Graphics Programs Reference
In-Depth Information
1
2
3
4
// initialize
Mouse.hide();
// hide the normal cursor
flicked = false;
// the tongue has not yet been flicked
Step 2: Move the bug
Let's define a function,
moveBug()
, that will enable the bug to move. The first thing we
want to do is to set the bug's position to the coordinates of the cursor. This is straight-
forward, but the results aren't satisfactory. The bug needs to be a little more uneven in
its movement. To accomplish this, we will add
Math.random()*10
to both the horizontal
and vertical position of the bug in lines 8 and 9. So although the bug will globally follow
the cursor, there will be random variations between 0 and 10 in its location.
5
6
7
8
9
10
function moveBug()
{
// set the bug location to the cursor position
bug_mc._x = _xmouse + Math.random()*10;
bug_mc._y = _ymouse + Math.random()*10;
There is one other thing we will add to make the movement a little more realistic. When
we move the cursor to the right, we expect the bug to face to the right. When we move
the cursor to the left, we expect the bug to face to the left. This can easily be done by
setting the bug's
_xscale
to either 100 (pointing right) or -100 (pointing left).
To do this, we have to look at the current horizontal location of the cursor. If the cur-
rent x-axis value is less than the previous x-axis value of the cursor, then the cursor
has moved to the left. In this case we will set the scale factor to -1 (line 13). If the
current location is greater than the previous x-axis value of the cursor, then the cursor
has moved to the right, and we will set the scale factor to 1 (line 15). If there has been
no change in the cursor, we'll set the scale factor to be the same as the value it was
last set to (line 17).
11
12
13
14
15
16
17
// set the direction of the bug according to
// the horizontal movement of the cursor
if (_xmouse < oldx ) {scaleFactor = -1;}
else
if (_xmouse > oldx ) {scaleFactor = 1;}
else
{scaleFactor = lastScale;}
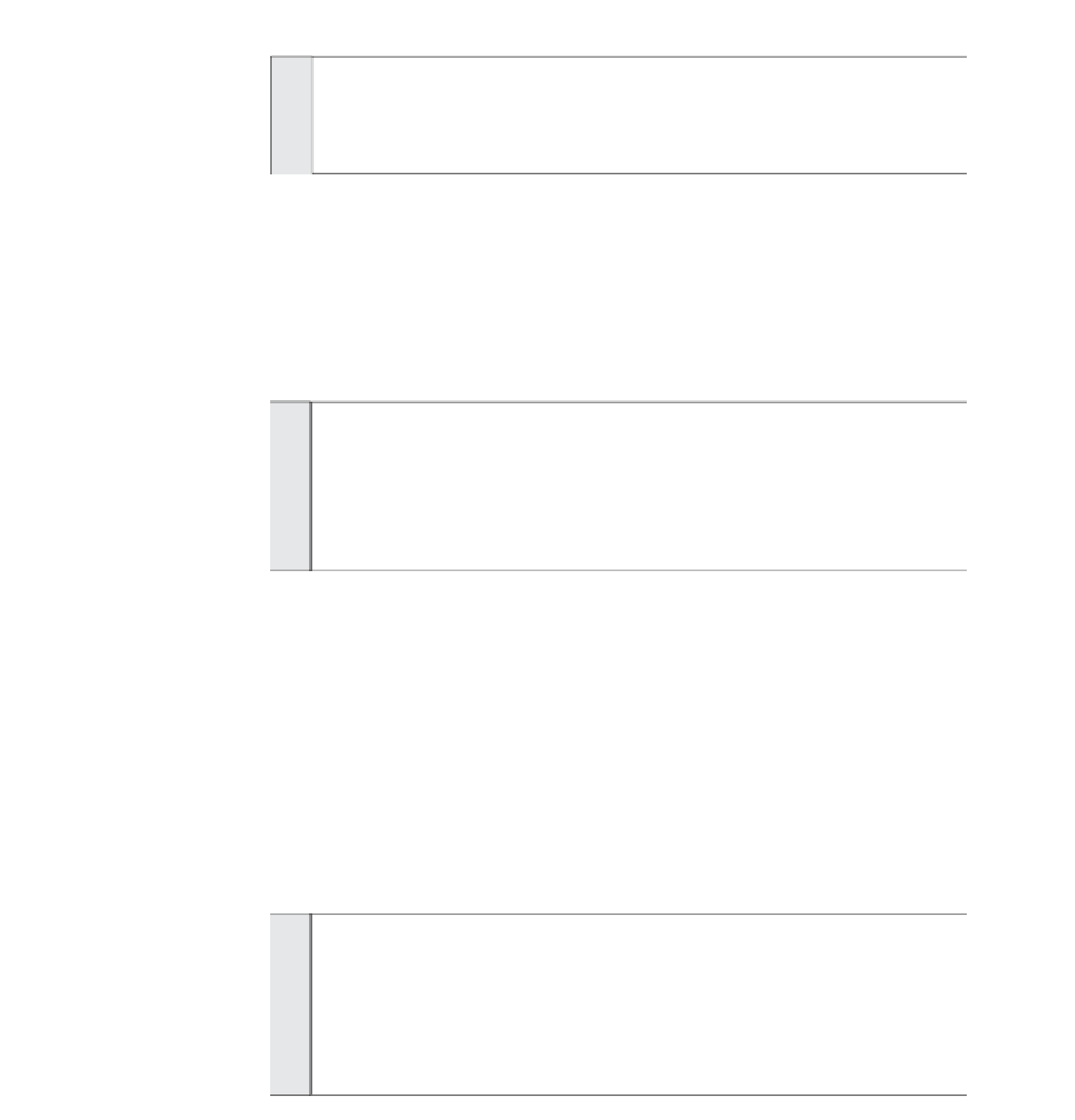
Search WWH ::

Custom Search