Graphics Programs Reference
In-Depth Information
We will have to include some additional viewer information to effect the angular rota-
tion. Let's define a viewer rotation angle that is initially set to 2 degrees in line 6, but
that could be any number we choose. This represents the incremental change in the
viewer angle
ry
during rotation about the y-axis.
1
2
3
4
5
6
7
8
9
//create a viewer object with position & rotation properties
viewer = {x:0, y:0, z:0, ry:0 }
// set the viewer parameters
var d:Number = 600;
// distance from viewer to picture plane
var viewerAngle:Number = 2;
// the angular change of viewer
var viewerSpeed:Number = 10;
// the viewer speed
var tooClose:Number = 100;
// the too close distance
Step 2: Calculate the rotational angle trig functions
There is one other piece of information we will need regarding the angle of rotation of
the viewer. As the rotation angle is increased or decreased, we will have to update the
sine and cosine of that angle. Let's write a function that will do this for us and, at the
same time, convert from degrees to radians. We've actually seen these expressions
many times already. Insert these lines before the creation of the picture plane.
10
11
12
13
14
15
16
17
// function to find the sin and cos
// of the y-angle of rotation (ry)
function calcSinCosY()
{
viewer.cy = Math.cos(viewer.ry * Math.PI/180);
viewer.sy = Math.sin(viewer.ry * Math.PI/180);
}
Step 3: Create the picture plane
Our current exercise has no
scene3D
picture plane defined, so let's add a line of code to
create one through an empty movie clip. We'll keep the same coordinates for the place-
ment of the movie clip.
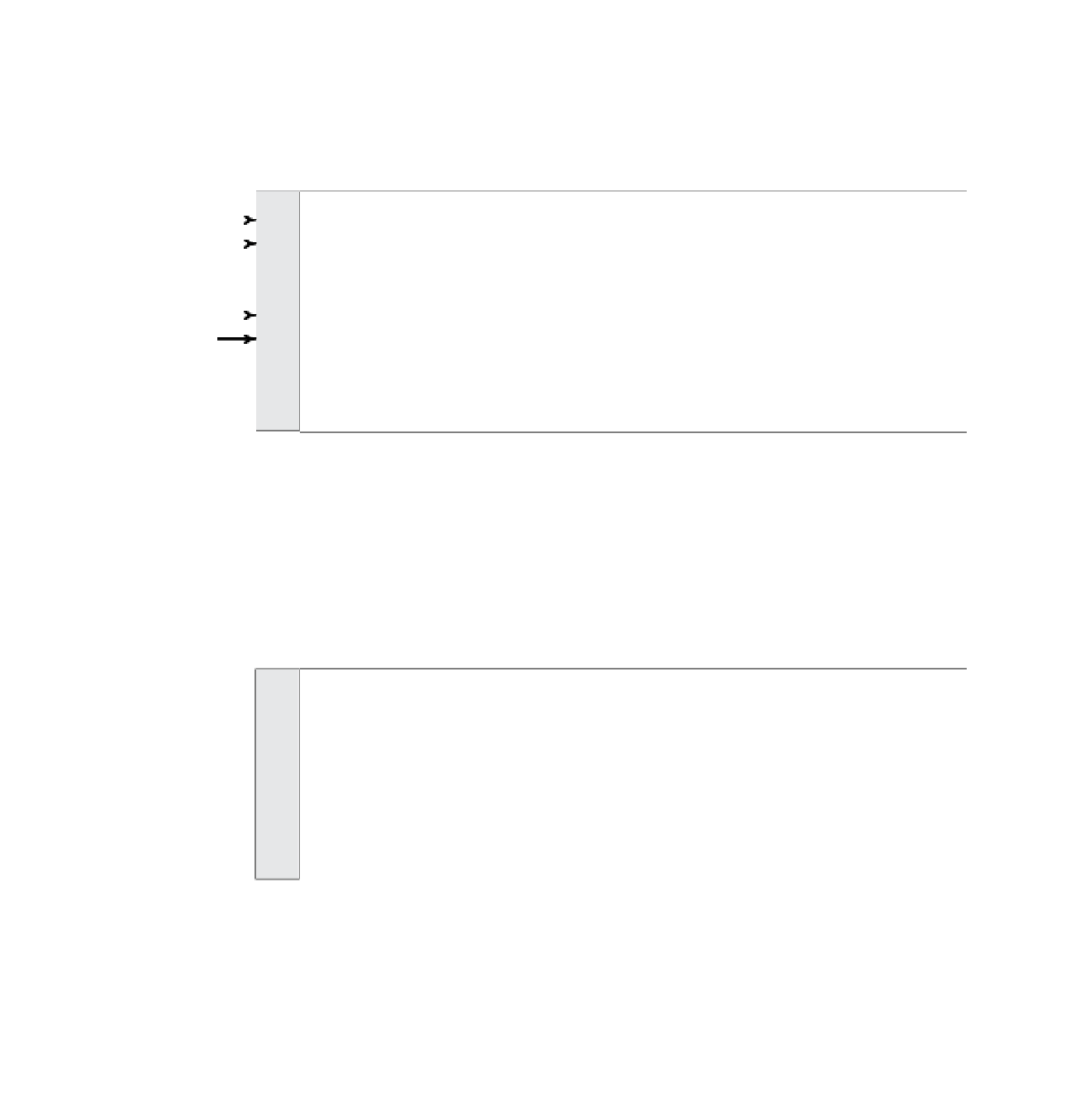



Search WWH ::

Custom Search