Graphics Programs Reference
In-Depth Information
The locations of the lasers are stored so that after firing, they can be reset to their orig-
inal locations. We are also attaching a laser sound that will be played when the lasers
are fired. Remember to go to the Sound Properties from the Library and make sure that
the linkage has been established for all sounds used.
After the game initialization, we come to the code for firing lasers at a target. When the
mouse is clicked, if the
shoot
variable is false meaning there are no lasers in motion,
the laser sound is played and
shoot
is set to true. When the lasers are fired, they go
from their starting positions to the target location in 8 steps. This is done by taking the
difference between the target location and their initial positions and dividing by 8.
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
// fire lasers at an asteroid target
target_mc.onRelease = function()
{
if (shoot == false)
{
// shoot the laser
shootLaser.start();
shoot = true;
// record the click location
xloc = target_mc._x;
yloc = target_mc._y;
// define the incremental laser moves
// (distance to asteroid)/8
dxL = (xloc - laserLx)/8;
// left laser
dyL = (yloc - laserLy)/8;
dxR = (xloc - laserRx)/8;
//right laser
dyR = (yloc - laserRy)/8;
}
}
The asteroids are created and displayed using the
placeObj()
and
displayObj()
functions previously discussed.
The only new wrinkle is the recording of the asteroid color using the Color object in
line 76. This is used to darken the tint of faraway asteroids rather than applying alpha
transparency. A tint color value is determined based on the z-value of the asteroid,
and the
setTransform
method carries out the color change in lines 123 and 124.
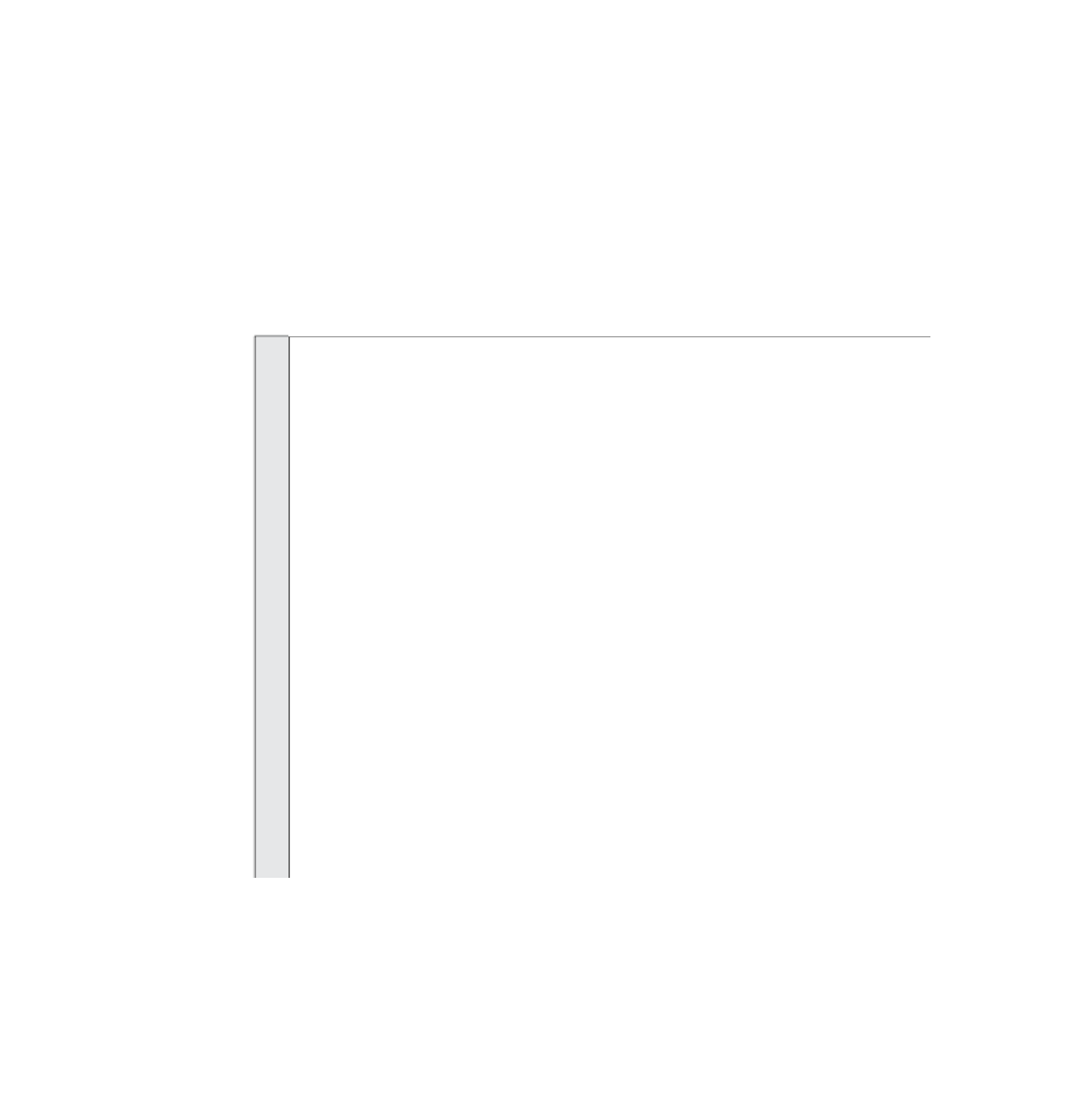
Search WWH ::

Custom Search