Java Reference
In-Depth Information
figure 2.9
Printing a two-
dimensional array
1
public class MatrixDemo
2
{
3
public static void printMatrix( int [ ][ ] m )
4
{
5
for( int i = 0; i < m.length; i++ )
6
{
7
if( m[ i ] == null )
8
System.out.println( "(null)" );
9
else
10
{
11
for( int j = 0; j < m[i].length; j++ )
12
System.out.print( m[ i ][ j ] + " " );
13
System.out.println( );
14
}
15
}
16
}
17
18
public static void main( String [ ] args )
19
{
20
int [ ][ ] a = { { 1, 2 }, { 3, 4 }, { 5, 6 } };
21
int [ ][ ] b = { { 1, 2 }, null, { 5, 6 } };
22
int [ ][ ] c = { { 1, 2 }, { 3, 4, 5 }, { 6 } };
23
24
System.out.println( "a: " ); printMatrix( a );
25
System.out.println( "b: " ); printMatrix( b );
26
System.out.println( "c: " ); printMatrix( c );
27
}
28
}
2.4.6
enhanced
for
loop
Java 5 adds new syntax that allows you to access each element in an array or
ArrayList
, without the need for array indexes. Its syntax is
for(
type var
:
collection
)
statement
Inside
statement
,
var
represents the current element in the iteration. For
instance, to print out the elements in
arr
, which has type
String[]
, we can
write:
for( String val : arr )
System.out.println( val );
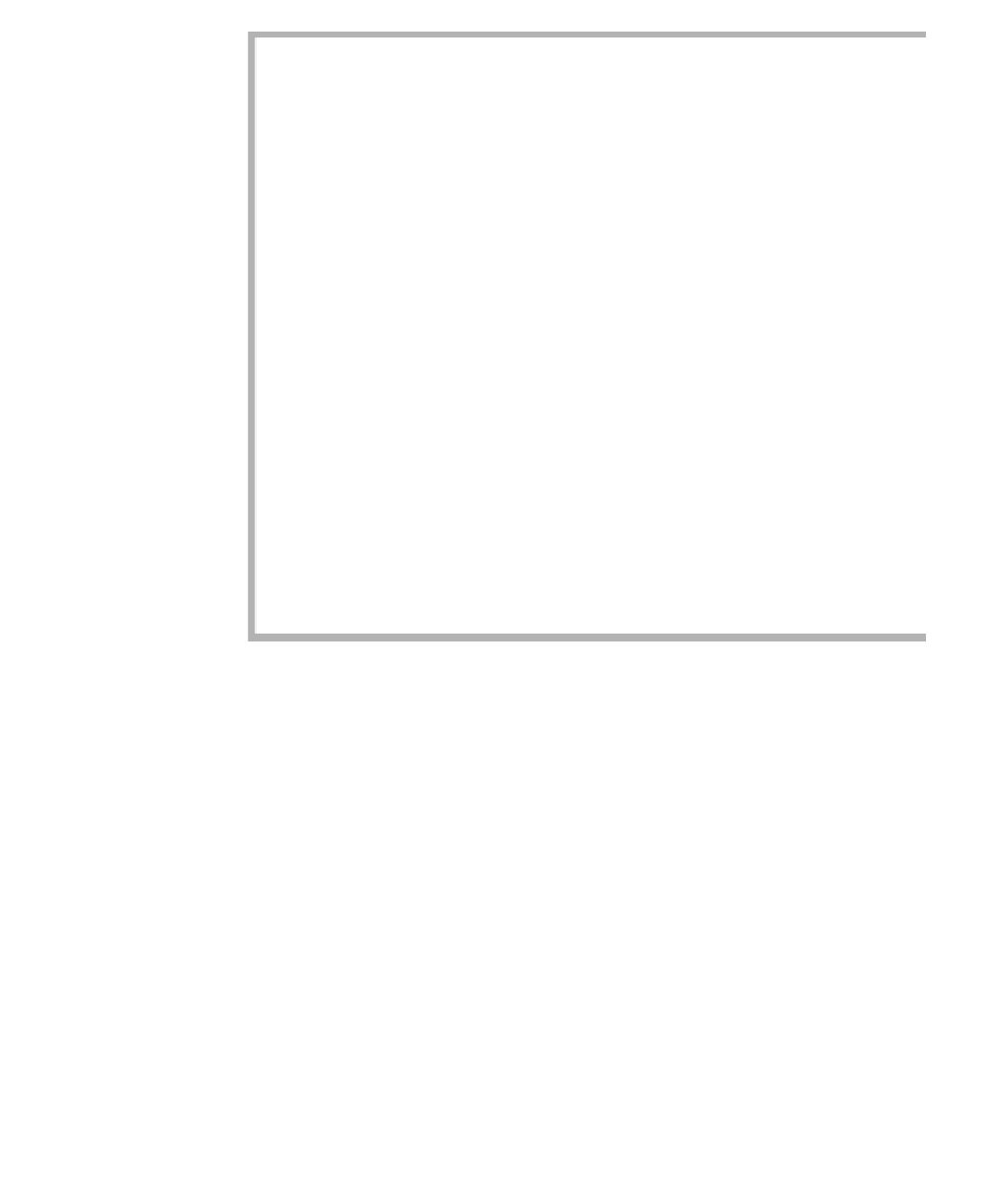
Search WWH ::

Custom Search