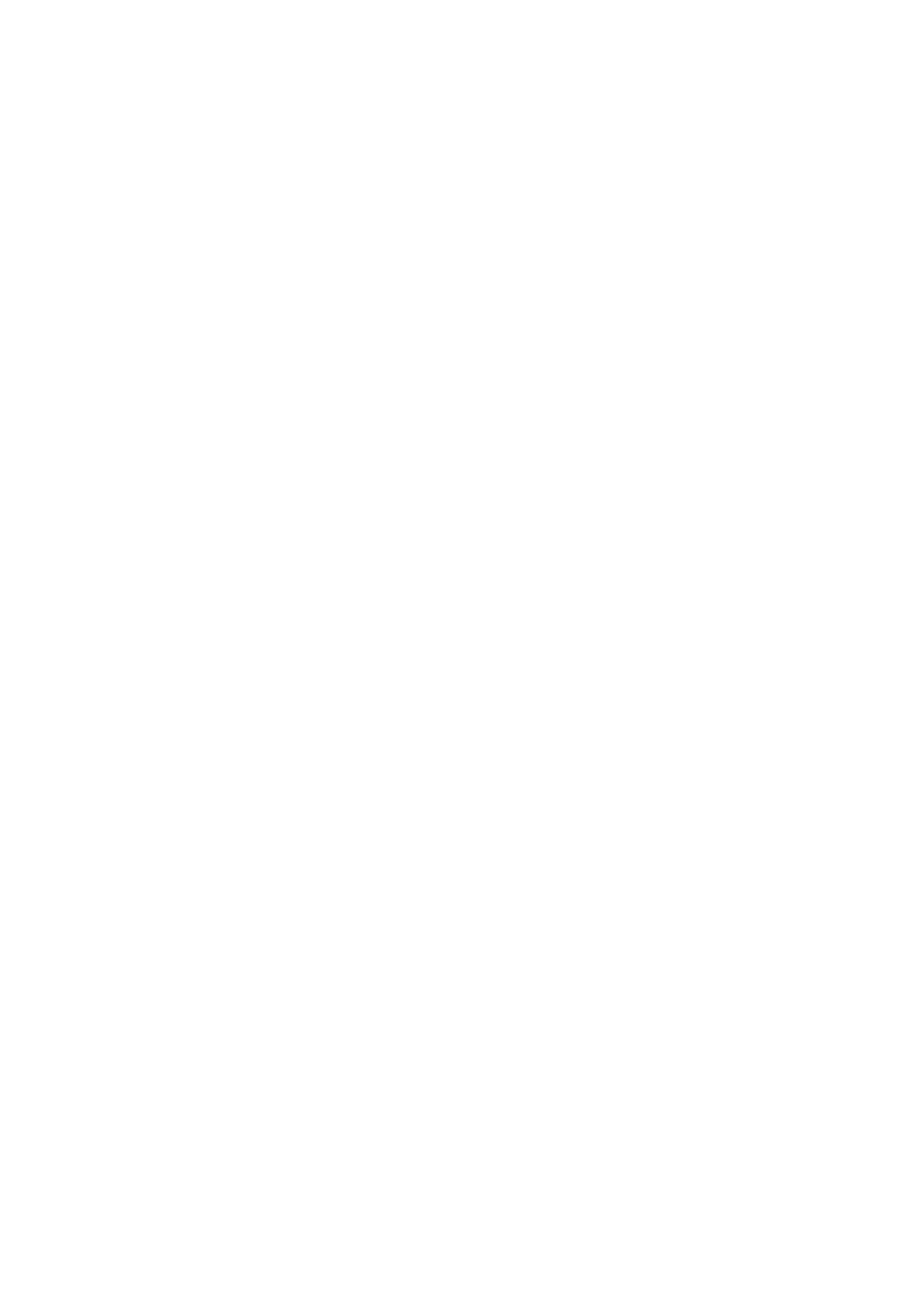
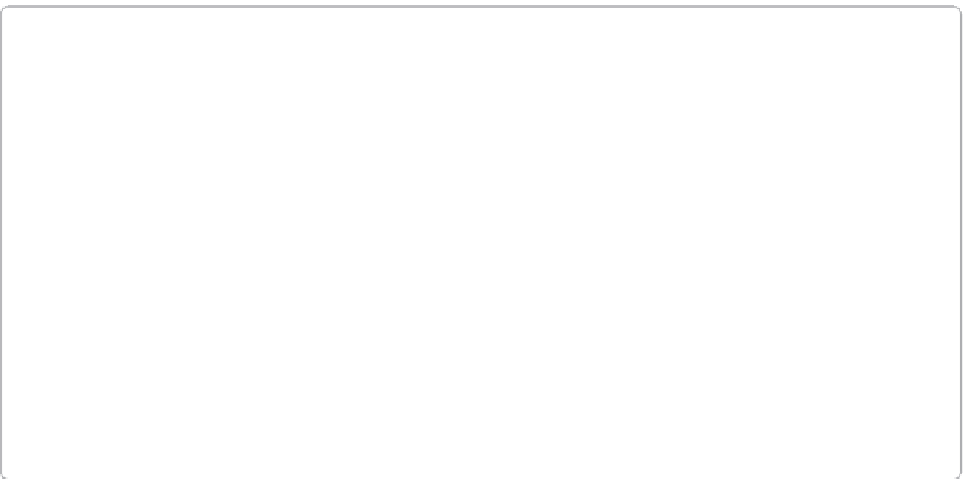



Search WWH ::

Custom Search